How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
introduction
Have you ever wondered how to share a conversation between different subdomains? In modern web development, the seamlessness of user experience is crucial, and conversation sharing is the key to achieving this. Today, we will explore in-depth how to share conversations between subdomains, revealing technical details and practical experiences.
This article not only tells you how to do it, but also provides you with the reasons why you do it, as well as the problems and solutions you may encounter in your practical application. Read this article and you will learn the techniques of sharing sessions between subdomains and learn some optimizations and best practices from it.
Review of basic knowledge
Before we go deeper, let’s review the relevant basics. Session is an important concept in web development, which is used to maintain status information when users browse websites. Typically, session data is stored on a server and the user is identified by a unique session ID.
Subdomain is part of the domain name system, used to organize and separate different parts of the website. For example, blog.example.com
and shop.example.com
are different subdomains under the same domain example.com
.
Core concept: Implementation of session sharing
Definition and function of session sharing
The core idea of session sharing is to share the same session data between different subdomains. This means that the user's session state on blog.example.com
can be seamlessly passed to shop.example.com
, providing a consistent user experience.
The main method of implementing session sharing is to store session cookies by setting a common domain name. For example, if your domain name is example.com
, you can set the domain of the session cookie to .example.com
so that all subdomains can access this cookie.
How it works
To implement session sharing, you first need to configure the session management system on the server side. This usually involves the following steps:
Set the domain of session cookie : When setting the session cookie on the server side, set its domain to
.example.com
so that all subdomains can access this cookie.Session storage : Session data can be stored in memory, in a database, or using a distributed cache system (such as Redis). Which storage method to choose depends on your application requirements and architecture.
Session ID delivery : When a user accesses different subdomain names, the session ID is passed through cookies, and the server retrieves and updates the session data based on this ID.
Here is a simple example showing how to set up session sharing in PHP:
// Set session sharing session_set_cookie_params(0, '/', '.example.com'); session_start();
This code snippet sets the domain of the session cookie to .example.com
, thus enabling session sharing.
Example of usage
Basic usage
In practical applications, the basic steps for implementing session sharing are as follows:
// Basic usage of session sharing in PHP session_set_cookie_params(0, '/', '.example.com'); session_start(); // Set session data $_SESSION['user_id'] = 123; // Access session data between different subdomains echo $_SESSION['user_id']; // Output: 123
This example shows how to set up session sharing in PHP and access session data between different subdomains.
Advanced Usage
For more complex application scenarios, you may need to consider the following points:
Distributed Session Storage : Use Redis or Memcached to store session data to support high concurrency and scale-out.
Session persistence : Persist the session data into the database so that it can still be accessed after the server restarts.
Security : Use HTTPS to encrypt session cookies to prevent session hijacking.
Here is an example of using Redis to store session data:
// Use Redis to store session data $redis = new Redis(); $redis->connect('localhost', 6379); session_set_save_handler( function($session_id) use ($redis) { return $redis->get($session_id); }, function($session_id, $data) use ($redis) { $redis->set($session_id, $data); }, // Other callback functions... ); session_set_cookie_params(0, '/', '.example.com'); session_start(); $_SESSION['user_id'] = 123;
This example shows how to use Redis to store session data to enable distributed session management.
Common Errors and Debugging Tips
When implementing session sharing, you may encounter the following common problems:
Session cookie setting error : If the domain setting of the session cookie is incorrect, it may cause the session data to be unable to be shared among subdomains. Make sure the domain of the session cookie is set to
.example.com
.Session data loss : If there is a problem with the session storage system, session data may be lost. Back up session data regularly and use a distributed storage system to improve reliability.
Security Risk : Session hijacking is a common security risk in web applications. Use HTTPS to encrypt session cookies and rotate session IDs regularly for increased security.
Performance optimization and best practices
Here are some recommendations for performance optimization and best practices when implementing session sharing:
Using distributed caching : Using Redis or Memcached to store session data can significantly improve the performance and scalability of session management.
Session data minimization : Only the necessary session data is stored to reduce the overhead of storage and transmission.
Session Expiration Policy : Set a reasonable session expiration time to prevent session data from pile up.
Code readability and maintenance : Keep code readability and maintenance when implementing session sharing. Use comments and documents to explain the logic of session management.
Here is an optimized session management example:
// Optimized session management example session_set_cookie_params(0, '/', '.example.com', true, true); // Using HTTPS and HttpOnly session_start(); // Only the necessary session data is stored $_SESSION['user_id'] = 123; // Set the session expiration time ini_set('session.gc_maxlifetime', 3600); // 1 hour
This example shows how to optimize session management by setting security properties of session cookies, storing only necessary data, and setting session expiration time.
In-depth thinking and suggestions
When implementing session sharing, the following points need to be considered:
Pros and cons analysis : Session sharing can provide a consistent user experience, but also adds complexity and security risks. You need to weigh the pros and cons and decide whether to use session sharing based on specific needs.
Points : Common pitfalls include session cookie setting errors, session data loss and security risks. When implementing session sharing, special attention should be paid to these issues and corresponding measures should be taken to avoid them.
Future Development : As technology develops, conversation management may have new solutions and best practices. Stay tuned for industry trends and timely update and optimize conversation management strategies.
Through this article, you should have mastered the techniques of sharing sessions between subdomains and understand some of these optimizations and best practices. I hope this knowledge can help you better realize session sharing in real projects and provide a better user experience.
The above is the detailed content of How can you share sessions across subdomains?. For more information, please follow other related articles on the PHP Chinese website!
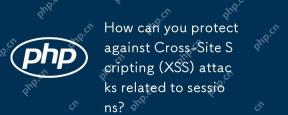
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
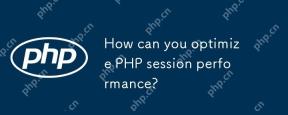
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
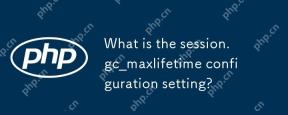
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
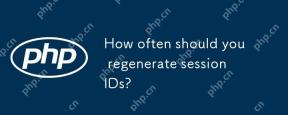
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
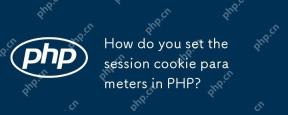
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
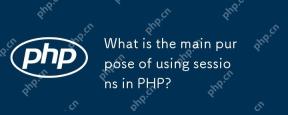
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
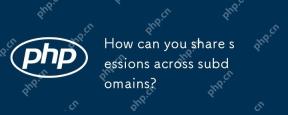
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
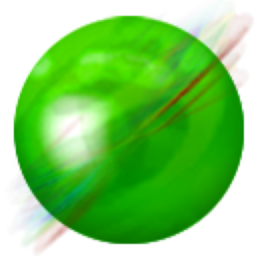
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
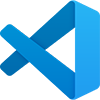
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version