Go's Select Statement: Multiplexing Concurrent Operations
Go's select statement streamlines concurrent programming by multiplexing operations. 1) It allows waiting on multiple channel operations, executing the first ready one. 2) The default case prevents deadlocks by allowing the program to proceed if no operation is ready. 3) It can be used for sending operations for load balancing. 4) Understanding its overhead and potential race conditions is crucial for optimization and synchronization.
Go's Select Statement: Mastering the Art of Multiplexing Concurrent Operations
Ever wondered how Go's select
statement can streamline your concurrent programming? Let's dive into the world of multiplexing operations and see how select
can be your secret weapon in managing goroutines effectively.
In Go, concurrency isn't just a feature; it's a core philosophy. The select
statement stands out as a powerful tool for handling multiple channel operations simultaneously. It's like having a Swiss Army knife for your concurrent code, allowing you to elegantly manage different scenarios without breaking a sweat.
Let's start by exploring what select
is all about. Imagine you're juggling multiple tasks, each represented by a goroutine. These tasks might involve sending or receiving data through channels. The select
statement lets you wait on multiple channel operations and proceed with the first one that becomes ready. It's akin to being a master of ceremonies, directing the flow of your program with finesse.
Here's a simple example to illustrate the basics:
package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(2 * time.Second) ch1 <- "one" }() go func() { time.Sleep(1 * time.Second) ch2 <- "two" }() for i := 0; i < 2; i { select { case msg1 := <-ch1: fmt.Println("Received", msg1) case msg2 := <-ch2: fmt.Println("Received", msg2) } } }
In this code, we're using select
to listen to two channels. The first goroutine sends "one" after a 2-second delay, while the second sends "two" after a 1-second delay. The select
statement will pick up "two" first, demonstrating how it waits on multiple operations and executes the first one that's ready.
Now, let's delve into the intricacies of select
. It's not just about waiting; it's about managing the flow of your program. One of the key features of select
is the default
case, which allows your program to proceed if no channel operation is ready. This can be crucial for avoiding deadlocks and ensuring your program remains responsive.
Here's an example showcasing the default
case:
package main import ( "fmt" "time" ) func main() { ch := make(chan string) go func() { time.Sleep(2 * time.Second) ch <- "data" }() for { select { case msg := <-ch: fmt.Println("Received", msg) return default: fmt.Println("No data available, doing other work...") time.Sleep(500 * time.Millisecond) } } }
In this scenario, the select
statement checks for data on the channel. If no data is available, it falls back to the default
case, allowing the program to perform other tasks without getting stuck.
Using select
effectively requires understanding its nuances. For instance, the order of cases within a select
block can impact performance. Go's runtime randomly shuffles the order of cases to ensure fairness, but in high-performance scenarios, this randomness can lead to unexpected behavior. It's a double-edged sword: while it prevents starvation, it can also introduce variability in execution times.
Another aspect to consider is the use of select
with multiple send operations. This is less common but can be powerful in certain scenarios:
package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(1 * time.Second) select { case ch1 <- "one": fmt.Println("Sent to ch1") case ch2 <- "two": fmt.Println("Sent to ch2") } }() time.Sleep(2 * time.Second) fmt.Println(<-ch1) fmt.Println(<-ch2) }
Here, we're using select
to send data to one of two channels. This can be useful for load balancing or when you need to distribute work across multiple channels.
When it comes to performance optimization, it's crucial to understand the overhead of select
. While it's an elegant solution for managing concurrency, it does come with a cost. Each select
operation involves a certain amount of runtime overhead, especially when dealing with a large number of cases. In such scenarios, consider alternative approaches like using a single channel with a struct type that can represent multiple operations.
One of the common pitfalls with select
is the potential for race conditions. If you're not careful, you might end up with unexpected behavior due to concurrent access to shared resources. Always ensure that your channel operations are properly synchronized, and consider using mutexes or other synchronization primitives when necessary.
In terms of best practices, always strive for clarity in your select
statements. Use meaningful variable names and add comments to explain complex logic. This not only makes your code more maintainable but also helps other developers understand your intent.
To wrap up, Go's select
statement is a versatile tool that can significantly enhance your concurrent programming skills. By mastering its use, you can create more efficient, responsive, and scalable applications. Remember to consider the trade-offs, such as runtime overhead and potential race conditions, and always aim for clarity and maintainability in your code.
So, go forth and conquer the world of concurrency with the power of select
!
The above is the detailed content of Go's Select Statement: Multiplexing Concurrent Operations. For more information, please follow other related articles on the PHP Chinese website!
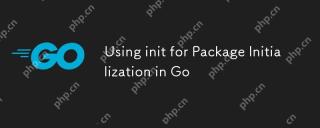
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
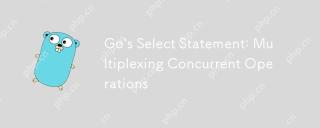
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
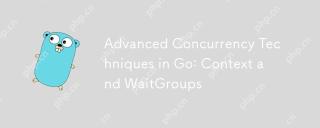
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev
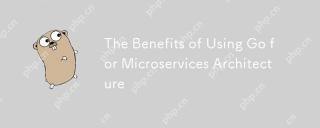
Goisbeneficialformicroservicesduetoitssimplicity,efficiency,androbustconcurrencysupport.1)Go'sdesignemphasizessimplicityandefficiency,idealformicroservices.2)Itsconcurrencymodelusinggoroutinesandchannelsallowseasyhandlingofhighconcurrency.3)Fastcompi
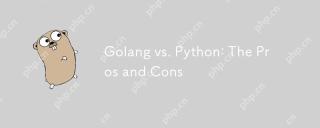
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
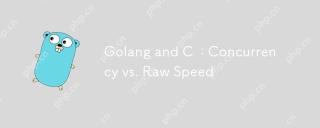
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
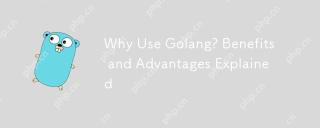
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
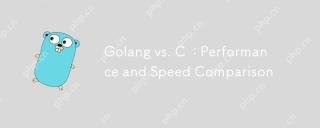
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
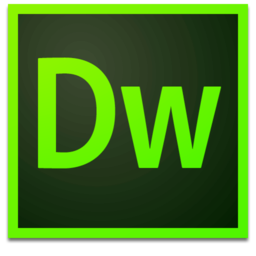
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
