


How to solve the performance bottleneck in PHP back-end function development?
How to solve the performance bottleneck in PHP back-end function development?
With the development of the Internet, PHP, as a popular back-end development language, is widely used in the development of various websites and applications. However, during the function development process of PHP backend, we often face the challenge of performance bottleneck. This article will introduce some common performance bottlenecks and provide solutions to optimize the performance of background functions.
1. Database query performance optimization
One of the most common performance bottlenecks in PHP back-end development is database query. Here are some ways to optimize database queries:
- Ensure the correct use of indexes: Indexes are one of the key factors in database query performance. Check whether the indexes on the table are used correctly and perform index optimization based on actual query requirements.
- Reduce the number of queries: Multiple queries will increase the load on the database. You can reduce the number of database queries by using JOIN statements, subqueries or reducing redundant queries.
- Appropriate use of cache: For some data that does not change frequently, cache can be used to reduce the number of database queries. For example, use in-memory databases such as Redis to cache data and reduce IO operations on the disk.
2. Code logic optimization
In addition to database queries, code logic is also a common cause of performance bottlenecks. The following are some ways to optimize code logic:
- Reduce loop nesting: Avoid performing a large number of complex operations or nested loops in loops. You can try to use more efficient algorithms or data structures to reduce the number of loops.
- Merge duplicate code: Identical code blocks can be merged into a function or method to reduce the number of repeated executions.
- Avoid global variables: Global variables can cause performance degradation in PHP. Try to avoid using global variables and use local variables or class attributes instead.
3. Cache usage
Cache is one of the effective means to improve back-end performance. The following are some common cache usage methods:
- Use PHP built-in cache: PHP provides some built-in caching mechanisms, such as OPcache, APCu, etc. These caching mechanisms can be enabled in the PHP configuration file to improve performance.
- Use external cache: In addition to PHP's built-in cache, you can also use some external cache systems, such as Memcached, Redis, etc. Cache some popular data or commonly used calculation results to reduce repeated execution of the same calculations.
4. Server Optimization
Server optimization is also the key to improving PHP back-end performance. The following are some server optimization methods:
- Adjust server configuration: According to specific application requirements, appropriately adjust the server configuration, such as increasing memory, adjusting the processor, etc.
- Use a high-performance server: Choose a high-performance server, such as Nginx or Apache, to improve system performance.
5. Concurrent processing
In a high-concurrency environment, the ability to process requests becomes a performance bottleneck. The following are some methods of concurrent processing:
- Use asynchronous mechanism: Using asynchronous mechanism can improve the ability of concurrent processing. For example, use Swoole extension for asynchronous network communication.
- Use message queue: Put the request task into the message queue and let the backend process the requests one by one to reduce the pressure of processing requests at the same time.
To sum up, by optimizing database query, code logic, cache usage, server configuration and concurrent processing, the performance bottleneck in PHP back-end function development can be effectively solved. Select appropriate optimization methods based on specific problems and needs, and perform testing and adjustments to improve the performance and user experience of backend functions.
The following is a code example for optimizing database queries:
// 使用索引优化查询 $sql = "SELECT * FROM users WHERE age > 18"; $index = "idx_age"; $result = $db->query("SELECT * FROM users USE INDEX ($index) WHERE age > 18"); // 减少查询次数 $ids = [1, 2, 3]; $data = []; foreach ($ids as $id) { $result = $db->query("SELECT * FROM users WHERE id = :id", [":id" => $id]); $data[$id] = $result; } // 使用缓存 $cacheKey = "user:{$id}"; if (!$data = $cache->get($cacheKey)) { $result = $db->query("SELECT * FROM users WHERE id = :id", [":id" => $id]); $cache->set($cacheKey, $result, 3600); $data = $result; }
This code example shows how to use indexes to optimize queries, reduce the number of queries, and use caching to optimize the performance of database queries. Depending on the specific situation, corresponding optimization can be carried out based on actual needs and database structure.
The above is the detailed content of How to solve the performance bottleneck in PHP back-end function development?. For more information, please follow other related articles on the PHP Chinese website!
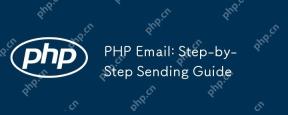
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
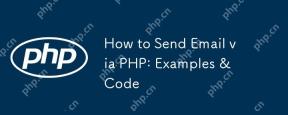
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
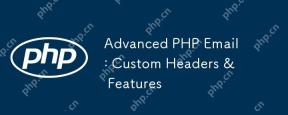
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
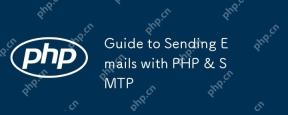
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
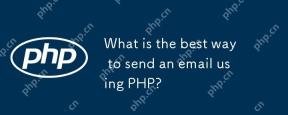
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
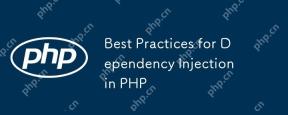
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
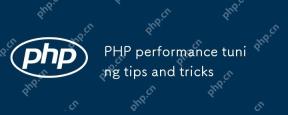
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
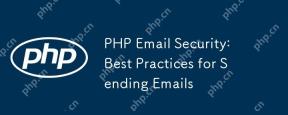
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
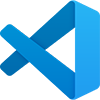
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
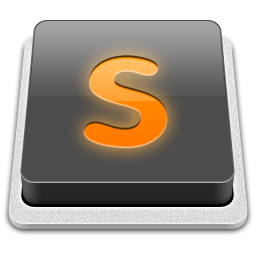
SublimeText3 Mac version
God-level code editing software (SublimeText3)
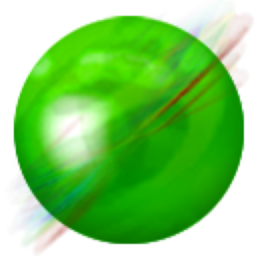
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
