In C language, there are three ways to express the nth power of a number: pow() function: used to calculate numbers with specified powers, with higher accuracy but slower speed. Power operator ^: only works with integer powers, faster but less precise. Loop: works with any power value, but is slower.
Representing the nth power of a number in C language
In C language, a number can be expressed in the following way Number raised to the nth power:
pow() function
pow()
The function is used to calculate the specified power of a given number. The syntax is as follows:
double pow(double base, double exponent);
Among them,
-
base
is the base (the number to be used to calculate the power) -
exponent
is the exponent (the value of the power)
For example: to calculate the third power of 2, you can write like this:
double result = pow(2, 3); // result 将等于 8
Power operator (^)
In C language, the exponentiation operator ^
can be used to calculate the integer power of a number. The syntax is as follows:
int base ^ exponent;
Among them,
-
base
is the base (the number to be used to calculate the power) -
exponent
is the exponent (the value of the power)
Note: The power operator can only be used to calculate integer powers.
For example: to calculate the 3rd power of 2, you can write like this:
int result = 2 ^ 3; // result 将等于 8
Use a loop
For non-integer powers or situations where precise calculations are required , you can use a loop to represent the nth power of a number.
For example: to calculate 2 raised to the 2.5th power, you can write like this:
double base = 2; double exponent = 2.5; double result = 1; for (int i = 0; i < exponent; i++) { result *= base; }
Compare
-
pow()
The function is faster and more accurate. - The exponentiation operator can only calculate integer powers, but it is faster.
- The loop method works with any power value, but is slower.
The above is the detailed content of How to express the nth power of a number in C language. For more information, please follow other related articles on the PHP Chinese website!
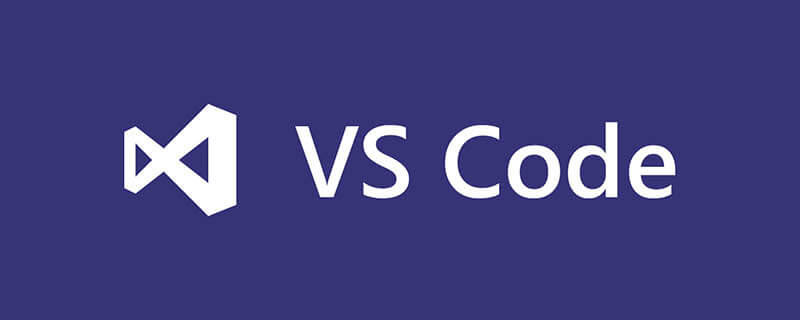
VScode中怎么配置C语言环境?下面本篇文章给大家介绍一下VScode配置C语言环境的方法(超详细),希望对大家有所帮助!
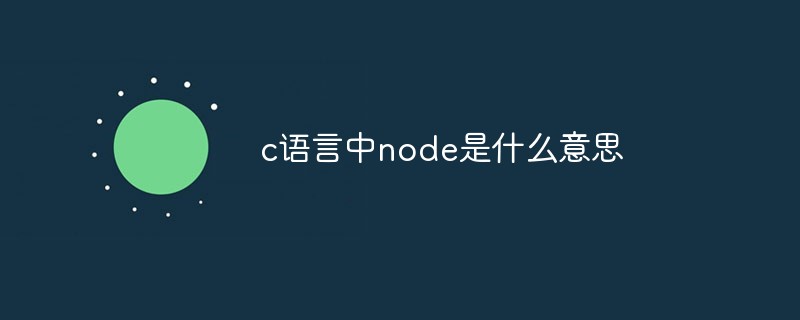
在C语言中,node是用于定义链表结点的名称,通常在数据结构中用作结点的类型名,语法为“struct Node{...};”;结构和类在定义出名称以后,直接用该名称就可以定义对象,C语言中还存在“Node * a”和“Node* &a”。

c语言将数字转换成字符串的方法:1、ascii码操作,在原数字的基础上加“0x30”,语法“数字+0x30”,会存储数字对应的字符ascii码;2、使用itoa(),可以把整型数转换成字符串,语法“itoa(number1,string,数字);”;3、使用sprintf(),可以能够根据指定的需求,格式化内容,存储至指针指向的字符串。
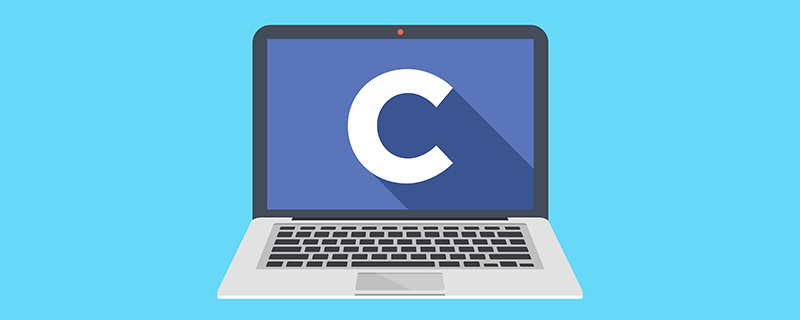
在c语言中,没有开根号运算符,开根号使用的是内置函数“sqrt()”,使用语法“sqrt(数值x)”;例如“sqrt(4)”,就是对4进行平方根运算,结果为2。sqrt()是c语言内置的开根号运算函数,其运算结果是函数变量的算术平方根;该函数既不能运算负数值,也不能输出虚数结果。

C语言数组初始化的三种方式:1、在定义时直接赋值,语法“数据类型 arrayName[index] = {值};”;2、利用for循环初始化,语法“for (int i=0;i<3;i++) {arr[i] = i;}”;3、使用memset()函数初始化,语法“memset(arr, 0, sizeof(int) * 3)”。
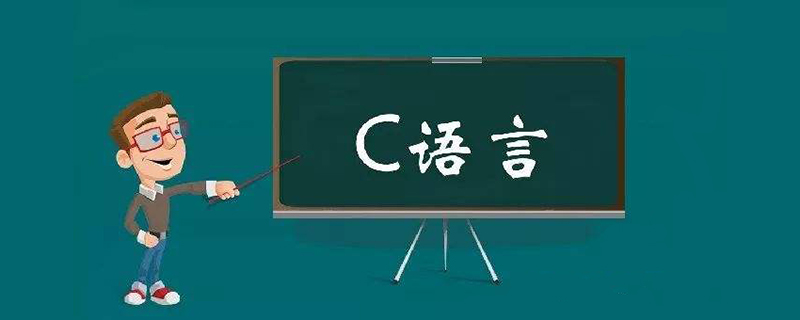
c语言合法标识符的要求是:1、标识符只能由字母(A~Z, a~z)、数字(0~9)和下划线(_)组成;2、第一个字符必须是字母或下划线,不能是数字;3、标识符中的大小写字母是有区别的,代表不同含义;4、标识符不能是关键字。
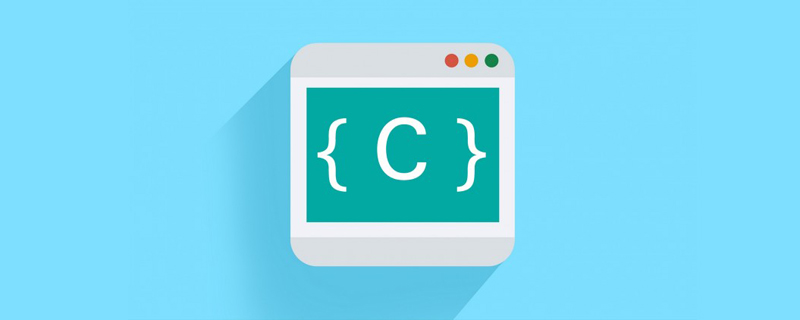
c语言编译后生成“.OBJ”的二进制文件(目标文件)。在C语言中,源程序(.c文件)经过编译程序编译之后,会生成一个后缀为“.OBJ”的二进制文件(称为目标文件);最后还要由称为“连接程序”(Link)的软件,把此“.OBJ”文件与c语言提供的各种库函数连接在一起,生成一个后缀“.EXE”的可执行文件。
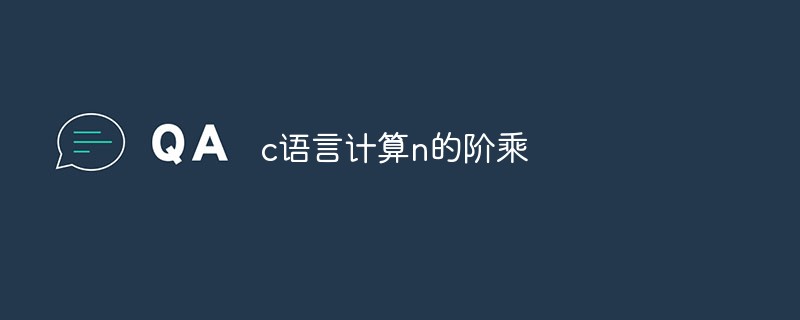
c语言计算n的阶乘的方法:1、通过for循环计算阶乘,代码如“for (i = 1; i <= n; i++){fact *= i;}”;2、通过while循环计算阶乘,代码如“while (i <= n){fact *= i;i++;}”;3、通过递归方式计算阶乘,代码如“ int Fact(int n){int res = n;if (n > 1)res...”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
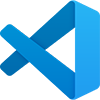
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
