


Implement dynamic programming logic using PHP closures, generators, and reflection technology
Use PHP closures, generators and reflection technology to implement dynamic programming logic
Overview:
In software development, dynamic programming logic is a very useful A technology that changes the behavior of a program at runtime based on conditions or user input. PHP is a powerful programming language that provides functions such as closures, generators, and reflection to implement dynamic programming logic. This article will introduce how to use these technologies to implement dynamic programming logic and give specific code examples.
- Use of closure:
A closure is a special object that can pass, store and call functions as variables. Closures can take external variables and use these variables to change the behavior of the function. By using closures, we can implement dynamic programming logic.
Sample code:
$dynamicLogic = function ($input) { if ($input > 0) { echo "Input is positive."; } else { echo "Input is negative or zero."; } }; $input = -5; $dynamicLogic($input);
In the above example, we defined a closure variable $dynamicLogic
, which accepts a parameter $input
. If $input
is greater than 0, output "Input is positive."; otherwise, output "Input is negative or zero.". By changing the value of $input
, we can change the behavior of the closure and implement dynamic programming logic.
- Use of Generator:
A generator is a special function in PHP that can iteratively generate a series of values. Generators produce one value at a time and then pause execution to wait for the next iteration. This feature allows us to change the behavior of the generator at each iteration and implement dynamic programming logic.
Sample code:
function dynamicGenerator() { $i = 0; while ($i < 5) { yield $i; $i++; } } $generator = dynamicGenerator(); foreach ($generator as $value) { if ($value % 2 == 0) { echo $value . " is even."; } else { echo $value . " is odd."; } }
In the above example, we have defined a generator function dynamicGenerator
which uses the yield
keyword Produces values from 0 to 4. At each iteration, we can change the behavior of the generator based on the parity of the values. In this way we can implement dynamic programming logic.
- Use of Reflection:
Reflection is a powerful mechanism provided by PHP, which can obtain detailed information about classes, methods, properties, etc. at runtime, and can dynamically Create objects, call methods, etc. By utilizing reflection, we can implement more flexible dynamic programming logic.
Sample code:
class DynamicClass { public function dynamicMethod($input) { if ($input > 0) { echo "Input is positive."; } else { echo "Input is negative or zero."; } } } $className = "DynamicClass"; $methodName = "dynamicMethod"; $input = -5; $reflectionClass = new ReflectionClass($className); $reflectionMethod = $reflectionClass->getMethod($methodName); $reflectionMethod->invoke(new $className, $input);
In the above example, we define a class DynamicClass
, which contains a method dynamicMethod
, which The method accepts one parameter $input
. Through reflection, we can obtain methods based on class names and method names, and dynamically create objects and call methods. By changing the value of $input
, we can change the behavior of the method and implement dynamic programming logic.
Summary:
Using PHP closures, generators and reflection technology, we can implement dynamic programming logic. Closures can change the behavior of functions through external variables; generators can change the behavior of generators at each iteration; reflection can obtain information about classes, methods, etc. at runtime, and dynamically create objects, call methods, etc. Through the combined application of these technologies, we can write more flexible and dynamic code.
The above is the detailed content of Implement dynamic programming logic using PHP closures, generators, and reflection technology. For more information, please follow other related articles on the PHP Chinese website!
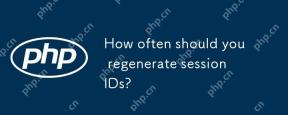
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
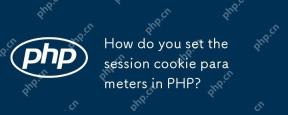
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
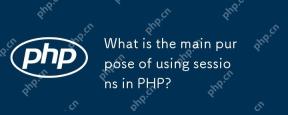
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
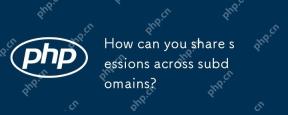
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
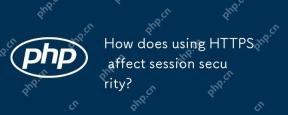
HTTPS significantly improves the security of sessions by encrypting data transmission, preventing man-in-the-middle attacks and providing authentication. 1) Encrypted data transmission: HTTPS uses SSL/TLS protocol to encrypt data to ensure that the data is not stolen or tampered during transmission. 2) Prevent man-in-the-middle attacks: Through the SSL/TLS handshake process, the client verifies the server certificate to ensure the connection legitimacy. 3) Provide authentication: HTTPS ensures that the connection is a legitimate server and protects data integrity and confidentiality.
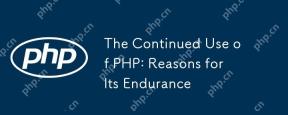
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
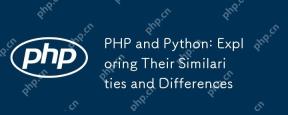
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
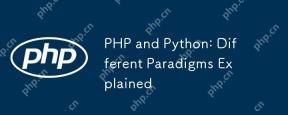
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
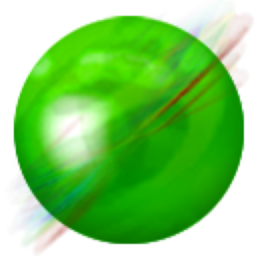
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
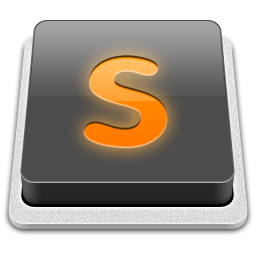
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
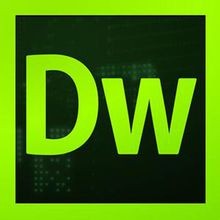
Dreamweaver CS6
Visual web development tools