How to resolve runtime errors and exceptions in PHP development
How to solve runtime errors and exceptions in PHP development
In the PHP development process, runtime errors and exceptions are frequently encountered problems. Handling these errors and exceptions is a key part of ensuring the stable operation of the program and improving development efficiency. This article describes some common runtime errors and exceptions and provides specific code examples to resolve them.
- Grammar Error
Grammar error is one of the most common errors, usually caused by spelling errors, grammatical errors or lack of necessary symbols in the code. The most effective way to solve this type of error is to use a good IDE (Integrated Development Environment) to check the code for syntax errors. At the same time, you can also view specific error information through the PHP error log.
Sample code:
//示例一:拼写错误 ech "Hello World"; //错误示例:ech应为echo //示例二:缺少必要的符号 if ($condition { //错误示例:缺少了一个右括号 echo "Condition is true."; }
- Class does not exist
PHP throws a fatal error when trying to instantiate a class that does not exist. The way to solve this type of error is to make sure that the file where the class is located is included before using theclass_exists
function to determine whether the class exists.
Sample code:
//判断类是否存在 if (class_exists('MyClass')) { //实例化类 $obj = new MyClass(); //调用类的方法 $obj->myMethod(); } else { echo "Class does not exist."; }
- Uncaught exceptions
Uncaught exceptions are a common cause of program interruption and error messages. In order to handle this type of exception, you can use the try-catch statement to catch and handle the exception. In the catch block, you can output error information, record error logs, or perform other processing.
Sample code:
try { //可能抛出异常的代码块 $result = 10 / 0; } catch (Exception $e) { //捕获异常并处理 echo "An error occurred: " . $e->getMessage(); //或者记录错误日志 error_log("An error occurred: " . $e->getMessage(), 0); }
- Array out-of-bounds access
When trying to access an array index that does not exist, PHP will throw a warning and return a null value. In order to avoid this type of problem, you can use theisset
function to determine whether the array index exists.
Sample code:
//定义一个数组 $data = array('A', 'B', 'C'); //访问不存在的数组索引 if (isset($data[5])) { echo $data[5]; //正常执行 } else { echo "Array index does not exist."; //提示索引不存在 }
- File operation error
In PHP, file operations are very common. However, file opening or read and write errors may occur due to file permission issues or file non-existence. In order to deal with this type of problem, you can use thefile_exists
function to first determine whether the file exists and then perform file operations.
Sample code:
//判断文件是否存在 if (file_exists('file.txt')) { //打开文件进行读写操作 $handle = fopen('file.txt', 'r'); //... fclose($handle); } else { echo "File does not exist."; }
Summary:
In PHP development, solving runtime errors and exceptions is very important. By using a good IDE to check for syntax errors, using the class to determine if the class has been included before it does not exist, using try-catch blocks to catch exceptions, using the isset function to determine whether the array index exists, and using the file_exists function to determine whether the file exists, you can Help us better deal with and solve these problems. At the same time, it is also a good habit to check PHP error logs regularly, which can help us discover and solve potential problems in time, ensuring program stability and performance optimization.
The above is the detailed content of How to resolve runtime errors and exceptions in PHP development. For more information, please follow other related articles on the PHP Chinese website!
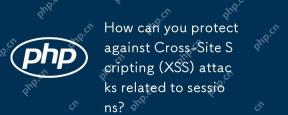
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
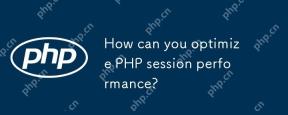
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
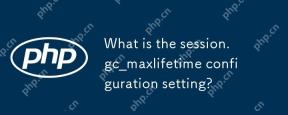
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
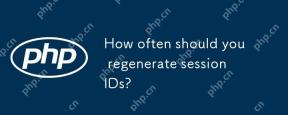
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
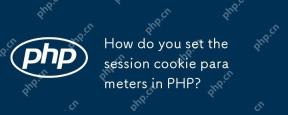
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
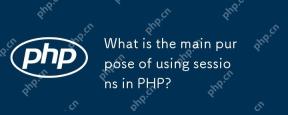
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
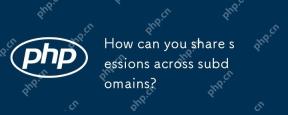
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
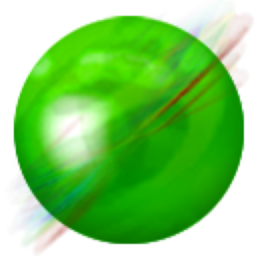
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
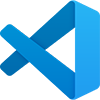
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version