The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
introduction
The combination of C# and .NET frameworks provides developers with a powerful programming environment that you can benefit greatly from whether you are a beginner or a senior programmer. Today we will dive into C# and .NET to uncover their mystery. After reading this article, you will have a comprehensive understanding of the syntax of C#, the .NET ecosystem, and their application scenarios, and you will find how interesting and practical they are.
Review of basic knowledge
C# is an object-oriented programming language developed by Microsoft. It is syntactically influenced by C and Java, but it has its own uniqueness. .NET is a development platform launched by Microsoft that supports multiple programming languages, among which C# is one of the main forces. .NET provides a huge class library and runtime environment, allowing developers to easily create applications of all types, from desktop applications to web services to mobile applications.
Before we start to dive into it, let's take a look at the basic syntax of C# and the core concepts of .NET. The syntax of C# is concise and clear, and supports the characteristics of modern programming languages such as garbage collection, type safety and exception handling. .NET provides developers with a unified execution environment through its common language runtime (CLR) and common language infrastructure (CLI), so that code written in different languages can interact seamlessly.
Core concept or function analysis
Polymorphism of C# and cross-platform capabilities of .NET
The polymorphism of C# is an important concept in object-oriented programming. It allows you to call the specific implementation of derived classes through a base class reference. This is well supported in .NET, making the code more flexible and maintainable. Let's look at a simple example:
// Polymorphic example public class Shape { public virtual void Draw() { Console.WriteLine("Drawing a shape"); } } public class Circle: Shape { public override void Draw() { Console.WriteLine("Drawing a circle"); } } public class Rectangle : Shape { public override void Draw() { Console.WriteLine("Drawing a rectangle"); } } class Program { static void Main(string[] args) { Shape shape1 = new Circle(); Shape shape2 = new Rectangle(); shape1.Draw(); // Output: Drawing a circle shape2.Draw(); // Output: Drawing a rectangle } }
.NET's cross-platform capabilities are implemented through .NET Core and .NET 5 and later, allowing developers to deploy C# applications to various operating systems such as Windows, Linux and macOS. This greatly expands the scope of C# and .NET applications, making them no longer limited to Windows platforms.
Asynchronous programming of C# and concurrent processing of .NET
C#'s asynchronous programming model (async/await) allows developers to easily write efficient asynchronous code, avoid blocking the main thread and improve application responsiveness. .NET provides rich concurrency processing mechanisms, such as Task Parallel Library (TPL) and Parallel LINQ (PLINQ), allowing developers to make full use of the performance of multi-core processors.
// Asynchronous programming example public async Task<string> DownloadContentAsync(string url) { using (HttpClient client = new HttpClient()) { string content = await client.GetStringAsync(url); return content; } } class Program { static async Task Main(string[] args) { string result = await DownloadContentAsync("https://example.com"); Console.WriteLine(result); } }
Example of usage
Basic usage
The basic usage of C# includes variable declaration, control structure, method definition, etc. Let's look at a simple example:
// Basic usage example int number = 10; if (number > 5) { Console.WriteLine("The number is greater than 5"); } else { Console.WriteLine("The number is less than or equal to 5"); } void SayHello(string name) { Console.WriteLine($"Hello, {name}!"); } SayHello("World");
Advanced Usage
Advanced usage of C# includes LINQ queries, generic programming, delegates and events, etc. Let's look at an example using LINQ query:
// LINQ query example List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; var evenNumbers = numbers.Where(n => n % 2 == 0).Select(n => n * 2); foreach (var number in evenNumbers) { Console.WriteLine(number); }
Common Errors and Debugging Tips
When using C# and .NET, common errors include null reference exceptions, type conversion errors, deadlocks in asynchronous programming, etc. Methods to debug these problems include using Visual Studio's debugging tools, adding logging, using exception handling mechanisms, etc.
Performance optimization and best practices
In practical applications, optimizing the performance of C# and .NET code is an important topic. The performance of the code can be improved by using appropriate data structures, avoiding unnecessary memory allocation, and leveraging asynchronous programming and concurrent processing. Let's look at an example of performance optimization:
// Performance optimization example// Use StringBuilder instead of string splicing StringBuilder sb = new StringBuilder(); for (int i = 0; i < 10000; i ) { sb.Append(i); } string result = sb.ToString();
Following best practices when writing C# and .NET code can improve the readability and maintenance of your code. For example, use meaningful variable names and method names, write clear comments, follow SOLID principles, etc.
Overall, C# and .NET are a powerful combination that provides developers with rich tools and features. Whether you are just starting to learn programming or already have extensive development experience, you can benefit from it. I hope this article can help you better understand and apply C# and .NET, and I wish you greater success on the road of programming!
The above is the detailed content of C# .NET: An Introduction to the Powerful Programming Language. For more information, please follow other related articles on the PHP Chinese website!
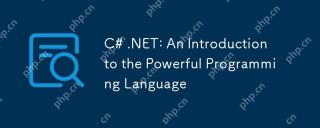
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
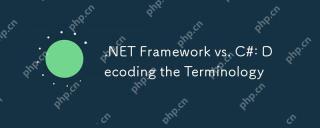
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
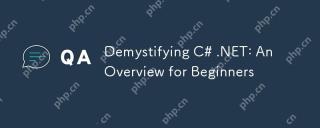
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
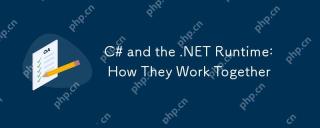
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
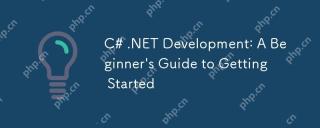
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
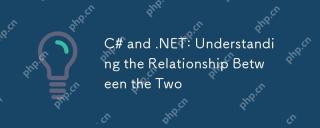
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
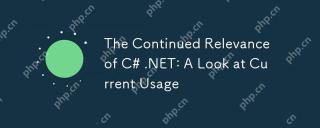
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
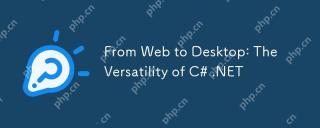
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.