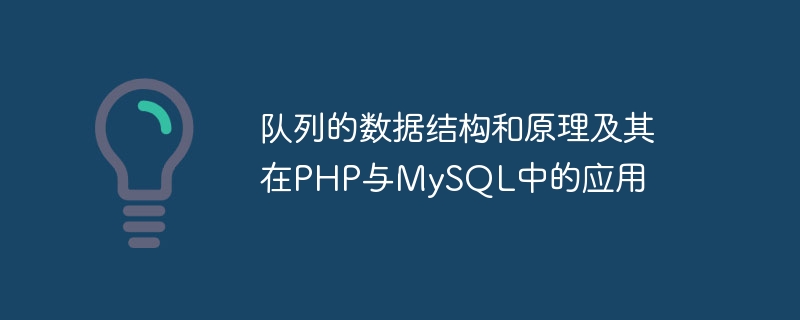
The data structure and principle of queue and its application in PHP and MySQL
- Introduction
Queue is a common data structure. Based on the first-in-first-out (FIFO) principle, it can be used to store and retrieve data, and can also implement task scheduling in a concurrent environment. In this article, we will explore the basic principles and data structures of queues, and illustrate its application in PHP and MySQL.
- The principle of queue
A queue is a linear data structure that contains an ordered collection of elements. The two main operations of a queue are enqueue and dequeue. The enqueue operation adds an element to the tail of the queue, while the dequeue operation removes an element from the head of the queue. Queues can be implemented using arrays or linked lists.
- Queue data structure
In PHP, we can use arrays to implement queues. The following is a simple PHP class that implements the basic operations of the queue:
class Queue {
private $queue;
function __construct() {
$this->queue = [];
}
function enqueue($item) {
array_push($this->queue, $item);
}
function dequeue() {
if ($this->isEmpty()) {
return null;
}
return array_shift($this->queue);
}
function isEmpty() {
return empty($this->queue);
}
}
- Queue Application Task Scheduling
In a concurrent environment, queues can be used for task scheduling. Suppose we have a list of tasks that need to be processed, each with a different execution time. We can use queues to schedule tasks according to their priority and order. The following is a simple example:
$taskQueue = new Queue();
$taskQueue->enqueue("Task 1");
$taskQueue->enqueue("Task 2");
$taskQueue->enqueue("Task 3");
while (!$taskQueue->isEmpty()) {
$task = $taskQueue->dequeue();
// 处理任务
echo "Processing task: " . $task . "
";
// 模拟任务执行时间
usleep(rand(100000, 500000));
}
- Application of Queue in MySQL Message Queue
Queue can also be used in MySQL database, especially when processing a large number of asynchronous tasks or When the message is delivered. We can use MySQL tables to simulate queue operations. Here is an example:
CREATE TABLE `message_queue` (
`id` INT(11) NOT NULL AUTO_INCREMENT,
`message` VARCHAR(255) NOT NULL,
PRIMARY KEY (`id`),
KEY `id_message_idx` (`id`, `message`)
) ENGINE=InnoDB;
-- 入队操作
INSERT INTO `message_queue` (`message`) VALUES ('Message 1');
INSERT INTO `message_queue` (`message`) VALUES ('Message 2');
-- 出队操作
SELECT `message` FROM `message_queue` ORDER BY `id` ASC LIMIT 1;
DELETE FROM `message_queue` ORDER BY `id` ASC LIMIT 1;
- Summary
Queue is an important data structure that can implement the first-in-first-out principle and implement task scheduling in a concurrent environment. In PHP, we can use arrays to implement basic queue operations. In MySQL, we can use tables to simulate queue operations. Proficient in the principles of queues and their applications in PHP and MySQL can improve the efficiency and maintainability of your code. Hope this article is helpful to you.
The above is the detailed content of The data structure and principle of queue and its application in PHP and MySQL. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn