Custom interfaces in Go are crucial for writing flexible, maintainable, and testable code. They enable developers to focus on behavior over implementation, enhancing modularity and robustness. By defining method signatures that types must implement, interfaces allow for code reusability and abstraction, making it easier to switch between different implementations like local file systems or cloud storage without altering core functions.
When diving into the world of Go, or Golang, custom interfaces are a cornerstone for creating flexible and maintainable code. They allow developers to define a contract that can be implemented by various types, enhancing code reusability and promoting abstraction. So, why should you care about custom interfaces in Go? Well, they're your key to writing cleaner, more modular code that's easier to test and maintain. Plus, they open up a world of possibilities for designing your applications with a focus on behavior rather than specific implementations.
Let's dive right into the world of custom interfaces in Go and see how they can transform your coding approach.
Custom interfaces in Go are essentially a way to define a set of method signatures that a type must implement. This concept might sound simple, but it's incredibly powerful. Imagine you're building a system where you need to handle different types of data storage—say, a local file system, a cloud storage service, or a database. By defining an interface for these storage mechanisms, you can write code that interacts with any storage type without knowing the specifics of how each one works.
Here's a simple yet elegant example of defining a custom interface in Go:
type Storage interface { Save(data string) error Load() (string, error) }
This Storage
interface declares two methods: Save
and Load
. Any type that implements both of these methods satisfies the Storage
interface. Let's see how you might implement this interface with a local file system:
type FileSystem struct { path string } func (fs *FileSystem) Save(data string) error { // Implementation to save data to a file return nil } func (fs *FileSystem) Load() (string, error) { // Implementation to load data from a file return "", nil }
And now, you can use this FileSystem
type wherever a Storage
is expected:
func ProcessData(storage Storage) { err := storage.Save("Hello, Go!") if err != nil { log.Fatal(err) } data, err := storage.Load() if err != nil { log.Fatal(err) } fmt.Println(data) } func main() { fs := &FileSystem{path: "/tmp/data.txt"} ProcessData(fs) }
This approach allows you to switch out the FileSystem
for any other type that implements the Storage
interface—say, a CloudStorage
or DatabaseStorage
—without changing the ProcessData
function.
Now, let's talk about some nuances and best practices when working with custom interfaces in Go.
One thing to keep in mind is that Go's interfaces are implicitly satisfied. This means you don't need to explicitly declare that a type implements an interface; if it has all the required methods, it automatically satisfies the interface. This feature is both a blessing and a curse. It's great for flexibility and ease of use, but it can also lead to runtime errors if you're not careful. Always ensure that your types fully implement the interfaces you expect them to.
Another aspect to consider is the granularity of your interfaces. It's tempting to define large, comprehensive interfaces, but smaller, more focused interfaces often lead to more flexible and maintainable code. For instance, instead of a single Storage
interface, you might define Saver
and Loader
interfaces:
type Saver interface { Save(data string) error } type Loader interface { Load() (string, error) }
This approach allows you to mix and match implementations more easily and can lead to more testable code.
When it comes to testing, custom interfaces are your best friend. By defining interfaces for the dependencies of your code, you can easily mock out these dependencies in your tests. For example, if you're testing a function that uses a Storage
interface, you can create a mock implementation for your tests:
type MockStorage struct { savedData string } func (ms *MockStorage) Save(data string) error { ms.savedData = data return nil } func (ms *MockStorage) Load() (string, error) { return ms.savedData, nil }
This MockStorage
can be used in your tests to verify that the function behaves correctly without actually interacting with real storage.
In terms of performance, using interfaces in Go doesn't typically introduce significant overhead. However, be mindful of the fact that interfaces are implemented using a technique called "fat pointers" under the hood, which means they carry some additional metadata. In most cases, this won't be a bottleneck, but it's worth considering if you're working in a performance-critical environment.
One of the pitfalls to watch out for when using custom interfaces is overuse. It's easy to get carried away and define an interface for every little thing, but this can lead to overly complex code. Use interfaces where they add value—typically at the boundaries of your system or where you need to decouple components.
Finally, let's touch on some advanced uses of interfaces in Go. One powerful technique is the use of interface embedding, which allows you to compose interfaces from other interfaces. This can lead to very clean and expressive code. For example:
type Reader interface { Read(p []byte) (n int, err error) } type Writer interface { Write(p []byte) (n int, err error) } type ReadWriter interface { Reader Writer }
This ReadWriter
interface is satisfied by any type that implements both Reader
and Writer
, allowing you to write code that works with types that support both operations.
In conclusion, custom interfaces in Go are a fundamental tool for writing flexible, maintainable, and testable code. They allow you to focus on behavior rather than implementation details, which is a key principle of good software design. By understanding and applying the concepts and best practices outlined here, you'll be well on your way to leveraging the full power of Go's interface system in your projects. Remember, the key is to use interfaces thoughtfully and strategically to enhance your code's modularity and robustness.
The above is the detailed content of Defining and Using Custom Interfaces in Go. For more information, please follow other related articles on the PHP Chinese website!
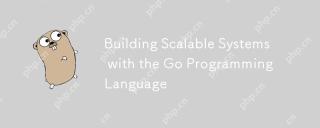
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
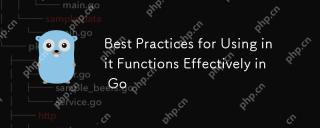
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
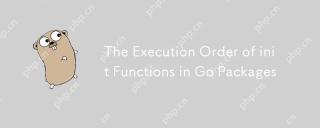
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations
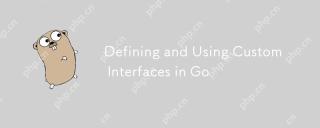
CustominterfacesinGoarecrucialforwritingflexible,maintainable,andtestablecode.Theyenabledeveloperstofocusonbehavioroverimplementation,enhancingmodularityandrobustness.Bydefiningmethodsignaturesthattypesmustimplement,interfacesallowforcodereusabilitya
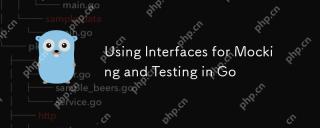
The reason for using interfaces for simulation and testing is that the interface allows the definition of contracts without specifying implementations, making the tests more isolated and easy to maintain. 1) Implicit implementation of the interface makes it simple to create mock objects, which can replace real implementations in testing. 2) Using interfaces can easily replace the real implementation of the service in unit tests, reducing test complexity and time. 3) The flexibility provided by the interface allows for changes in simulated behavior for different test cases. 4) Interfaces help design testable code from the beginning, improving the modularity and maintainability of the code.
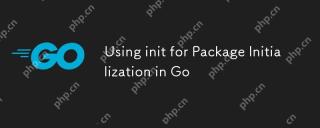
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
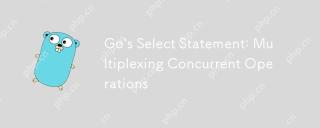
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
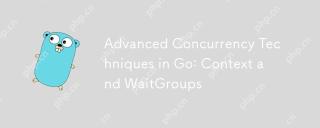
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
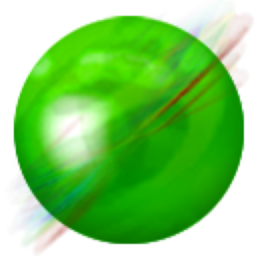
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
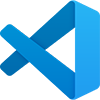
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
