


What are the optimization strategies and implementation methods of the Hill sorting algorithm in PHP?
Hill sorting is an efficient sorting algorithm. It divides the array to be sorted into several sub-arrays by defining an increment sequence, performs insertion sorting on these sub-arrays, and then gradually reduces the increment Until the increment reaches 1, a final insertion sort is performed to complete the entire sorting process. Compared with traditional insertion sort, Hill sort can turn the array to be sorted into partially ordered faster, thereby reducing the number of comparisons and exchanges.
The optimization strategy of Hill sorting is mainly reflected in two aspects: defining incremental sequences and using insertion sorting.
- Define incremental sequence
The choice of incremental sequence has a great impact on the efficiency of Hill sorting. Common incremental sequences include Hill incremental sequence, Hibbard incremental sequence, Sedgewick incremental sequence, etc. Among them, the Hill increment sequence is the simplest, and its definition is as follows: h = h * 3 1, where h is the increment and the initial value is 1. Hibbard incremental sequence and Sedgewick incremental sequence are more complicated, and their definitions and calculation methods can be found online with specific formulas. Choosing an appropriate increment sequence can reduce the time complexity of sorting. - Use insertion sort
In each round of Hill sorting, insert the subarray to be sorted into sorting. The reason for using insertion sort is that when the increment is large, inserting the subarray into an insertion sort can move smaller elements to the appropriate location faster, thus improving performance. In practical applications, you can choose a version of insertion sort based on data volume and performance requirements, such as direct insertion sort, binary insertion sort, etc. In addition, different insertion sort algorithms can be used for each group based on the number of sorted groups and the characteristics of Hill sort.
The following is a PHP code example that demonstrates how to sort using Hill sort:
function shellSort(&$arr) { $len = count($arr); // 定义增量序列 $h = 1; while ($h < intval($len / 3)) { $h = $h * 3 + 1; } while ($h >= 1) { // 子数组进行插入排序 for ($i = $h; $i < $len; $i++) { $temp = $arr[$i]; $j = $i - $h; while ($j >= 0 && $arr[$j] > $temp) { $arr[$j + $h] = $arr[$j]; $j -= $h; } $arr[$j + $h] = $temp; } // 减小增量 $h = intval($h / 3); } } // 测试代码 $arr = [9, 5, 2, 7, 1, 8, 6, 4, 3]; shellSort($arr); print_r($arr);
The above code example demonstrates how to sort an integer array using the Hill sort algorithm. First define the increment sequence, then control the size of the increment through a loop and call the insertion sort algorithm to sort the subarray. The final output is the sorted result.
Hill sorting algorithm can move smaller elements to the appropriate position faster when the increment is large through appropriate increment sequences and the use of insertion sorting algorithm, thereby improving sorting efficiency. In practical applications, the appropriate incremental sequence and insertion sort algorithm can be selected according to the specific problem and the size of the data to achieve the best sorting effect.
The above is the detailed content of What are the optimization strategies and implementation methods of Hill sorting algorithm in PHP?. For more information, please follow other related articles on the PHP Chinese website!
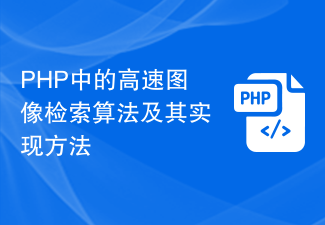
PHP中的高速图像检索算法及其实现方法随着数字图像的广泛应用,图像检索技术也越来越受到关注。高速图像检索算法是图像检索中的一种重要方法,它可以在海量图像数据中快速找到与查询图像相似的图像。本文将介绍PHP中的高速图像检索算法及其实现方法。一、高速图像检索算法的原理高速图像检索算法的核心思想是将图像转换为特征向量,然后计算特征向量之间的相似度,从而找到与查询图
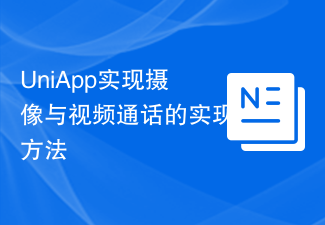
UniApp是一款基于HBuilder开发的跨平台开发框架,能够实现一份代码在多个平台上运行。本文将介绍在UniApp中如何实现摄像与视频通话的功能,并给出相应的代码示例。一、获取用户摄像头权限在UniApp中,我们需要首先获取用户的摄像头权限。在页面的mounted生命周期函数中,使用uni的authorize方法调用摄像头权限。代码示例如下:mounte
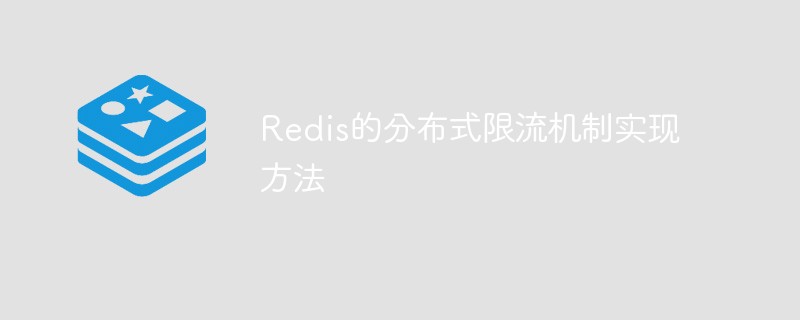
随着互联网应用的发展,高并发访问成为了互联网公司极为重要的问题。为了保证系统的稳定性,我们需要对访问进行限制,防止恶意攻击或者过度访问导致系统崩溃。限流机制被广泛应用于互联网应用中,其中Redis作为一个流行的缓存数据库,也提供了分布式限流的解决方案。Redis的限流机制主要有以下两种实现方法:1.基于令牌桶算法的限流令牌桶算法是互联网常用的限流算法之一,R
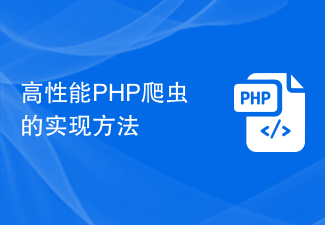
随着互联网的发展,网页中的信息量越来越大,越来越深入,很多人需要从海量的数据中快速地提取出自己需要的信息。此时,爬虫就成了重要的工具之一。本文将介绍如何使用PHP编写高性能的爬虫,以便快速准确地从网络中获取所需的信息。一、了解爬虫基本原理爬虫的基本功能就是模拟浏览器去访问网页,并获取其中的特定信息。它可以模拟用户在网页浏览器中的一系列操作,比如向服务器发送请
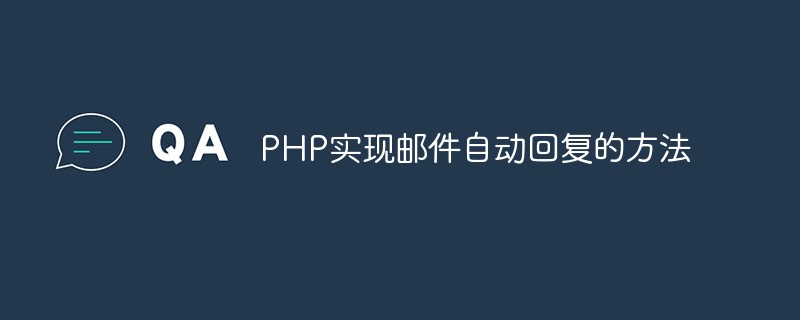
PHP是一种流行的服务器端脚本语言,它可以用于实现各种不同类型的应用程序,其中包括邮件自动回复。邮件自动回复是一种非常有用的功能,可以用于自动回复一系列电子邮件,从而节省时间和精力。在本文中,我将介绍如何使用PHP实现邮件自动回复。第一步:安装PHP和web服务器在开始实现邮件自动回复之前,必须先安装PHP和web服务器。对于大多数人来说,Apache是最常
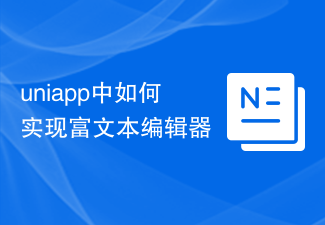
uniapp中如何实现富文本编辑器在许多应用程序中,我们经常遇到需要用户输入富文本内容的情况,比如编辑文章、发布动态等。为了满足这个需求,我们可以使用富文本编辑器来实现。在uniapp中,我们可以使用一些开源的富文本编辑器组件,比如wangeditor、quill等。下面,我将以wangeditor为例,介绍在uniapp中如何实现富文本编
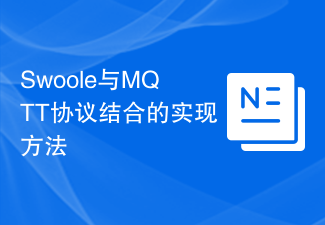
随着物联网的发展,越来越多的应用程序需要实时地进行数据传输和通信。消息队列传输协议(MQTT)是一种轻量级的协议,适用于小型设备和低带宽环境下,常被用于物联网设备数据传输。Swoole作为一种高性能、异步、事件驱动的网络通信框架,提供了高效的TCP/UDP/UnixSocket协议的实现,可以和MQTT协议结合使用,提供更加高效的系统通信。本文将会介绍如何使
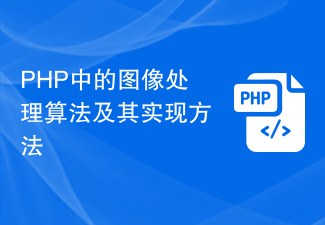
在Web开发中,图像处理是一个十分重要的话题。而PHP作为一个功能强大的服务器端脚本语言,自然也有着充分的图像处理能力。本文将介绍PHP中常用的图像处理算法以及如何实现这些算法。一、PHP中的图像处理函数在PHP中,处理图像的函数是位于GD库(GraphicsDraw)中的。这些函数提供了许多用于处理图像的功能,包括裁剪、缩放、旋转、滤镜、水印等。下面是几


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
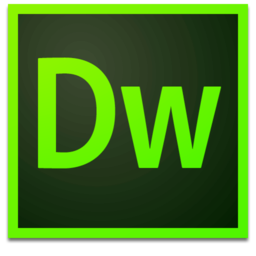
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
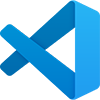
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
