Best data structure choice for PHP array specific element lookup
The best data structure choice for finding specific elements in PHP depends on the search requirements: Array: Suitable for small arrays or infrequent searches. Ordered array: Allows binary search, suitable for sorted arrays that require efficient search. SplFixedArray: Optimizes arrays, improves speed and memory utilization, and has similar search efficiency to arrays. Hash table: Stores data in key-value pairs, allowing extremely fast lookups by key, but takes up more memory.
The best data structure choice for PHP array specific element lookup
In PHP, dealing with arrays is common and essential. In order to find specific elements in an array quickly and efficiently, it is crucial to choose an appropriate data structure. This article will explore the best data structure options for different search requirements and provide practical examples.
Search methods and their complexity
Before choosing a data structure, it is important to understand the different search methods and their complexity:
- Linear search: Check each element in the array one by one until the target element is found. The complexity is O(n), where n is the size of the array.
- Binary search: Split the array into two halves, compare the target element and the middle element, and eliminate half of the possibilities. The complexity is O(log n).
- Hash table: Stores elements in key-value pairs, allowing fast lookup of elements by key. The complexity is O(1), as long as the hash function is efficient.
Data structure options
1. Array
Array is the default data structure in PHP. Although it can perform linear search, the complexity is high. However, arrays can be a simple and effective choice if they are relatively small and lookups are performed infrequently.
Practical case:
$array = ['apple', 'banana', 'cherry']; $key = 'cherry'; if (in_array($key, $array)) { // 目标元素存在于数组中 } else { // 目标元素不存在于数组中 }
2. Ordered array
An ordered array is an array arranged in a specific order (ascending or descending order). It allows efficient binary searches.
Practical case:
$array = ['apple', 'banana', 'cherry', 'dog', 'fish']; sort($array); // 将数组按升序排列 $key = 'apple'; $low = 0; $high = count($array) - 1; while ($low <= $high) { $mid = floor(($low + $high) / 2); $guess = $array[$mid]; if ($guess == $key) { // 目标元素存在于数组中 break; } elseif ($guess < $key) { $low = $mid + 1; } else { $high = $mid - 1; } } if ($guess == $key) { // 目标元素存在于数组中 } else { // 目标元素不存在于数组中 }
3. SplFixedArray
SplFixedArray is an optimized array in the PHP standard library, designed to provide fast index access accelerate. It has similar lookup efficiency as arrays but provides better performance and memory utilization.
Practical case:
$array = new SplFixedArray(100); $array[42] = 'foo'; $key = 42; if ($array->offsetExists($key)) { // 目标元素存在于数组中 } else { // 目标元素不存在于数组中 }
4. Hash table
Hash table stores data in the form of key-value pairs. It allows fast lookup by key with O(1) complexity. However, it takes up more memory than an array, and can be a waste for arrays where lookups are not often needed.
Practical case:
$map = new SplObjectStorage(); $map['apple'] = 'red'; $map['banana'] = 'yellow'; $key = 'apple'; if ($map->offsetExists($key)) { // 目标元素存在于哈希表中 } else { // 目标元素不存在于哈希表中 }
The above is the detailed content of Best data structure choice for PHP array specific element lookup. For more information, please follow other related articles on the PHP Chinese website!
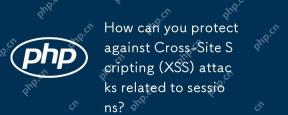
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
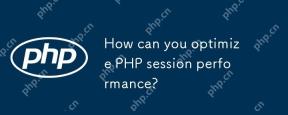
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
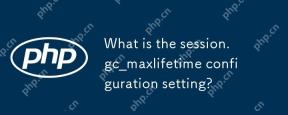
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
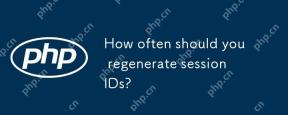
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
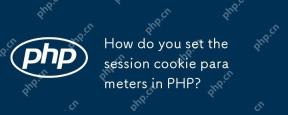
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
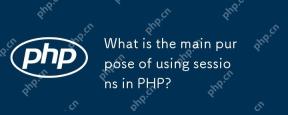
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
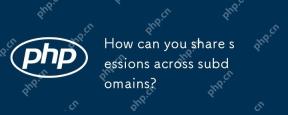
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),