PHP is a programming language widely used in web development. It provides many features and tools that can be used to implement URL redirection and routing. This article will introduce how to use PHP to implement these functions and help readers better understand and apply PHP.
URL redirection refers to redirecting users from one URL address to another URL address. In web development, URL redirection is widely used in scenarios such as web page jumps, page redirection processing, and error handling. In PHP, you can use the header function to implement URL redirection.
The header function is a built-in function of PHP, used to send original HTTP header information to the client. To implement URL redirection, just call the header function in the PHP code and set the Location header information to the URL address to be redirected. The following is a simple sample code:
header("Location: http://example.com");
In the above code, when the PHP script is executed to this line, a Location header information will be sent to the browser, telling the browser to redirect the page to http: //example.com.
It should be noted that there cannot be any output before calling the header function, including HTML tags and spaces. Because the header function must be called before the HTTP header information is sent to the client, otherwise an error will occur.
In addition to using the header function to implement basic URL redirection, you can also use more advanced methods, such as redirecting to relative paths, using 301 permanent redirects, and passing parameters. These usages can be expanded and applied based on specific needs and PHP programming experience.
In web development, routing refers to distributing requests through URLs to different processing logic or controllers. Routing plays a very important role in web applications. It can map URLs to specified processing logic, thereby realizing the distribution and processing of requests.
In PHP, routing can be implemented by using a framework or by coding manually. If you use a framework, it will generally provide relevant routing functions and routing configurations, and developers only need to configure them according to the prescribed methods and rules. Common PHP frameworks such as Laravel, Symfony and Zend Framework all provide powerful routing functions.
If you are not using a framework, you can manually implement routing by parsing the URL and processing the request parameters. The following is a simple sample code:
$url = $_SERVER['REQUEST_URI']; $route = [ '/' => 'HomeController@index', '/about' => 'HomeController@about', '/contact' => 'ContactController@index', '/product/(d+)' => 'ProductController@show', ]; foreach ($route as $pattern => $handler) { if (preg_match('~^' . $pattern . '$~', $url, $matches)) { $parts = explode('@', $handler); $controller = $parts[0]; $method = $parts[1]; // 调用对应的控制器和方法 call_user_func_array([$controller, $method], array_slice($matches, 1)); break; } }
In the above code, the $route variable defines the corresponding relationship between URL and processing logic, in which some URLs with parameters are defined in regular expressions. By traversing the $route array, parsing the URL and matching the corresponding processing logic, the corresponding controller and method are called.
It should be noted that the above code is just a simplified version of routing implementation, and actual applications may be more complex, including error handling, parameter filtering, etc. In addition, in order to improve the readability and maintainability of the code, you can consider separating the definition and execution logic of routes into different files or classes.
To sum up, PHP provides a wealth of functions and tools that can be used to implement URL redirection and routing. This article introduces the method of using the header function to implement URL redirection and using regular expressions to manually implement routing, helping readers better understand and apply the URL redirection and routing functions in PHP.
The above is the detailed content of How to implement URL redirection and routing in PHP?. For more information, please follow other related articles on the PHP Chinese website!
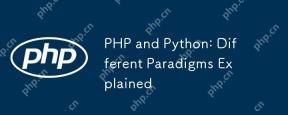
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
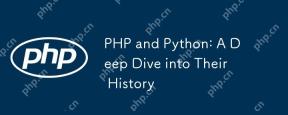
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
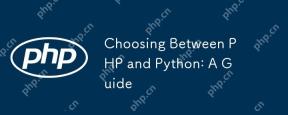
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
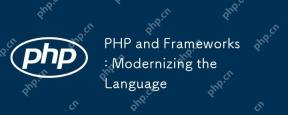
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
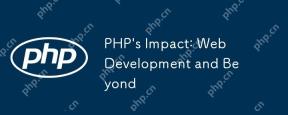
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
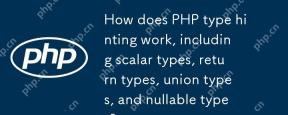
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
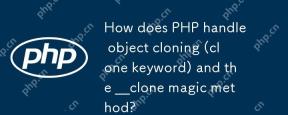
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
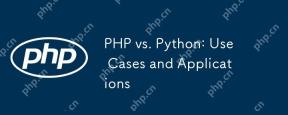
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
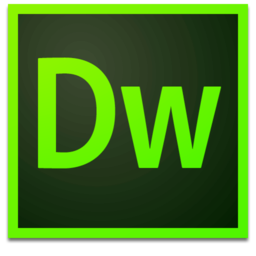
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
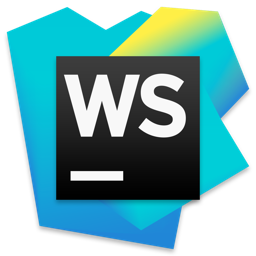
WebStorm Mac version
Useful JavaScript development tools