


Go language, as a high-performance, concurrency-friendly programming language, is widely used in various large-scale file processing tasks. This article will introduce how to deal with large-scale file processing problems in Go language development from the aspects of file reading, file writing, and concurrent processing.
1. File reading
When processing large-scale files, you first need to consider how to read the file content efficiently. The Go language provides a variety of ways to read files, the most commonly used of which is to use the Scanner type in the bufio package. The Scanner type can easily read file contents line by line and provides many convenient methods for processing the read data.
The following is a simple sample code that demonstrates how to use Scanner to read the file content line by line and output the content of each line:
package main import ( "bufio" "fmt" "os" ) func main() { file, err := os.Open("input.txt") if err != nil { fmt.Println("打开文件失败:", err) return } defer file.Close() scanner := bufio.NewScanner(file) for scanner.Scan() { line := scanner.Text() fmt.Println(line) } if err := scanner.Err(); err != nil { fmt.Println("文件读取错误:", err) } }
Through the above code, we can quickly read large files scale the contents of the file and process it further.
2. File writing
During the file processing process, sometimes it is necessary to write the processing results to a file. The Go language provides the File type in the os package, which can directly create or open a file and perform writing operations.
The following is a simple sample code that demonstrates how to write the processing results to a file:
package main import ( "fmt" "os" ) func main() { file, err := os.Create("output.txt") if err != nil { fmt.Println("创建文件失败:", err) return } defer file.Close() content := "Hello, world!" _, err = file.WriteString(content) if err != nil { fmt.Println("写入文件失败:", err) return } fmt.Println("写入文件成功") }
With the above code, we can write the processing results to a file and write the console output Enter successful information.
3. Concurrent processing
When processing large-scale files, in order to improve efficiency, we often use concurrency for file processing. The Go language inherently supports concurrency, so it can easily implement the task of processing large-scale files concurrently.
The following is a simple sample code that demonstrates how to use goroutine for concurrent processing in the Go language:
package main import ( "bufio" "fmt" "os" "sync" ) func processLine(line string, wg *sync.WaitGroup) { defer wg.Done() // 在这里处理每一行的逻辑 fmt.Println(line) } func main() { file, err := os.Open("input.txt") if err != nil { fmt.Println("打开文件失败:", err) return } defer file.Close() scanner := bufio.NewScanner(file) var wg sync.WaitGroup for scanner.Scan() { line := scanner.Text() wg.Add(1) go processLine(line, &wg) } wg.Wait() if err := scanner.Err(); err != nil { fmt.Println("文件读取错误:", err) } }
The processLine function in the above code is a function used to process each line, This function can be written by yourself according to actual needs. By using the WaitGroup type in the sync package, we can achieve the purpose of concurrent execution of the processLine function.
Through concurrent processing, we can greatly improve the efficiency of large-scale file processing tasks.
To sum up, the Go language provides a series of efficient file processing methods, which can well meet the needs of large-scale file processing. By rationally using technologies such as file reading, file writing, and concurrent processing, we can efficiently process large-scale file data. I believe that with the continuous development of the Go language, the file processing capabilities will become more powerful and more suitable for various scenarios.
The above is the detailed content of How to deal with large-scale file processing problems in Go language development. For more information, please follow other related articles on the PHP Chinese website!
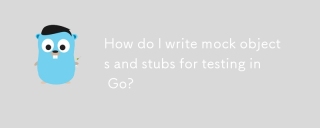
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
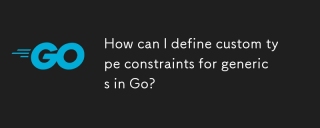
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
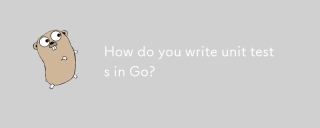
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
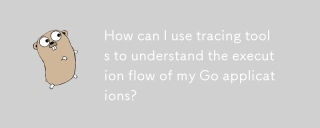
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
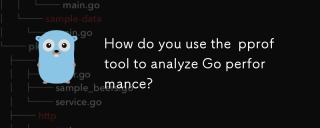
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
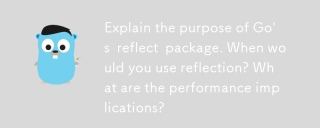
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
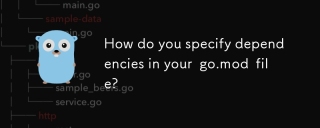
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
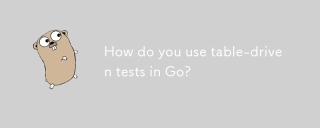
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
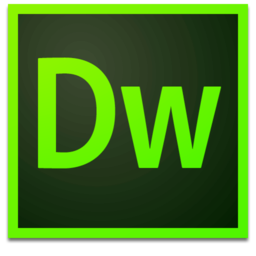
Dreamweaver Mac version
Visual web development tools
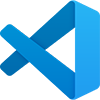
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
