Select Dependency Injection (DI) for large applications, Service Locator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) Service Locator obtains services through central registration, which is convenient but may lead to an increase in code coupling.
When it comes to managing dependencies in PHP applications, two popular patterns often come into play: Dependency Injection (DI) and Service Locator. So, which one should you choose? Let's dive into this topic with a bit of flair and personal experience.
Dependency Injection, in my view, is like having a personal chef who prepares your meals exactly to your taste. You tell them what you need, and they deliver it right to your plate. On the other hand, the Service Locator pattern feels more like going to a buffet. You go to the counter, and you pick what you need from a variety of options. Both have their charm, but they also come with their own set of challenges and benefits.
Let's explore Dependency Injection first. It's all about injecting dependencies into your classes rather than letting them fetch their own. Here's a simple example using PHP:
class Logger { public function log($message) { echo $message . "\n"; } } class UserService { private $logger; public function __construct(Logger $logger) { $this->logger = $logger; } public function getUser($id) { $this->logger->log("Fetching user with id: $id"); // Fetch user logic here } } $logger = new Logger(); $userService = new UserService($logger); $userService->getUser(1);
In this example, UserService
depends on Logger
, and we inject it through the constructor. This approach promotes loose coupling and makes your code more testable. You can easily swap out the Logger
with a mock in your unit tests. However, setting up all these dependencies can sometimes feel like a puzzle, especially in larger applications.
Now, let's look at the Service Locator pattern. It's like having a central registry where you can fetch any service you need. Here's how you might implement it:
class ServiceLocator { private static $services = []; public static function set($key, $service) { self::$services[$key] = $service; } public static function get($key) { if (!isset(self::$services[$key])) { throw new Exception("Service not found: $key"); } return self::$services[$key]; } } class Logger { public function log($message) { echo $message . "\n"; } } class UserService { public function getUser($id) { $logger = ServiceLocator::get('logger'); $logger->log("Fetching user with id: $id"); // Fetch user logic here } } $logger = new Logger(); ServiceLocator::set('logger', $logger); $userService = new UserService(); $userService->getUser(1);
With the Service Locator, you don't need to pass dependencies around. You can fetch them from a central place. It's convenient, especially when you're dealing with a lot of services. But, it can lead to tighter coupling, and it might make your code harder to test because it's less explicit about what it needs.
From my experience, Dependency Injection shines in larger, more complex applications where you want to keep everything modular and testable. I've worked on projects where setting up a proper DI container feel like building a Lego castle, but it paid off in the long run with easier maintenance and better test coverage.
On the other hand, the Service Locator can be a quick fix for smaller applications or prototypes. It's like the fast food of dependency management – quick and easy but not always the healthiest choice for your codebase.
When choosing between the two, consider the following:
Testability : Dependency Injection makes it easier to write unit tests because you can easily mock dependencies. Service Locator can hide dependencies, making it harder to test in isolation.
Flexibility : DI allows you to change dependencies at runtime more easily. With a Service Locator, you might need to change the registry, which can be more cumbersome.
Complexity : DI might require more upfront setup, especially with a DI container, but it pays off in the long run. Service Locator can be simpler to implement but might lead to more complex code over time.
Code Readability : DI makes it clear what dependencies a class needs. Service Locator can obscure these dependencies, making it harder to understand the class's requirements at a glance.
One pitfall I've encountered with Dependency Injection is over-engineering. Sometimes, in the quest for perfect modularity, you end up with a DI configuration that's more complex than the application itself. It's important to find a balance and not go overboard with containers and abstractions.
With Service Locator, the main pitfall is the hidden dependencies. I once worked on a project where the Service Locator was used extensively, and it became a nightmare to debug because it was hard to track down where a service was being used.
In conclusion, both Dependency Injection and Service Locator have their place in PHP development. DI is my go-to for larger, more maintained applications, while Service Locator can be useful for quick prototypes or smaller projects. The key is to understand the trade-offs and choose the pattern that best fits your project's needs and your team's experience. Remember, the goal is to write clean, maintained code that stands the test of time.
The above is the detailed content of Dependency Injection vs. Service Locator in PHP. For more information, please follow other related articles on the PHP Chinese website!
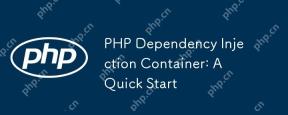
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
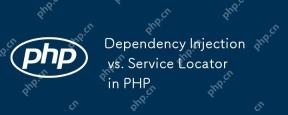
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
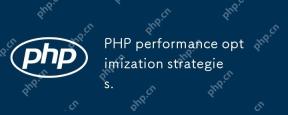
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
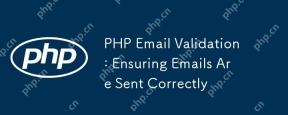
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
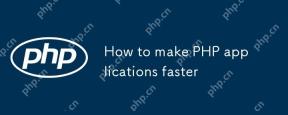
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
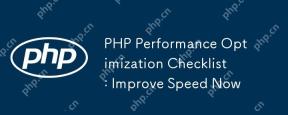
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
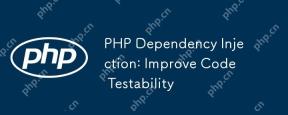
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
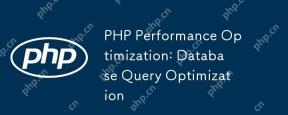
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
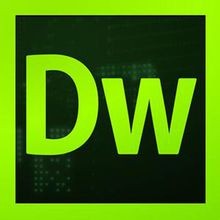
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
