


Best practices for object-relational mapping in PHP object-relational mapping and database abstraction layers
PHP Object Relational Mapping (ORM) best practices include naming consistency, proper mapping, annotations, avoiding hardcoding, leveraging query builders, and monitoring database schema changes. In practical cases, the Doctrine ORM framework can be used to connect to the MySQL database and query data. You need to configure the database connection and use the query builder to generate efficient queries.
PHP object-relational mapping and database abstraction layer: object-relational mapping best practices
Between relational databases and PHP objects The transformation is called object-relational mapping (ORM). ORM frameworks simplify this process and provide additional features such as query construction and object relationship management.
Best Practices
- Object naming consistency: ORM automatically generated object names should match database column names.
- Build appropriate mappings: Ensure that the ORM model and database structure closely match to avoid data inconsistencies.
- Use mapping annotations: Use annotations in ORM model classes to specify column mappings, primary keys, and relationships.
- Avoid Hardcoding: Avoid hardcoding database connections or queries in ORM code.
- Utilize query builders: ORM frameworks usually provide query builders for generating efficient, readable queries.
- Monitor database schema changes: Use tools or mechanisms to monitor database schema changes and update the ORM model accordingly.
Practical Case: Using Doctrine ORM
Doctrine ORM is a popular PHP ORM framework. Here is an example that demonstrates how to use Doctrine ORM to connect to a MySQL database and query data.
1. Install Doctrine ORM:
composer require doctrine/orm
2. Configure database connection:
use Doctrine\ORM\EntityManager; use Doctrine\ORM\Tools\Setup; use Doctrine\ORM\Configuration; // 创建一个 Entity Manager $isDevMode = true; $config = Setup::createAnnotationMetadataConfiguration([__DIR__ . '/src'], $isDevMode); $conn = array( 'driver' => 'pdo_mysql', 'user' => 'root', 'password' => 'password', 'dbname' => 'database_name', ); $em = EntityManager::create($conn, $config);
3. Query Data:
$qb = $em->createQueryBuilder(); $qb->select('p.id', 'p.name') ->from('Product', 'p'); $query = $qb->getQuery(); $results = $query->getResult(); foreach ($results as $result) { echo $result['name'] . PHP_EOL; }
The above is the detailed content of Best practices for object-relational mapping in PHP object-relational mapping and database abstraction layers. For more information, please follow other related articles on the PHP Chinese website!
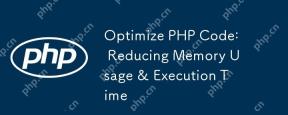
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
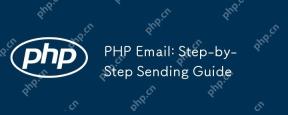
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
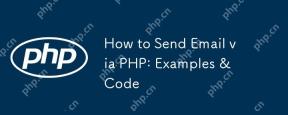
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
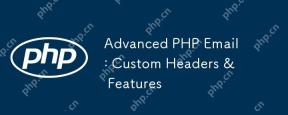
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
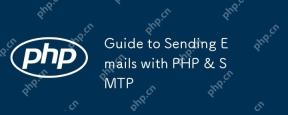
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
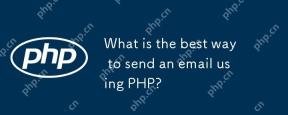
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
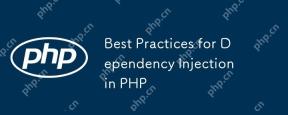
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
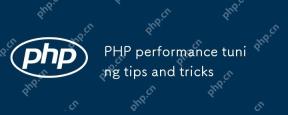
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
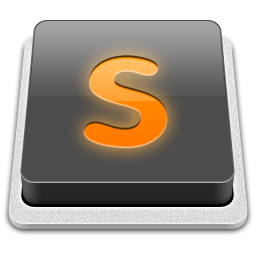
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
