Usage and examples of namespace keyword in PHP
PHP is a popular server-side scripting language that is widely used to develop web applications. In large projects, there may be a large number of code files. In order to avoid naming conflicts and improve the maintainability of the code, PHP introduces the namespace keyword.
The namespace keyword is used to define a namespace. A namespace can contain multiple classes, interfaces, functions, etc. It can help us organize and manage code and reduce conflicts in class names, function names, etc.
The syntax for using the namespace keyword is as follows:
namespace MyNamespace; // ... 此处是代码
In the above example, we created a namespace named MyNamespace.
After defining the namespace, we can define classes, interfaces, functions, etc. in the namespace. An example is as follows:
namespace MyNamespace; class MyClass { // ... 类的代码 } function myFunction() { // ... 函数的代码 }
When using classes and functions that define a namespace, we need to specify the complete namespace path. For example, if we want to use the MyClass class, we can use the following code:
$myObject = new MyNamespaceMyClass();
Similarly, if we want to use the myFunction function, we can use the following code:
MyNamespacemyFunction();
In addition, we can also use use Keywords introduce namespaces to simplify code. For example, we can use the following code:
namespace MyNamespace; use SomeNamespaceSomeClass; $someObject = new SomeClass();
In the above code, we use the use keyword to introduce the SomeNamespaceSomeClass class, and then use the SomeClass class directly without writing the complete namespace path.
Of course, if we introduce classes with the same name in multiple namespaces, we can specify aliases for them to avoid conflicts. For example:
namespace MyNamespace; use AnotherNamespaceSomeClass as AnotherClass; $anotherObject = new AnotherClass();
In addition to classes and functions, namespaces can also be used to define interfaces, constants, etc. Just use similar syntax.
In summary, the namespace keyword in PHP can help us organize and manage code, avoid naming conflicts, and improve the maintainability of the code. We can define a namespace and then define classes, functions, etc. in the namespace. When using it, you need to specify the complete namespace path, or use the use keyword to introduce the namespace to simplify the code. At the same time, we can also specify aliases for the imported namespaces to avoid conflicts.
I hope to have a better understanding of the usage and examples of the namespace keyword in PHP through this article. If you encounter naming conflicts or code management problems during development, you might as well try using the namespace keyword to organize and manage your code.
The above is the detailed content of Usage and examples of namespace keyword in PHP. For more information, please follow other related articles on the PHP Chinese website!
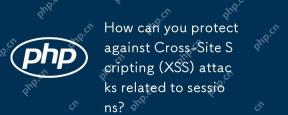
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
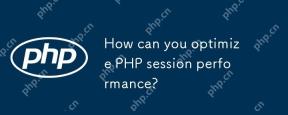
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
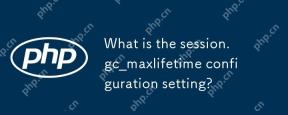
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
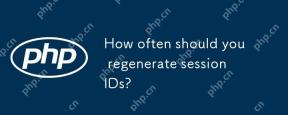
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
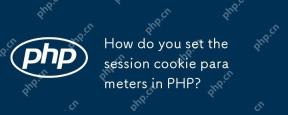
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
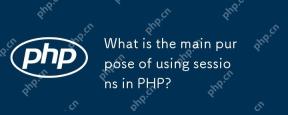
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
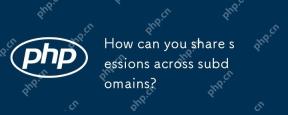
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
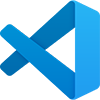
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!