Randomly sorted arrays are one of the common requirements in PHP language. For scenarios such as photo display and product display, randomly sorted arrays can enhance the interactivity and user experience of the page. Below, this article will use an example to explain how to implement random sorting of arrays through PHP functions.
We first define an array containing 5 elements, as shown below:
$products = array( "Apple", "Banana", "Orange", "Pineapple", "Watermelon" );
Next, we will use PHP’s built-in shuffle()
function to shuffle this array Sort randomly. shuffle()
The function will randomly shuffle the elements of the array to achieve a random sorting effect. The code is as follows:
shuffle($products);
Now, the $products
array has been randomly sorted, we can loop through the array through foreach
and output the value of each element. The complete code is as follows:
$products = array( "Apple", "Banana", "Orange", "Pineapple", "Watermelon" ); shuffle($products); foreach ($products as $value) { echo "$value
"; }
When we execute this code, it will output the following results:
Banana Pineapple Orange Watermelon Apple
As you can see, the elements of the array $products
are typed If the order is messed up, the output results of each execution of the code will be different. In this way, we successfully implemented the need to randomly sort the array through PHP function instances.
In addition to the shuffle()
function, PHP also provides some other functions to implement randomly sorted arrays, such as the array_rand()
function. But unlike the shuffle()
function, the array_rand()
function will only return the randomly sorted key names, but not the randomly sorted values. If you want to return a value, you also need to call $array[$key]
to get the value.
In general, it is very simple to implement randomly sorted arrays in PHP. You only need to call the built-in shuffle()
function. Through this example, it is not difficult for us to find that the role of functions in the PHP language is very important, and can help developers realize some common operation requirements more efficiently.
The above is the detailed content of PHP function example: Array random sorting. For more information, please follow other related articles on the PHP Chinese website!
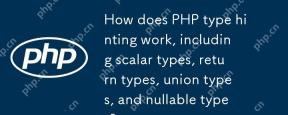
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
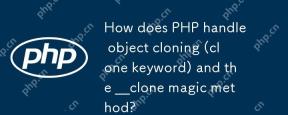
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
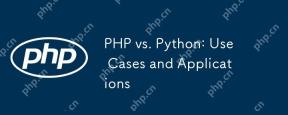
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
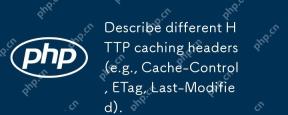
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
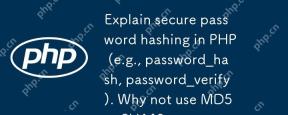
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
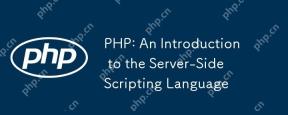
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
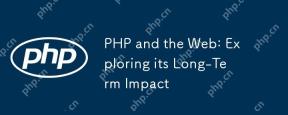
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
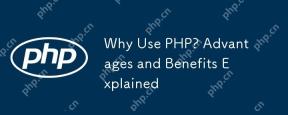
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
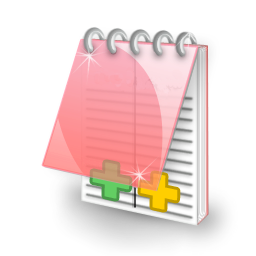
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.