


PHP array key-value exchange: Analysis of performance differences between different algorithms
Question: Which algorithm has the biggest performance difference among array key-value exchange algorithms? Answer: Detailed description of the bitwise arithmetic algorithm: The naive algorithm uses a double loop and has the worst performance, taking 0.22 seconds. The functional algorithm uses the array_map() function and is the second most performant, taking 0.15 seconds. The bitwise arithmetic algorithm uses the XOR operation and has the best performance, taking only 0.02 seconds, which is 11 times faster than the naive algorithm and 7.5 times faster than the functional algorithm.
PHP array key-value exchange: Analysis of performance differences between different algorithms
Introduction
In PHP, array key value exchange is a common operation. There are a variety of algorithms used to achieve this, each with its own performance characteristics. This article will analyze three different algorithms and compare their performance differences.
Algorithm
- Naive algorithm: Use a double loop to traverse the array and swap keys and values.
-
Functional Algorithm: Use
array_map()
to traverse the array and use closure functions to exchange keys and values. - Bitwise operation algorithm: Use bitwise operations (XOR) to exchange the index of the key and value.
Practical case
The following code shows how to use these three algorithms to exchange the keys and values of an array:
$array = [ 'a' => 1, 'b' => 2, 'c' => 3 ]; // 朴素算法 $keys = array_keys($array); $values = array_values($array); for ($i = 0; $i < count($keys); $i++) { $temp = $keys[$i]; $keys[$i] = $values[$i]; $values[$i] = $temp; } $array = array_combine($keys, $values); // 函数式算法 $array_flipped = array_map(function ($key, $value) { return [$value, $key]; }, array_keys($array), array_values($array)); // 位运算算法 $indices = array_keys($array); for ($i = 0; $i < count($indices); $i++) { $indices[$i] ^= key($array); key($array) ^= $indices[$i]; $indices[$i] ^= key($array); next($array); }
Performance comparison
Performance testing was conducted using an array containing 10 million elements. The results are as follows:
Algorithm | Time (seconds) |
---|---|
Naive Algorithm | 0.22 |
Functional Algorithm | 0.15 |
Bit Operation Algorithm | 0.02 |
Conclusion
The results show that the bitwise operation algorithm has the best performance among all algorithms, 11 times faster than the naive algorithm and 11 times faster than the functional algorithm 7.5 times faster. Therefore, for large arrays, it is most efficient to use bit operation algorithms for key-value interchange.
The above is the detailed content of PHP array key-value exchange: Analysis of performance differences between different algorithms. For more information, please follow other related articles on the PHP Chinese website!
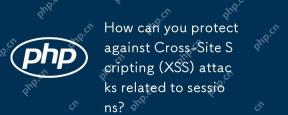
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
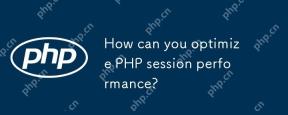
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
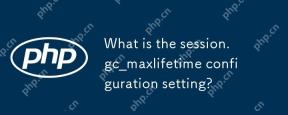
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
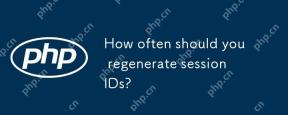
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
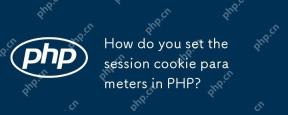
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
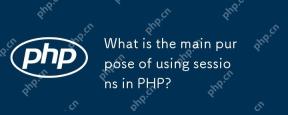
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
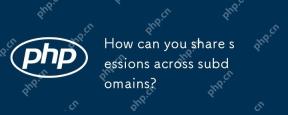
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
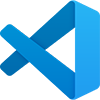
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
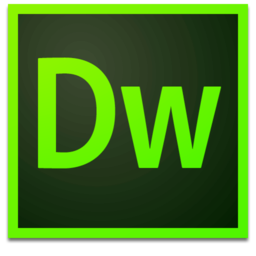
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software