


How to use PHP microservices to achieve distributed data synchronization and replication
How to use PHP microservices to achieve distributed data synchronization and replication
Introduction:
In a distributed system, data synchronization and replication are very important operations , which can ensure the consistency of data on different nodes. As a popular server-side scripting language, PHP can use microservice architecture to achieve distributed data synchronization and replication. This article will introduce in detail how to use PHP microservices to implement this function and provide specific code examples.
- Architecture Design
When designing a distributed data synchronization and replication system, the following aspects need to be considered: - Data storage: Choose an appropriate database or data storage Systems, such as MySQL, MongoDB, etc.
- Data synchronization: Choose an appropriate synchronization strategy, such as real-time synchronization, periodic synchronization, etc.
- Data replication: Choose an appropriate replication strategy, such as master-slave replication, multi-master replication, etc.
- Using PHP microservice framework
PHP microservice framework can help us quickly build a microservice architecture and provides some commonly used functions and components. In this article, we use the Lumen framework to implement distributed data synchronization and replication functions. The following is a simple example code to build the Lumen framework:
// index.php require_once __DIR__.'/vendor/autoload.php'; $app = new LaravelLumenApplication( realpath(__DIR__.'/../') ); $app->withFacades(); $app->router->group([ 'namespace' => 'AppHttpControllers', ], function ($router) { require __DIR__.'/../routes/web.php'; }); $app->run();
composer install
Install the required dependencies and run php -S localhost:8000 -t public
Start the Lumen framework.
- Achieve data synchronization
In order to achieve data synchronization, we can consider using message queues. When the data on a node changes, it will send the change information to the message queue, and other nodes can achieve data synchronization by subscribing to the message queue. Below is a simple example code using Redis as a message queue:
// app/Http/Controllers/ExampleController.php namespace AppHttpControllers; use IlluminateSupportFacadesRedis; use IlluminateHttpRequest; class ExampleController extends Controller { public function sync(Request $request) { $data = $request->all(); Redis::publish('data-sync', json_encode($data)); return response()->json(['message' => 'Sync successful']); } }
In the above code, when a synchronous request is received, we publish the data to Redis' data-sync
in the channel. Other nodes can achieve data synchronization by subscribing to this channel.
- Implement data replication
In order to achieve data replication, we can use the master-slave replication strategy. When the data on one node changes, it will send the change information to other nodes, and other nodes will process the change information accordingly after receiving the change information, thereby realizing data replication. The following is a simple example code using MySQL to implement master-slave replication:
// app/Http/Controllers/ExampleController.php namespace AppHttpControllers; use IlluminateSupportFacadesDB; use IlluminateHttpRequest; class ExampleController extends Controller { public function duplicate(Request $request) { $data = $request->all(); DB::table('example_table')->insert($data); return response()->json(['message' => 'Duplicate successful']); } }
In the above code, we use the DB Facade provided by Laravel to perform database operations. When executing a data copy request, we insert data into the example_table
table of the database.
Conclusion:
By using the PHP microservices framework and appropriate data synchronization and replication strategies, we can achieve distributed data synchronization and replication functions. This article provides specific code using the Lumen framework, Redis, and MySQL as examples for readers' reference and learning. Of course, the above example is just a simple implementation method and should be adjusted and improved according to actual needs. I hope this article is helpful to readers, thank you for reading!
(Note: The above code examples are for reference only, and need to be adjusted and improved according to specific circumstances in actual applications.)
The above is the detailed content of How to use PHP microservices to achieve distributed data synchronization and replication. For more information, please follow other related articles on the PHP Chinese website!
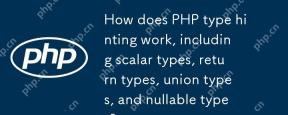
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
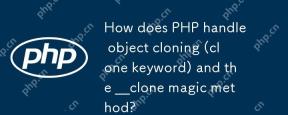
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
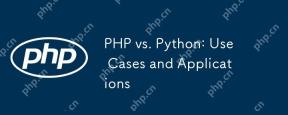
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
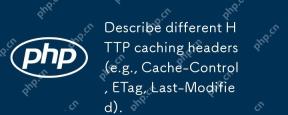
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
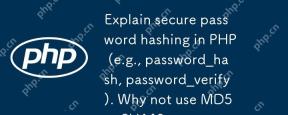
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
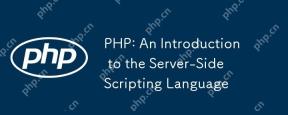
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
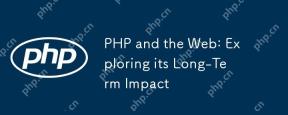
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
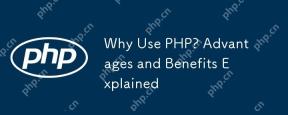
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
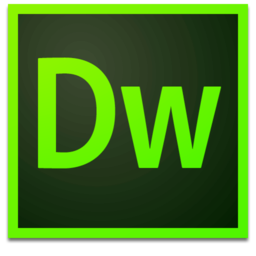
Dreamweaver Mac version
Visual web development tools
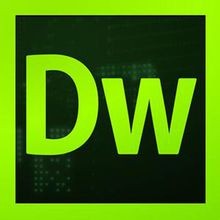
Dreamweaver CS6
Visual web development tools