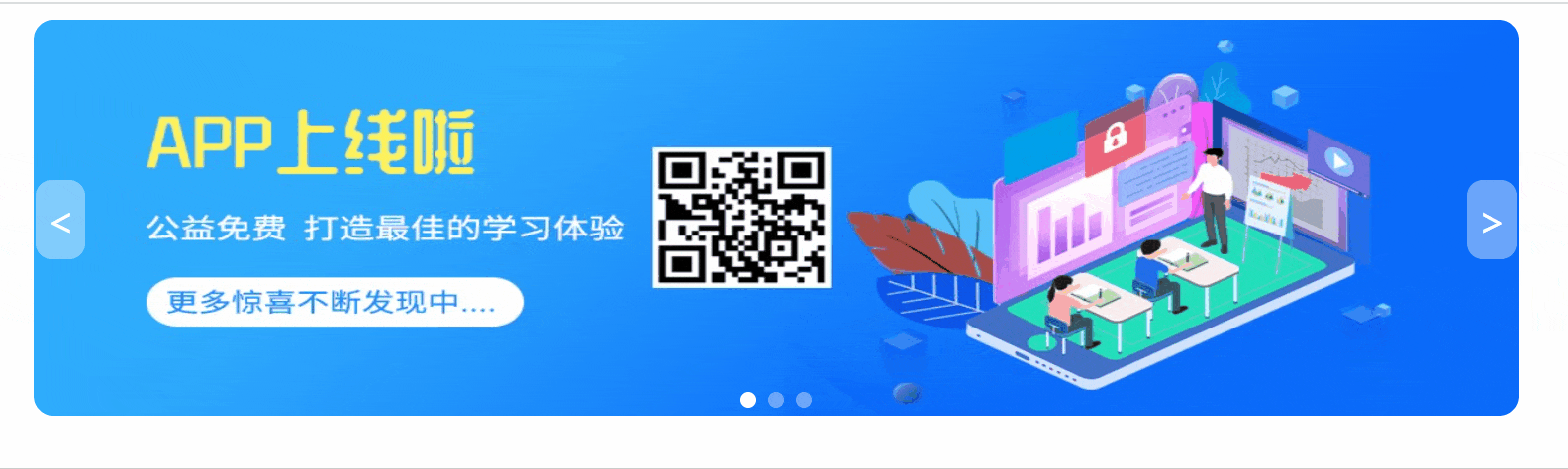
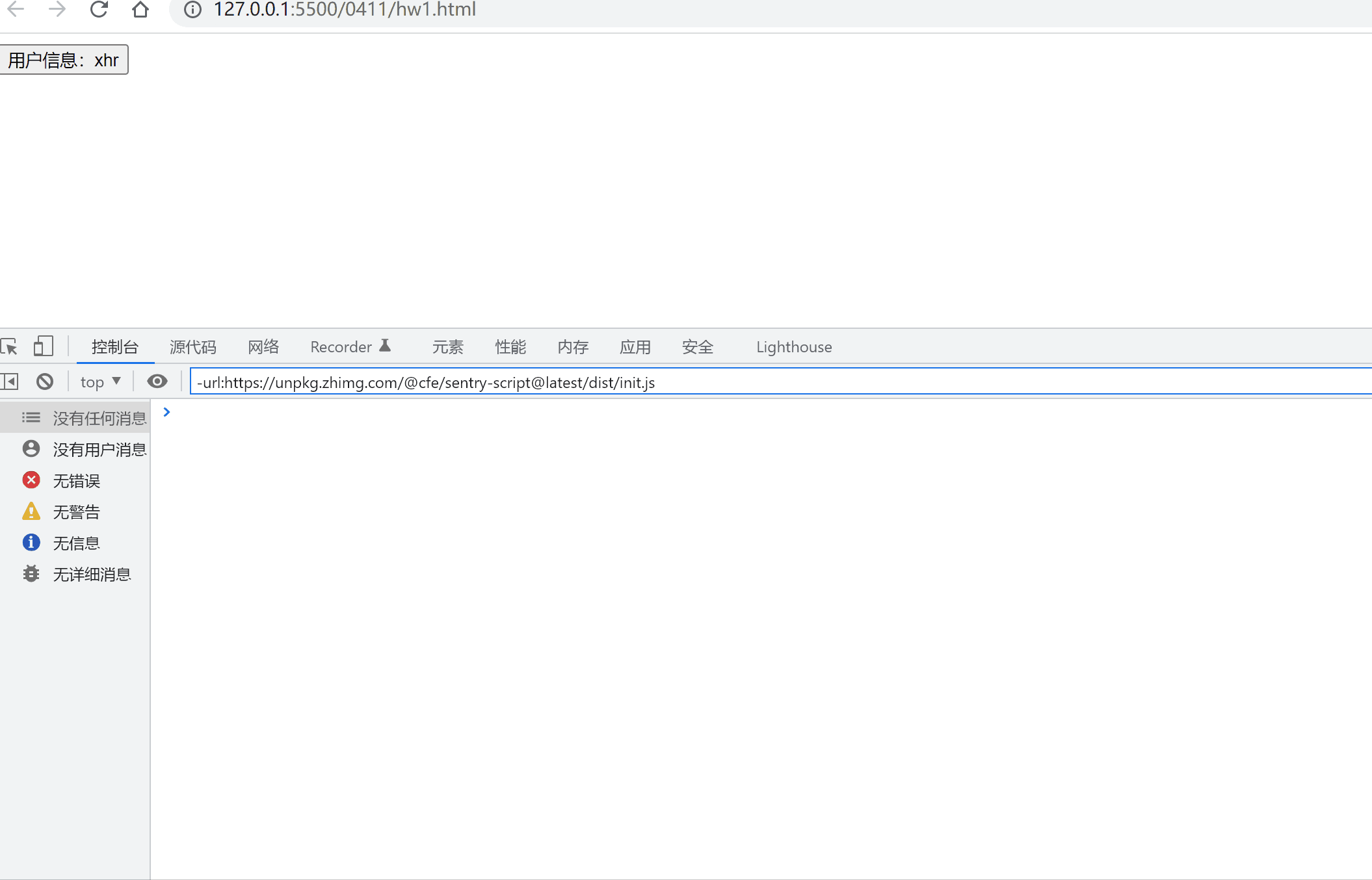
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>轮播图</title>
<style>
/* ! 3. 轮播图 */
.slider {
max-width: 750px;
min-width: 320px;
margin: auto;
padding: 0 10px;
transition: width 0.5s;
}
.slider .imgs {
/* 图片容器必须要有高度,否则下面图片不能正常显示 */
height: 200px;
}
.slider .imgs img {
/* 图片完全充满父级空间显示 */
height: 100%;
width: 100%;
/* 图片带有圆角 */
border-radius: 10px;
/* 默认图片全部隐藏,只有有active的图片才显示 */
display: none;
}
.slider .imgs img:hover {
cursor: pointer;
}
/* 默认显示第一张 */
.slider .imgs img.active {
display: block;
}
/* 轮播图按钮组 */
.slider .btns {
/* 按钮水平一排显示,用flex,且水平居中 */
display: flex;
place-content: center;
}
.slider .btns span {
/* 按钮宽高相同,确定显示成一个正圆 */
width: 8px;
height: 8px;
/* 加上红色背景和数字是为了布局时可以看到,一会更去掉 */
background-color: rgba(255, 255, 255, 0.4);
/* 50%可确保显示为正圆 */
border-radius: 50%;
/* 按钮上外边距负值,可将它上移,可移动到图片中下方 */
margin: -12px 3px 5px;
}
.slider .btns span.active {
background-color: #fff;
}
.slider .side {
box-sizing: border-box;
display: flex;
place-content: space-between;
font-size: 20px;
color: #fff;
}
.slider .side .leftside {
display: flex;
align-items: center;
place-content: center;
width: 25px;
height: 40px;
border-radius: 8px;
background-color: rgba(255, 255, 255, 0.4);
margin: -120px 1px 0px;
}
.slider .side .leftside:hover {
cursor: pointer;
background-color: red;
}
.slider .side .rightside {
display: flex;
align-items: center;
place-content: center;
width: 25px;
height: 40px;
border-radius: 8px;
background-color: rgba(255, 255, 255, 0.4);
margin: -120px 1px 0px;
}
.slider .side .rightside:hover {
cursor: pointer;
background-color: red;
}
</style>
</head>
<body>
<div class="slider">
<!--
图片容器
1. 图片组
2. 按钮组
注: 按钮数组与图片数量是一样的
-->
<div class="imgs" onmouseleave="autoPlay()" onmouseover="pause()">
<!-- 轮播图默认从第一张开始显示 -->
<img src="./images/banner1.jpg" alt="" data-index="1" class="active" />
<img src="./images/banner2.jpg" alt="" data-index="2" />
<img src="./images/banner3.png" alt="" data-index="3" />
</div>
<!-- 切换按钮数量与图片数量必须一致 -->
<div class="btns" onmouseleave="autoPlay()" onmouseover="pause()">
<span data-index="1" class="active" onclick="setActive()"></span>
<span data-index="2" onclick="setActive()"></span>
<span data-index="3" onclick="setActive()"></span>
</div>
<!-- 左右切换按钮 -->
<div class="side" onmouseleave="autoPlay()" onmouseover="pause()">
<div class="leftside" onclick="sideclick()"><</div>
<div class="rightside" onclick="sideclick()">></div>
</div>
</div>
</body>
<script>
const imgList = document.querySelectorAll(".imgs img");
const btnList = document.querySelectorAll(".btns span");
let dataList = [...imgList].map((item) => item.dataset.index);
const tempIndex = dataList.shift();
dataList.push(tempIndex);
let playMain = null;
function cleanActive() {
// 清空active
[...imgList].forEach((item) => item.classList.remove("active"));
[...btnList].forEach((item) => item.classList.remove("active"));
}
function setActive() {
cleanActive();
// 切换active
[...imgList].filter((item) => {
if (item.dataset.index === event.currentTarget.dataset.index) {
item.classList.add("active");
}
});
[...btnList].filter((item) => {
if (item.dataset.index === event.currentTarget.dataset.index) {
item.classList.add("active");
}
});
}
function pause() {
clearInterval(playMain);
}
function autoPlayRender(tempIndex){
cleanActive();
[...imgList].filter((item) => {
if (item.dataset.index === tempIndex) {
item.classList.add("active");
}
});
[...btnList].filter((item) => {
if (item.dataset.index === tempIndex) {
item.classList.add("active");
}
});
}
function autoPlay() {
playMain = setInterval(() => {
const tempIndex = dataList.shift();
// console.log(typeof parseInt(tempIndex));
dataList.push(tempIndex);
autoPlayRender(tempIndex);
}, 3000);
}
function sideclick(){
if(event.currentTarget.className==="leftside"){
const tempIndex = dataList.pop();
dataList.unshift(tempIndex);
autoPlayRender(tempIndex);
}else{
const tempIndex = dataList.shift();
dataList.push(tempIndex);
autoPlayRender(tempIndex);
}
}
window.onload = autoPlay;
</script>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Ajax-XHR</title>
</head>
<body>
<button onclick="getUser(this)">用户信息:xhr</button>
</body>
<script>
function getUser() {
// 1.创建xhr对象
const xhr = new XMLHttpRequest();
const url = "http://xhr411.edu/users.php";
// 2.响应类型
xhr.responseType = "json";
// 3.配置参数
xhr.open("GET", url, true);
// 4.请求成功的回调
xhr.onload = () => {
console.log(xhr.response);
};
// 5.请求失败的回调
xhr.onerror = () => {
console.log("请求错误");
};
// 6.返回的值
xhr.send(null);
}
</script>
</html>