


MQTT subscription and publishing load balancing optimization method in PHP development
MQTT (Message Queuing Telemetry Transport) is a lightweight message transmission protocol that is widely used in fields such as the Internet of Things and instant messaging. When using MQTT to subscribe and publish messages in PHP development, load balancing optimization is an important issue. This article will introduce an MQTT load balancing optimization method based on Redis and give corresponding code examples.
The goal of MQTT load balancing optimization is to improve the scalability and fault tolerance of the system and minimize the impact of single points of failure. In the traditional MQTT architecture, the message publisher sends the message to an MQTT Broker, and then the subscriber gets the message from the MQTT Broker. This architecture runs the risk of a single point of failure because if the MQTT Broker fails, the entire system will not work properly. Therefore, we need to introduce load balancing and distribute message publishers and subscribers to multiple Brokers to achieve high availability and fault tolerance.
In this load balancing architecture, we need to use Redis to register the publication and subscription of messages. First, we create a Redis instance to store the publisher information and subscriber information of the message. When the message publisher sends a message, it first sends the message to Redis, and then Redis forwards the message to the subscriber. Subscribers first register their subscription information with Redis, and then obtain subscribed messages from Redis.
The following is a code example of MQTT load balancing optimization using Redis:
<?php // 发布消息 function publishMessage($topic, $message) { $redis = new Redis(); $redis->connect('127.0.0.1', 6379); $redis->publish($topic, $message); } // 订阅消息 function subscribeMessage($topic) { $redis = new Redis(); $redis->connect('127.0.0.1', 6379); $redis->subscribe([$topic], 'processMessage'); } // 处理消息 function processMessage($redis, $topic, $message) { echo "Received message: $message "; } // 发布消息 publishMessage('topic1', 'Hello World!'); // 订阅消息 subscribeMessage('topic1');
In the above code example, we used the publish
method of Redis to implement it For message publishing, Redis's subscribe
method is used to implement message subscription. processMessage
function is used to process received messages.
Through this Redis-based load balancing optimization method, we can disperse message publishers and subscribers to multiple nodes to improve the scalability and fault tolerance of the system. At the same time, due to the high performance and high availability of Redis, reliable transmission of messages can be guaranteed.
To summarize, this article introduces an MQTT load balancing optimization method based on Redis. Using this method in PHP development can achieve high availability and fault tolerance of messages. Through the demonstration of sample code, readers can understand and master how to use Redis to publish and subscribe to messages, and understand how to use Redis to implement MQTT load balancing. I hope this article has provided some help to readers in using MQTT to optimize subscription and publishing load balancing in PHP development.
The above is the detailed content of MQTT subscription and publishing load balancing optimization method in PHP development. For more information, please follow other related articles on the PHP Chinese website!
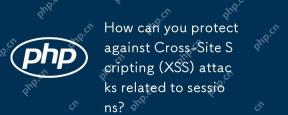
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
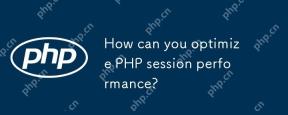
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
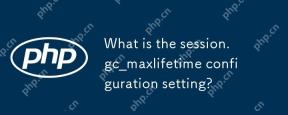
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
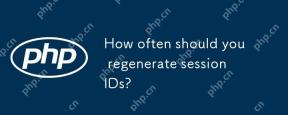
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
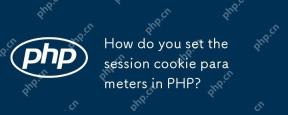
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
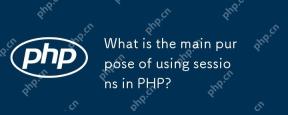
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
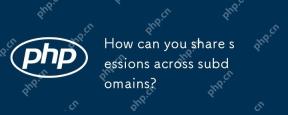
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
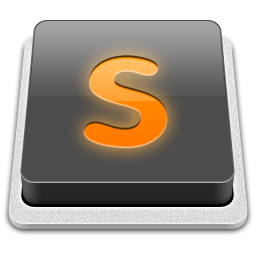
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
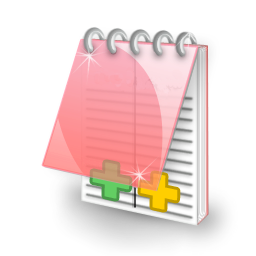
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.