Best practices for object-oriented design in PHP programs
PHP is a widely used programming language, and many websites and applications are written using PHP. With the continuous development of software development, more and more PHP programs adopt object-oriented programming ideas, which can bring better scalability, reusability and code maintenance. This article will introduce the best practices of object-oriented design in PHP programs.
- Using namespaces
Namespace is an important concept in PHP, which can help us avoid naming conflicts and improve code readability. In PHP programs, classes and interfaces should be placed in namespaces as much as possible. For example, we can put all classes in a namespace:
namespace MyClass; class MyClass1 {...} class MyClass2 {...}
- Use automatic loading
There are usually many classes and interfaces defined in PHP programs. Use automatic loading. Loading allows programs to load class files more efficiently. PHP5 introduced the __autoload function, but this function is obsolete. PHP7 recommends using the spl_autoload_register function to register autoloading functions. We can register the autoloading function in the header file:
spl_autoload_register(function ($class) { include 'classes/' . $class . '.php'; });
- Using Dependency Injection
Dependency injection is a software design pattern that helps us deal with objects dependencies. In PHP, we can use constructors to inject dependencies. For example, the following code shows how to use constructors to inject dependencies:
class A { private $b; public function __construct(B $b) { $this->b = $b; } public function doSomething() { $this->b->doSomethingElse(); } } class B { public function doSomethingElse() { // ... } } $b = new B(); $a = new A($b); $a->doSomething();
- Using abstract classes and interfaces
Abstract classes and interfaces are important in object-oriented programming Concepts that help us organize our code better. An interface defines a set of methods, and an abstract class can provide default implementations of some methods. In PHP, we can use the keywords interface and abstract to define interfaces and abstract classes. For example:
interface MyInterface { public function doSomething(); } abstract class MyAbstractClass { public function doSomething() { // ... } abstract public function doSomethingElse(); }
- Using the command pattern
The command pattern is an object-oriented design pattern that helps us decouple requests and receivers. In PHP, we can use the command pattern to achieve separation between the request and the receiver. For example:
interface Command { public function execute(); } class ConcreteCommand implements Command { private $receiver; public function __construct(Receiver $receiver) { $this->receiver = $receiver; } public function execute() { $this->receiver->action(); } } class Receiver { public function action() { // ... } } class Invoker { private $command; public function setCommand(Command $command) { $this->command = $command; } public function run() { $this->command->execute(); } } $receiver = new Receiver(); $command = new ConcreteCommand($receiver); $invoker = new Invoker(); $invoker->setCommand($command); $invoker->run();
- Using the singleton pattern
The singleton pattern is a design pattern that ensures that a class has only one instance and provides a global access point. In PHP, we can use static properties and methods to implement the singleton pattern. For example:
class Singleton { private static $instance; private function __construct() {} public static function getInstance() { if (!self::$instance) { self::$instance = new Singleton(); } return self::$instance; } }
- Using factory pattern
Factory pattern is a creation pattern that can help us hide the instantiation process of objects. In PHP, we can use factory classes to create objects. For example:
interface Product { public function doSomething(); } class ConcreteProduct implements Product { public function doSomething() { // ... } } class Factory { public static function createProduct() { return new ConcreteProduct(); } } $product = Factory::createProduct(); $product->doSomething();
Summary
The above seven best practices for object-oriented design in PHP programs are only part of what we use in our applications, but they can ensure that we are in object-oriented programming The correctness of the application and reducing our time waste, what we are proud of as developers is that we improve ourselves through continuous learning and continuous exploration, which is also the most basic principle of development.
The above is the detailed content of Best practices for object-oriented design in PHP programs. For more information, please follow other related articles on the PHP Chinese website!
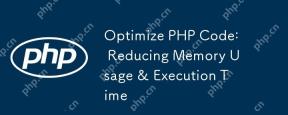
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
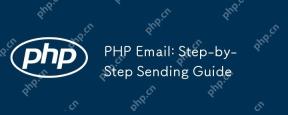
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
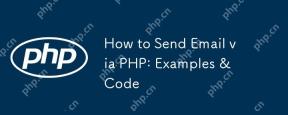
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
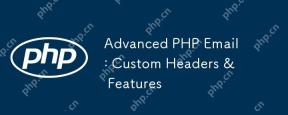
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
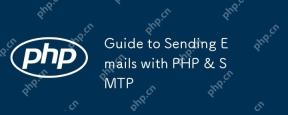
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
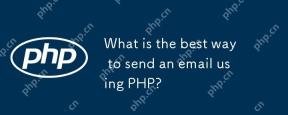
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
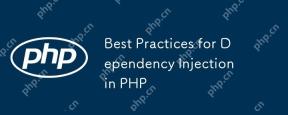
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
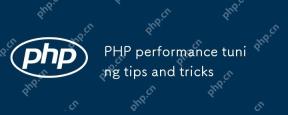
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
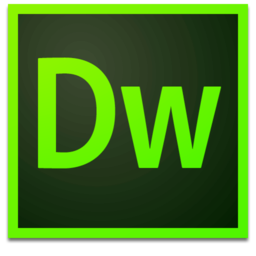
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
