Setting the session cookie parameter in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to requirements, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
Setting session cookie parameters in PHP is a key skill, especially when handling user sessions and security. Let's dive into how to achieve this and share some practical experiences.
introduction
Managing user sessions is a common and important task during development. PHP provides powerful session management functions, and setting session cookie parameters is one of the key links. Through this article, you will learn how to flexibly configure these parameters to improve the security and user experience of your application.
Review of basic knowledge
In PHP, sessions maintain user status through cookies. A cookie is a small file that stores data on the client, while a session cookie is used to keep the user's status while browsing a website. Understanding the basic concepts of cookies and PHP's session management mechanism is the basis for setting session cookie parameters.
Core concept or function analysis
Definition and function of session cookie parameters
Session cookie parameters include but are not limited to expiration time, path, domain name, security flag, etc. These parameters determine the life cycle and access scope of the cookie, which in turn affects the management and security of the session. For example, setting a short expiration time can improve security, while setting the correct path and domain name can control the access scope of cookies.
How it works
In PHP, setting the session cookie parameter can be implemented through the session_set_cookie_params()
function. This function allows you to define various properties of a cookie before the session begins. Here is a simple example:
// Set session cookie parameters session_set_cookie_params(3600, '/', 'example.com', true, true); session_start();
In this example, we set the expiration time of the session cookie to 3600 seconds (1 hour), the path to the root directory, the domain name is example.com
, and the security flag and the HttpOnly flag are enabled.
Example of usage
Basic usage
In actual applications, you may need to set session cookie parameters according to different needs. Here is a basic usage example:
// Set session cookie parameter session_set_cookie_params([ 'lifetime' => 1800, // 30 minutes 'path' => '/', 'domain' => 'example.com', 'secure' => true, 'httponly' => true, 'samesite' => 'Lax' ]); session_start();
In this example, we used array form to set parameters, which is supported in PHP 7.3 and above. Note the samesite
parameter, which can help prevent cross-site request forgery (CSRF) attacks.
Advanced Usage
In some cases, you may need to dynamically set the session cookie parameters. For example, adjust the life cycle of a cookie based on the user's login status:
if (user_is_logged_in()) { session_set_cookie_params([ 'lifetime' => 86400, // 24 hours 'path' => '/', 'domain' => 'example.com', 'secure' => true, 'httponly' => true, 'samesite' => 'Strict' ]); } else { session_set_cookie_params([ 'lifetime' => 1800, // 30 minutes 'path' => '/', 'domain' => 'example.com', 'secure' => true, 'httponly' => true, 'samesite' => 'Lax' ]); } session_start();
In this example, we set different session cookie parameters according to the user's login status. The logged-in user's session cookie has a longer life cycle and samesite
parameter is set to Strict
to provide higher security.
Common Errors and Debugging Tips
Common errors when setting session cookie parameters include:
- Forgot to call
session_start()
: If you do not callsession_start()
after setting parameters, these parameters will not take effect. - Improper parameter setting : For example, the wrong domain name or path is set, causing the cookies to not be stored or read correctly.
- Ignore security flags : Not setting
secure
andhttponly
flags may cause security vulnerabilities.
When debugging these issues, you can use var_dump($_SESSION)
and var_dump($_COOKIE)
to check the status of the session and cookies. In addition, checking the cookie information in the browser's developer tools can also help you find problems.
Performance optimization and best practices
There are several performance optimization and best practices to note when setting session cookie parameters:
- Set life cycle reasonably : Too long life cycles may increase the burden on the server, while too short life cycles may affect the user experience. Adjust according to actual needs.
- Use
samesite
parameter : Using thesamesite
parameter in supported browsers can improve security and reduce the risk of CSRF attacks. - Enable
secure
andhttponly
flags : These flags improve the security of cookies and prevent access to cookies through JavaScript. - Code readability and maintenance : Ensure the readability and maintenance of the code when setting session cookie parameters. For example, setting parameters using arrays can make the code clearer.
Through the above methods, you can flexibly set session cookie parameters to improve application security and performance. In actual development, it is very important to adjust according to specific needs and environment. Hope these experiences and suggestions can help you easily manage PHP sessions.
The above is the detailed content of How do you set the session cookie parameters in PHP?. For more information, please follow other related articles on the PHP Chinese website!
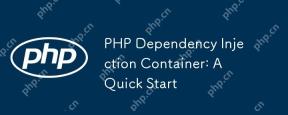
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
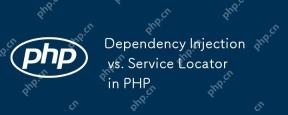
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
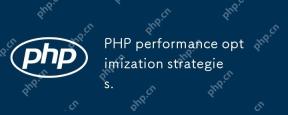
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
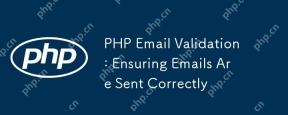
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
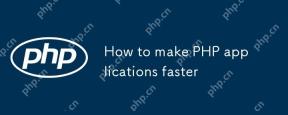
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
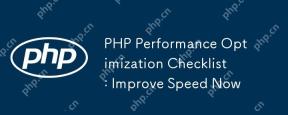
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
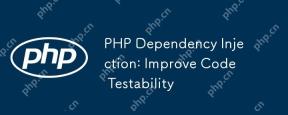
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
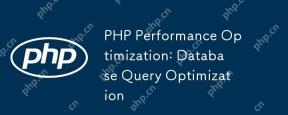
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
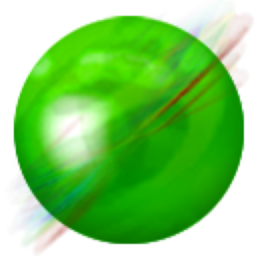
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
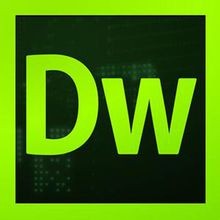
Dreamweaver CS6
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
