.NET Framework is Windows-centric, while .NET Core/5/6 supports cross-platform development. 1) .NET Framework, since 2002, is ideal for Windows applications but limited in cross-platform capabilities. 2) .NET Core, from 2016, and its evolutions (.NET 5/6) offer better performance, cross-platform support, and modern features, suitable for cloud and containerized applications.
When diving into the world of C# and .NET, one often encounters the question: What's the difference between .NET Framework and .NET Core/5/6? Let's unravel this mystery and explore the nuances that set these platforms apart.
In my journey as a developer, I've seen the evolution of .NET firsthand. The transition from .NET Framework to .NET Core and its subsequent versions has been both exciting and challenging. Let's dive into the details, starting with a brief overview of each.
.NET Framework is the original platform that many of us grew up coding with. It's been around since 2002 and has a rich ecosystem of libraries and tools. It's primarily designed for Windows, making it a go-to choice for desktop and server applications on the Windows platform. However, its Windows-centric nature limits its cross-platform capabilities.
On the other hand, .NET Core, introduced in 2016, marked a significant shift towards cross-platform development. It's lean, fast, and designed to run on Windows, Linux, and macOS. With the release of .NET 5 in 2020 and .NET 6 in 2021, the platform has continued to evolve, merging .NET Core with other .NET technologies into a single, unified .NET platform. This new .NET is not just about cross-platform; it's about performance, cloud readiness, and modern application development.
Now, let's explore the key differences and considerations between these platforms.
Performance and Cross-Platform Capabilities
.NET Core and its successors (.NET 5/6) are built with performance in mind. They're optimized for speed and efficiency, which is crucial in today's cloud-centric world. I've seen firsthand how .NET Core applications can outperform their .NET Framework counterparts, especially in high-load scenarios.
Cross-platform development is another game-changer. With .NET Core/5/6, you can write your code once and deploy it on Windows, Linux, or macOS. This flexibility is invaluable for teams working in diverse environments or targeting multiple platforms. I've worked on projects where this ability to run on different OSes was a major selling point for clients.
Here's a simple example of a cross-platform console application using .NET 6:
using System; class Program { static void Main(string[] args) { Console.WriteLine("Hello, Cross-Platform World!"); } }
This code can be compiled and run on any supported platform, showcasing the power of .NET 6.
Ecosystem and Libraries
.NET Framework has a vast ecosystem built over nearly two decades. Many legacy applications and libraries are still dependent on it. However, this can also be a double-edged sword. I've encountered situations where finding compatible libraries for newer .NET versions was challenging due to the reliance on older frameworks.
.NET Core/5/6, while initially having a smaller ecosystem, has been rapidly catching up. Microsoft and the community have been porting many popular libraries to .NET Core and beyond. The NuGet package manager has been a crucial tool in this transition, making it easier to find and use modern libraries.
Deployment and Containerization
One of the most exciting aspects of .NET Core/5/6 is its support for containerization. Docker integration is seamless, allowing you to package your application and its dependencies into a container that can run anywhere. This is particularly useful for microservices architectures, which I've implemented in several projects.
Here's an example of a Dockerfile for a .NET 6 application:
FROM mcr.microsoft.com/dotnet/sdk:6.0 AS build WORKDIR /app COPY *.csproj ./ RUN dotnet restore COPY . ./ RUN dotnet publish -c Release -o out FROM mcr.microsoft.com/dotnet/aspnet:6.0 AS runtime WORKDIR /app COPY --from=build /app/out ./ ENTRYPOINT ["dotnet", "MyApp.dll"]
This Dockerfile demonstrates how easy it is to containerize a .NET 6 application, a feature that's not as straightforward with .NET Framework.
Performance Optimization and Best Practices
When optimizing for performance, .NET Core/5/6 offers several advantages. The Just-In-Time (JIT) compiler in .NET Core is more efficient, and the runtime is designed to take advantage of modern hardware. I've seen significant performance gains by migrating applications from .NET Framework to .NET Core.
However, it's not just about the platform; it's also about how you use it. Here are some best practices I've learned:
- Use async/await: Asynchronous programming can significantly improve the responsiveness of your application, especially in I/O-bound operations.
-
Leverage Span
and Memory : These types can help reduce memory allocations and improve performance in scenarios involving large data sets. - Profile and Benchmark: Use tools like BenchmarkDotNet to measure and optimize your code's performance.
Here's an example of using async/await in .NET 6:
using System; using System.Threading.Tasks; class Program { static async Task Main(string[] args) { await DoSomethingAsync(); Console.WriteLine("Task completed."); } static async Task DoSomethingAsync() { await Task.Delay(1000); // Simulate some async work Console.WriteLine("Async operation finished."); } }
Common Pitfalls and Debugging Tips
When transitioning from .NET Framework to .NET Core/5/6, there are a few common pitfalls to watch out for:
- Dependency Issues: Some libraries might not be compatible with .NET Core/5/6. Always check the compatibility of your dependencies before migrating.
- Configuration Changes: The configuration system in .NET Core/5/6 is different from .NET Framework. Be prepared to update your configuration files and code.
- Platform-Specific Code: If you're using platform-specific APIs, you'll need to refactor them to work across different operating systems.
For debugging, I recommend using Visual Studio's built-in tools, which have excellent support for .NET Core/5/6. Additionally, tools like dotnet-trace and dotnet-dump can be invaluable for diagnosing issues in production environments.
In conclusion, the choice between .NET Framework and .NET Core/5/6 depends on your project's requirements. If you need cross-platform support, better performance, and modern features, .NET Core/5/6 is the way to go. However, if you're maintaining a legacy application or need specific Windows-only features, .NET Framework might still be the better choice. As a developer, embracing the new while understanding the old is key to navigating this evolving landscape.
The above is the detailed content of C# .NET Framework vs. .NET Core/5/6: What's the Difference?. For more information, please follow other related articles on the PHP Chinese website!
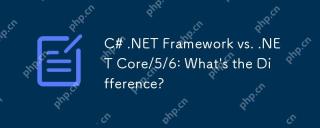
.NETFrameworkisWindows-centric,while.NETCore/5/6supportscross-platformdevelopment.1).NETFramework,since2002,isidealforWindowsapplicationsbutlimitedincross-platformcapabilities.2).NETCore,from2016,anditsevolutions(.NET5/6)offerbetterperformance,cross-
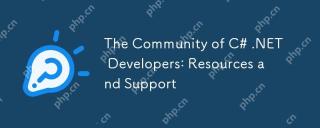
The C#.NET developer community provides rich resources and support, including: 1. Microsoft's official documents, 2. Community forums such as StackOverflow and Reddit, and 3. Open source projects on GitHub. These resources help developers improve their programming skills from basic learning to advanced applications.
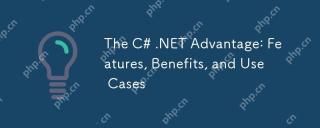
The advantages of C#.NET include: 1) Language features, such as asynchronous programming simplifies development; 2) Performance and reliability, improving efficiency through JIT compilation and garbage collection mechanisms; 3) Cross-platform support, .NETCore expands application scenarios; 4) A wide range of practical applications, with outstanding performance from the Web to desktop and game development.
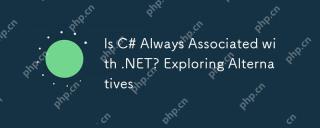
C# is not always tied to .NET. 1) C# can run in the Mono runtime environment and is suitable for Linux and macOS. 2) In the Unity game engine, C# is used for scripting and does not rely on the .NET framework. 3) C# can also be used for embedded system development, such as .NETMicroFramework.
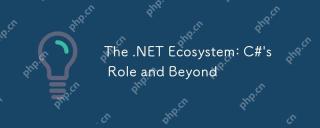
C# plays a core role in the .NET ecosystem and is the preferred language for developers. 1) C# provides efficient and easy-to-use programming methods, combining the advantages of C, C and Java. 2) Execute through .NET runtime (CLR) to ensure efficient cross-platform operation. 3) C# supports basic to advanced usage, such as LINQ and asynchronous programming. 4) Optimization and best practices include using StringBuilder and asynchronous programming to improve performance and maintainability.
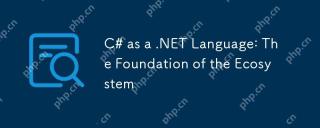
C# is a programming language released by Microsoft in 2000, aiming to combine the power of C and the simplicity of Java. 1.C# is a type-safe, object-oriented programming language that supports encapsulation, inheritance and polymorphism. 2. The compilation process of C# converts the code into an intermediate language (IL), and then compiles it into machine code execution in the .NET runtime environment (CLR). 3. The basic usage of C# includes variable declarations, control flows and function definitions, while advanced usages cover asynchronous programming, LINQ and delegates, etc. 4. Common errors include type mismatch and null reference exceptions, which can be debugged through debugger, exception handling and logging. 5. Performance optimization suggestions include the use of LINQ, asynchronous programming, and improving code readability.
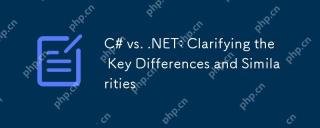
C# is a programming language, while .NET is a software framework. 1.C# is developed by Microsoft and is suitable for multi-platform development. 2..NET provides class libraries and runtime environments, and supports multilingual. The two work together to build modern applications.
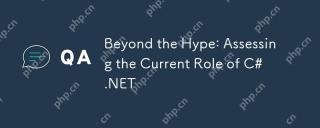
C#.NET is a powerful development platform that combines the advantages of the C# language and .NET framework. 1) It is widely used in enterprise applications, web development, game development and mobile application development. 2) C# code is compiled into an intermediate language and is executed by the .NET runtime environment, supporting garbage collection, type safety and LINQ queries. 3) Examples of usage include basic console output and advanced LINQ queries. 4) Common errors such as empty references and type conversion errors can be solved through debuggers and logging. 5) Performance optimization suggestions include asynchronous programming and optimization of LINQ queries. 6) Despite the competition, C#.NET maintains its important position through continuous innovation.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
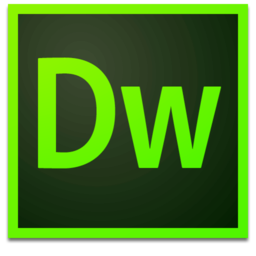
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
