How to write a simple file upload and download function via PHP
How to write a simple file upload and download function through PHP
With the development of Internet technology, file upload and download functions have become essential functions for many websites one. By writing a simple file upload and download function, users can easily upload and download files and improve user experience. This article will introduce how to use PHP to write a simple file upload and download function, and provide specific code examples.
1. Implementation of the file upload function
-
Create a form
First, create a form on the HTML page for users to upload files. The code of the form is as follows:<form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="file"> <input type="submit" value="上传"> </form>
Among them, the
action
attribute of the form tag specifies the processing script after the form is submitted asupload.php
,method The
attribute specifies the form submission method as POST, and theenctype
attribute specifies the form encoding type asmultipart/form-data
to support file upload. -
Write the upload script
In theupload.php
file, write the PHP code to handle the file upload. The code is as follows:<?php $targetDir = "uploads/"; // 上传文件存储目录 $targetFile = $targetDir . basename($_FILES["file"]["name"]); // 上传文件的路径 // 检查文件类型 $fileType = strtolower(pathinfo($targetFile, PATHINFO_EXTENSION)); $allowedTypes = array("jpg", "jpeg", "png", "gif"); if (!in_array($fileType, $allowedTypes)) { echo "只允许上传图片文件!"; exit; } // 将文件从临时目录移动到指定目录 if (move_uploaded_file($_FILES["file"]["tmp_name"], $targetFile)) { echo "文件上传成功!"; } else { echo "文件上传失败!"; } ?>
In the above code, a variable
$targetDir
is first defined to specify the storage directory after the file is uploaded, and then thebasename()
function is used Get the path of the uploaded file, then use thestrtolower()
function to convert the file type to lowercase, use thepathinfo()
function to get the extension of the file, then check if the file extension is allowed within the type range. If the file type does not meet the requirements, a prompt message is output and the program is terminated. If the file type meets the requirements, use themove_uploaded_file()
function to move the file from the temporary directory to the specified directory.
2. Implementation of the file download function
-
Create a file list
First, you need to display a list of files available for download on the page . The code is as follows:<?php $files = glob("uploads/*"); // 获取上传文件的列表 foreach ($files as $file) { $fileName = basename($file); echo "<a href='download.php?file={$fileName}'>{$fileName}</a><br>"; } ?>
In the above code, use the
glob()
function to get the list of uploaded files, then use thebasename()
function to get the file name, and finally Useecho
to output a list of files and generate a download link for each file. -
Write download script
In thedownload.php
file, write PHP code to download the file. The code is as follows:<?php $fileName = $_GET["file"]; // 获取要下载的文件名 $filePath = "uploads/" . $fileName; // 要下载的文件路径 if (file_exists($filePath)) { header("Content-Type: application/octet-stream"); header("Content-Disposition: attachment; filename={$fileName}"); readfile($filePath); } else { echo "文件不存在!"; } ?>
In the above code, the
$_GET
super global array is first used to obtain the file name to be downloaded, and then the path of the file is spliced based on the file name. Next, use thefile_exists()
function to check whether the file exists. If the file exists, set the HTTP header information so that the browser downloads the file as an attachment, and then use thereadfile()
function to The file is output to the browser. If the file does not exist, a prompt message is output.
The above is a sample code for writing a simple file upload and download function through PHP. Through these codes, we can easily implement file upload and download functions and improve the user experience of the website. Of course, this is just a simple example, and more security and performance optimization issues may need to be considered in actual development, but these sample codes can serve as the basis for getting started and learning, helping beginners quickly master the principles and implementation of file upload and download. Way.
The above is the detailed content of How to write a simple file upload and download function via PHP. For more information, please follow other related articles on the PHP Chinese website!
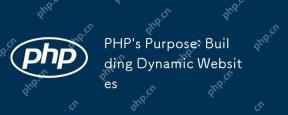
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
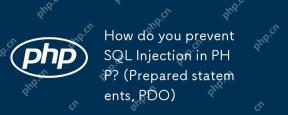
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
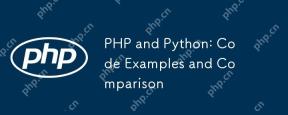
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
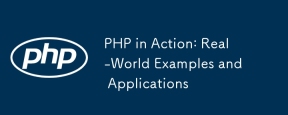
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
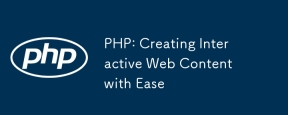
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
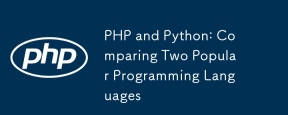
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
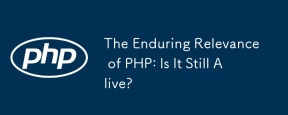
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
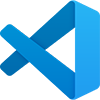
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
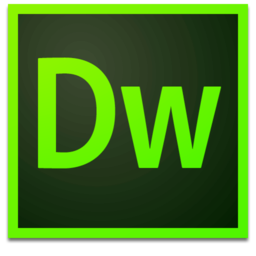
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor