


How to deploy a C# .NET app to Azure or AWS? The answer is to use Azure App Service and AWS Elastic Beanstalk. 1. On Azure, automate deployment with Azure App Service and Azure Pipelines. 2. On AWS, use Amazon Elastic Beanstalk and AWS Lambda to implement deployment and serverless compute.
introduction
If you ask me how to deploy a C# .NET app to Azure or AWS, I would say it is a challenging and exciting process. Today, I will take you on this journey and unveil the mystery of cloud deployment. We'll dive into how to seamlessly migrate C# .NET applications to Azure and AWS, ensuring you can not only deploy successfully, but also learn practical tips and avoid common pitfalls.
In this article, you will learn how to configure your development environment, how to use Azure and AWS services to host your applications, and how to optimize your deployment process. Whether you are a beginner or an experienced developer, I hope this article will bring you new insights and practical guidance.
Review of basic knowledge
Before we start, we will quickly review the basics needed. C# is a powerful and flexible programming language developed by Microsoft and is mainly used in the .NET framework. .NET is an open source development platform that supports a variety of programming languages, including C#, F# and VB.NET. Azure and AWS are cloud computing platforms for Microsoft and Amazon, respectively, and they provide a variety of services to support the development, deployment and management of applications.
If you are already familiar with these concepts, then we can go straight to the core content. If you don't know much about this, you can take some time to read some basic tutorials so that you will be easier to understand what's going on.
Core concept or function analysis
Deploy C# .NET apps to Azure
The most straightforward way to deploy C# .NET applications to Azure is to use Azure App Service. Azure App Service is a fully managed Platform as a Service (PaaS) that supports a variety of programming languages and frameworks, including .NET.
// Example: Deploy a simple C# .NET application using Microsoft.AspNetCore.Builder in Azure App Service; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.DependencyInjection; public class Startup { public void ConfigureServices(IServiceCollection services) { services.AddControllers(); } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } }
This example shows how to configure a basic ASP.NET Core application. To deploy this app to Azure App Service, you just need to push the code to Azure DevOps or GitHub and configure Azure Pipelines to automate the deployment process.
Deploy C# .NET applications to AWS
The common method to deploy C# .NET applications on AWS is to use Amazon Elastic Beanstalk. Elastic Beanstalk is a PaaS service that can automatically handle application deployment, capacity configuration, load balancing, etc.
// Example: Deploy a simple C# .NET application using Amazon.Lambda.Core in AWS Elastic Beanstalk; using Amazon.Lambda.APIGatewayEvents; public class Function { public APIGatewayProxyResponse FunctionHandler(APIGatewayProxyRequest request, ILambdaContext context) { var response = new APIGatewayProxyResponse { StatusCode = 200, Body = "Hello from AWS Lambda!" }; return response; } }
This example shows how to use AWS Lambda to handle HTTP requests. To deploy this app to Elastic Beanstalk, you need to package the code into a ZIP file and upload it to Elastic Beanstalk through the AWS Management Console or the AWS CLI.
How it works
On Azure, App Service automatically detects your app type and launches and manages your app based on your configuration files such as web.config
or appsettings.json
. Azure Pipelines automatically builds your code and deploys the build products to the App Service.
On AWS, Elastic Beanstalk configures the environment based on your application type (such as ASP.NET Core or .NET Framework) and automatically manages the deployment and extension of your application. AWS Lambda allows you to run code serverless and handle various event triggers.
Example of usage
Basic usage
The most basic way to deploy C# .NET applications on Azure is to use Azure App Service. You can use Azure DevOps or GitHub to manage your code and automate the deployment process with Azure Pipelines.
// Example: Deploy a simple C# .NET application using Microsoft.AspNetCore.Builder in Azure App Service; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.DependencyInjection; public class Startup { public void ConfigureServices(IServiceCollection services) { services.AddControllers(); } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } }
This example shows how to configure a basic ASP.NET Core application. To deploy this app to Azure App Service, you just need to push the code to Azure DevOps or GitHub and configure Azure Pipelines to automate the deployment process.
The most basic way to deploy C# .NET applications on AWS is to use Amazon Elastic Beanstalk. You can package the code into a ZIP file and upload it to Elastic Beanstalk via the AWS Management Console or the AWS CLI.
// Example: Deploy a simple C# .NET application using Amazon.Lambda.Core in AWS Elastic Beanstalk; using Amazon.Lambda.APIGatewayEvents; public class Function { public APIGatewayProxyResponse FunctionHandler(APIGatewayProxyRequest request, ILambdaContext context) { var response = new APIGatewayProxyResponse { StatusCode = 200, Body = "Hello from AWS Lambda!" }; return response; } }
This example shows how to use AWS Lambda to handle HTTP requests. To deploy this app to Elastic Beanstalk, you need to package the code into a ZIP file and upload it to Elastic Beanstalk through the AWS Management Console or the AWS CLI.
Advanced Usage
On Azure, you can leverage Azure Functions for serverless computing. Azure Functions allows you to run your code in an event-driven way, ideal for handling ephemeral, scalable tasks.
// Example: Using C# .NET in Azure Functions using Microsoft.Azure.WebJobs; using Microsoft.Azure.WebJobs.Host; using Microsoft.Extensions.Logging; public static void Run( [HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequestData req, FunctionContext executionContext) { var logger = executionContext.GetLogger("Function1"); logger.LogInformation("C# HTTP trigger function processed a request."); var response = req.CreateResponse(System.Net.HttpStatusCode.OK); response.WriteString("Welcome to Azure Functions!"); return response; }
This example shows how to use Azure Functions to handle HTTP requests. You can deploy this function to Azure Functions and automate the deployment process through Azure DevOps or GitHub.
On AWS, you can leverage AWS Lambda for serverless computing. AWS Lambda allows you to run your code in an event-driven way, ideal for handling ephemeral, scalable tasks.
// Example: Using C# .NET in AWS Lambda using Amazon.Lambda.Core; using Amazon.Lambda.APIGatewayEvents; public class Function { public APIGatewayProxyResponse FunctionHandler(APIGatewayProxyRequest request, ILambdaContext context) { var response = new APIGatewayProxyResponse { StatusCode = 200, Body = "Welcome to AWS Lambda!" }; return response; } }
This example shows how to use AWS Lambda to handle HTTP requests. You can deploy this function to AWS Lambda and automate the deployment process through the AWS Management Console or the AWS CLI.
Common Errors and Debugging Tips
When deploying C# .NET apps to Azure or AWS, you may encounter some common errors and problems. Here are some common errors and their solutions:
-
Connection string error : In Azure App Service, make sure your connection string is correctly configured in the
appsettings.json
orweb.config
file. If the connection string is wrong, the application may not be able to connect to the database.- Workaround: Check your connection strings, make sure they are correct, and update them in the Azure portal.
-
Dependency Issue : In AWS Elastic Beanstalk, if your application depends on certain NuGet packages, make sure that these packages are properly referenced in your project and installed correctly when deployed.
- Workaround: Check your
csproj
file, make sure all dependencies are listed correctly, and run thedotnet restore
command before deployment.
- Workaround: Check your
-
Permissions Issue : In Azure Functions or AWS Lambda, if your function needs access to certain resources, make sure your function has the corresponding permissions.
- Workaround: In the Azure portal or AWS Management Console, check and configure permissions for your functions to make sure they have access to the resources they need.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance and deployment process of your C# .NET application. Here are some recommendations for optimization and best practices:
Use Azure Pipelines or AWS CodePipeline : Automating your deployment process can greatly improve efficiency and reliability. Azure Pipelines and AWS CodePipeline both provide powerful CI/CD capabilities to help you automate the build, test, and deployment process.
Monitoring and Logging : Both on Azure and AWS provide powerful monitoring and logging services such as Azure Monitor and AWS CloudWatch. With these services, you can monitor the performance and health of your application in real time and quickly discover and resolve issues.
Optimize database access : If your application depends on the database, make sure your database access is efficient. Using ORM tools such as Entity Framework Core and optimizing your queries can significantly improve the performance of your application.
Code readability and maintenance : It is very important to write clear and readable code. Using meaningful variable and method names, adding detailed comments, and following code style guides can improve the maintainability of your code.
Performance testing : It is very important to perform performance testing before deployment. You can use tools such as JMeter or Visual Studio to simulate large number of user access and test your application's performance under high loads.
With these optimizations and best practices, you can ensure that your C# .NET application runs more efficiently and reliably on Azure or AWS. I hope this article will bring you some new insights and practical guidance, and I wish you a smooth journey in the cloud deployment!
The above is the detailed content of Deploying C# .NET Applications to Azure/AWS: A Step-by-Step Guide. For more information, please follow other related articles on the PHP Chinese website!
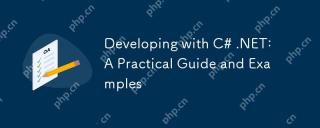
C# and .NET provide powerful features and an efficient development environment. 1) C# is a modern, object-oriented programming language that combines the power of C and the simplicity of Java. 2) The .NET framework is a platform for building and running applications, supporting multiple programming languages. 3) Classes and objects in C# are the core of object-oriented programming. Classes define data and behaviors, and objects are instances of classes. 4) The garbage collection mechanism of .NET automatically manages memory to simplify the work of developers. 5) C# and .NET provide powerful file operation functions, supporting synchronous and asynchronous programming. 6) Common errors can be solved through debugger, logging and exception handling. 7) Performance optimization and best practices include using StringBuild
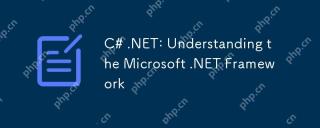
.NETFramework is a cross-language, cross-platform development platform that provides a consistent programming model and a powerful runtime environment. 1) It consists of CLR and FCL, which manages memory and threads, and FCL provides pre-built functions. 2) Examples of usage include reading files and LINQ queries. 3) Common errors involve unhandled exceptions and memory leaks, and need to be resolved using debugging tools. 4) Performance optimization can be achieved through asynchronous programming and caching, and maintaining code readability and maintainability is the key.
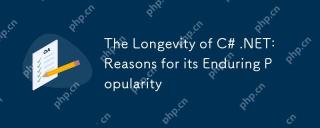
Reasons for C#.NET to remain lasting attractive include its excellent performance, rich ecosystem, strong community support and cross-platform development capabilities. 1) Excellent performance and is suitable for enterprise-level application and game development; 2) The .NET framework provides a wide range of class libraries and tools to support a variety of development fields; 3) It has an active developer community and rich learning resources; 4) .NETCore realizes cross-platform development and expands application scenarios.
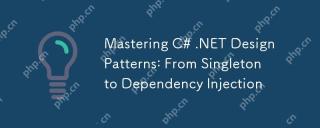
Design patterns in C#.NET include Singleton patterns and dependency injection. 1.Singleton mode ensures that there is only one instance of the class, which is suitable for scenarios where global access points are required, but attention should be paid to thread safety and abuse issues. 2. Dependency injection improves code flexibility and testability by injecting dependencies. It is often used for constructor injection, but it is necessary to avoid excessive use to increase complexity.
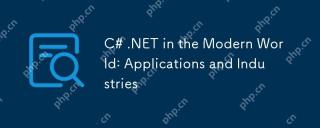
C#.NET is widely used in the modern world in the fields of game development, financial services, the Internet of Things and cloud computing. 1) In game development, use C# to program through the Unity engine. 2) In the field of financial services, C#.NET is used to develop high-performance trading systems and data analysis tools. 3) In terms of IoT and cloud computing, C#.NET provides support through Azure services to develop device control logic and data processing.
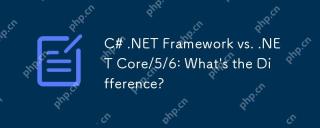
.NETFrameworkisWindows-centric,while.NETCore/5/6supportscross-platformdevelopment.1).NETFramework,since2002,isidealforWindowsapplicationsbutlimitedincross-platformcapabilities.2).NETCore,from2016,anditsevolutions(.NET5/6)offerbetterperformance,cross-
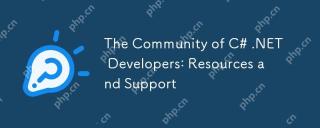
The C#.NET developer community provides rich resources and support, including: 1. Microsoft's official documents, 2. Community forums such as StackOverflow and Reddit, and 3. Open source projects on GitHub. These resources help developers improve their programming skills from basic learning to advanced applications.
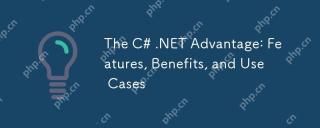
The advantages of C#.NET include: 1) Language features, such as asynchronous programming simplifies development; 2) Performance and reliability, improving efficiency through JIT compilation and garbage collection mechanisms; 3) Cross-platform support, .NETCore expands application scenarios; 4) A wide range of practical applications, with outstanding performance from the Web to desktop and game development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
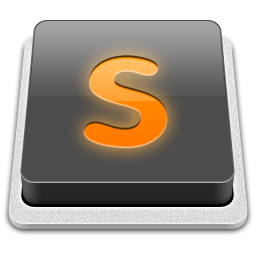
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
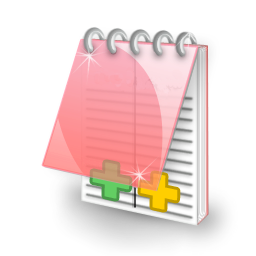
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
