How to use PHP to develop a simple picture filter function?
Introduction: In web development, adding image filters can add some interest to the user's visual experience. This article will introduce how to use PHP to develop a simple picture filter function and provide specific code examples.
- Ensure environment configuration
Before you start, make sure you have correctly configured the PHP environment and installed the GD library, because we will use the GD library to process images. You can enable the GD library in the php.ini file or select the GD library when installing PHP. - Load the original image
First, we need to load the original image to be filtered. Use the imagecreatefromjpeg() function in the code and pass in the path of the image file as a parameter to load a .jpeg format image. If you want to load images in other formats, you need to change the corresponding functions.
$sourceImage = imagecreatefromjpeg("path/to/source/image.jpg");
- Create target image
Next, we need to create a new target image and set its width, height and background color. You can use the imagecreatetruecolor() function to create a true color blank picture and set its width, height and background color.
$targetImage = imagecreatetruecolor(imagesx($sourceImage), imagesy($sourceImage)); $backgroundColor = imagecolorallocate($targetImage, 255, 255, 255); imagefill($targetImage, 0, 0, $backgroundColor);
- Apply filter effects
We can use different functions provided by the GD library to achieve different filter effects. Here are a few common filter examples:
- Grayscale filter
Grayscale filter can convert color pictures into black and white grayscale pictures. This can be achieved using the imagefilter() function and passing in the parameter IMG_FILTER_GRAYSCALE.
imagefilter($sourceImage, IMG_FILTER_GRAYSCALE);
- Inversion filter
The inversion filter can invert the color of the picture, that is, black becomes white, and white becomes black. This can be achieved using the imagefilter() function and passing in the parameter IMG_FILTER_NEGATE.
imagefilter($sourceImage, IMG_FILTER_NEGATE);
- Nostalgic Filter
Nostalgic filter can add an old-fashioned feel to the picture, making it look like an old photo. Use the imagefilter() function and pass in the parameters IMG_FILTER_GRAYSCALE and IMG_FILTER_COLORIZE to achieve this.
imagefilter($sourceImage, IMG_FILTER_GRAYSCALE); imagefilter($sourceImage, IMG_FILTER_COLORIZE, 100, 50, 0);
- Output the processed image
After completing the application of the filter effect, we need to output the processed image. You can use the imagejpeg() function to save the target image in .jpeg format and specify the save path.
imagejpeg($targetImage, "path/to/target/image.jpg");
- Complete code example
$sourceImage = imagecreatefromjpeg("path/to/source/image.jpg"); $targetImage = imagecreatetruecolor(imagesx($sourceImage), imagesy($sourceImage)); $backgroundColor = imagecolorallocate($targetImage, 255, 255, 255); imagefill($targetImage, 0, 0, $backgroundColor); imagecopy($targetImage, $sourceImage, 0, 0, 0, 0, imagesx($sourceImage), imagesy($sourceImage)); // 添加滤镜效果 imagefilter($sourceImage, IMG_FILTER_GRAYSCALE); // 或者:imagefilter($sourceImage, IMG_FILTER_NEGATE); // 或者:imagefilter($sourceImage, IMG_FILTER_GRAYSCALE); // imagefilter($sourceImage, IMG_FILTER_COLORIZE, 100, 50, 0); // 输出处理后的图片 imagejpeg($targetImage, "path/to/target/image.jpg"); // 释放图片资源 imagedestroy($sourceImage); imagedestroy($targetImage);
Summary: Through the above steps, we can use PHP to develop a simple picture filter function. In actual development, you can adjust filter effects or add more filter effects according to different needs. Using PHP and the GD library, you can easily perform various processing on images.
The above is the detailed content of How to use PHP to develop a simple picture filter function. For more information, please follow other related articles on the PHP Chinese website!
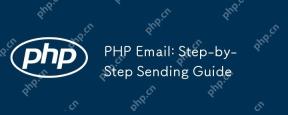
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
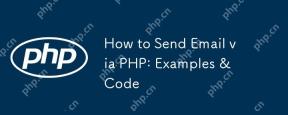
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
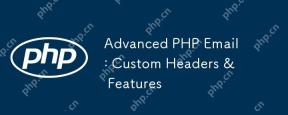
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
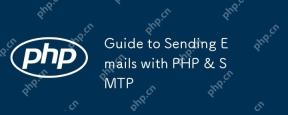
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
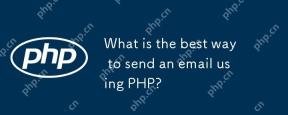
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
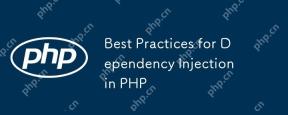
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
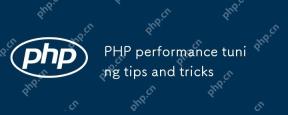
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
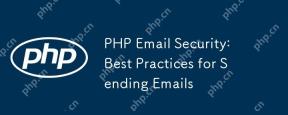
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
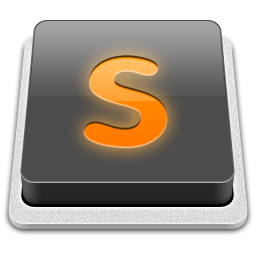
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version
