Go language is a concise, easy-to-learn programming language that focuses on code robustness and error handling. In the Go language, error handling and exception mechanisms are very important parts. This article will delve into the error handling and exception mechanism in the Go language, including the basis of error handling, error types, error handling methods, as well as the exception mechanism of the Go language and how to handle exceptions.
1. The basis of error handling
In the Go language, errors are usually represented by the error type. The error type is a predefined interface type. It has an Error method that returns a string describing the error message.
In the Go language, when a function needs to return an error, it usually returns a value of type error. If there is no error, it returns nil. For example:
func f() error { // some code if errorOccurred { return errors.New("some error occurred") } // continue return nil }
In the above example, if an error occurs in the f function, a value of type error containing error information will be returned, otherwise nil will be returned.
2. Error type
The error type in Go language can be any type, but the string type is usually used to represent error information. In order to facilitate error handling, the Go language library provides an errors package for creating and processing error messages. Create an error instance containing error information through the errors.New method, for example:
func div(a, b int) (int, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil }
In the above code, the div function is used to perform integer division. If the divisor is 0, an error type containing error information is returned. value.
3. Error handling methods
There are three main error handling methods in Go language:
- Return value to capture errors
Functions and methods in the Go language usually return a value of type error to indicate whether the function or method was executed successfully. Callers can handle errors in function or method execution through error returns. For example:
result, err := someFunction() if err != nil { // handle error }
- Panic and Recover
The panic function in Go language is used to throw a runtime exception. If a panic occurs, the program will stop execution immediately, the stack frame of the current function will be popped, and the parent function that continues to execute will continue to run.
Therecover function is used to capture runtime exceptions caused by panic and allow the program to continue execution after the exception is handled. If you call recover in the defer function, you can restore the scene and handle it when an exception occurs in the program. For example:
func main() { defer func() { if r := recover(); r != nil { fmt.Println("Recovered from panic:", r) } }() panic("Panic occurred") }
In the above code, when the program executes the panic function, a runtime exception will be thrown, causing the program to stop execution and output an error message. But by calling the recover function in the main function, we can restore the scene and handle it when the program throws an exception.
- Log records errors
The log package in Go language provides a set of functions for recording information and errors. Errors can be logged to a specified file or control tower. For example:
logger := log.New(os.Stderr, "LOG: ", log.Lshortfile) logger.Println("Error message")
In the above code, we create a new logger and use the Println function to record error information. The log package provides many different methods for logging information and errors. Using the log package to log error messages is usually simpler, but not as flexible as returning error values and using the recover function.
4. Exception mechanism and processing of Go language
In Go language, exceptions are different from the exception mechanism in traditional programming languages, and do not provide syntax similar to throw and catch. However, by using statement mechanisms such as defer and recover, exception capture and processing similar to the try-catch structure can be achieved.
The defer statement is used to specify the operations that need to be performed when the function exits. Calling the defer function in a function allows the program to defer execution of certain statements until the end of the function, which makes it easy to implement operations such as resource release, exception checking, and return value calculation.
Therecover function is used to capture runtime exceptions caused by panic and return the exception that stops the program. Before calling recover, you need to use the defer function to defer the function call until after the program is executed. For example:
func panicAndRecover() { defer func() { if r := recover(); r != nil { fmt.Println("Recovered from panic:", r) } }() panic("Panic occurred") }
In the above code, we call the panic function to raise a runtime exception, and use the recover function outside it to capture the exception information. The defer statement is used to specify the operation that needs to be performed at the end of the function, that is, to handle the Panic exception. If recover is called at any time before Panic and it returns a non-nil value, execution will continue, otherwise the program will stop. If there is no recover function outside the code that can catch the exception, the program will exit.
5. Summary
This article takes the Go language as an example to introduce the implementation and processing methods of error handling and exception mechanisms. In the Go language, error handling is very important and is very convenient to implement and handle. It usually involves the creation of error information, error type definition, processing of function return values, and exception capture and handling. In addition to the above-mentioned processing methods, Go language also has other error handling methods, such as using Go language coroutines and pipelines to handle error messages. In the actual development process, it is necessary to choose an appropriate error handling method based on actual needs and business scenarios.
The above is the detailed content of Error handling and exception mechanism in Go language. For more information, please follow other related articles on the PHP Chinese website!
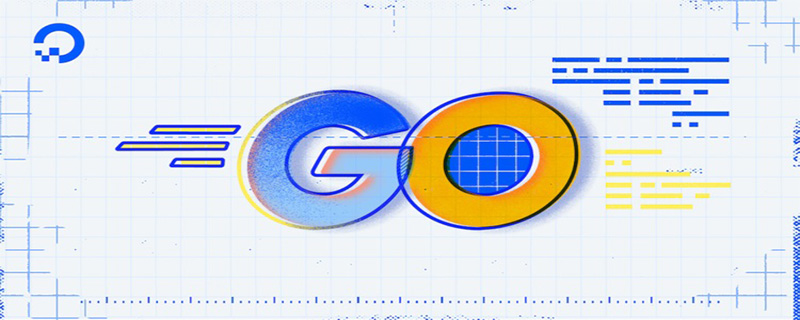
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
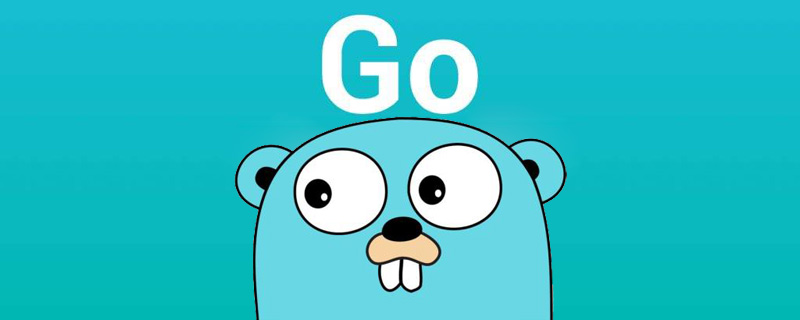
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
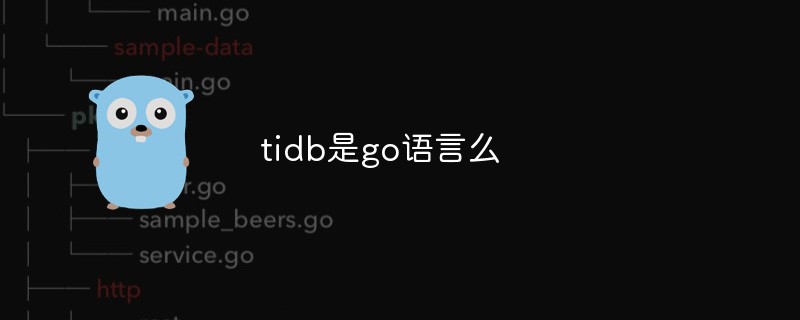
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
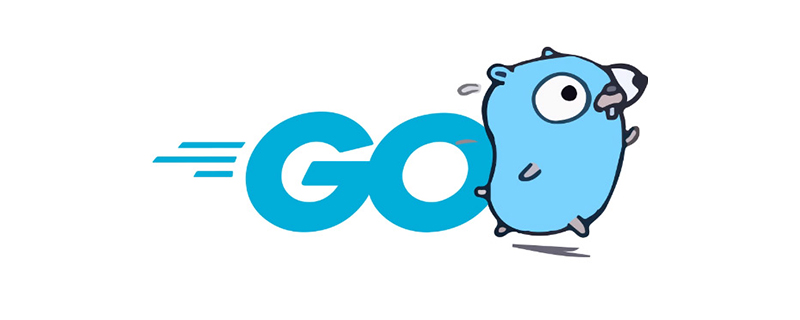
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
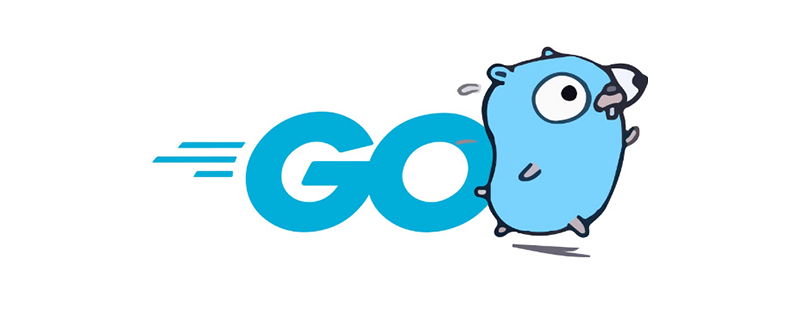
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
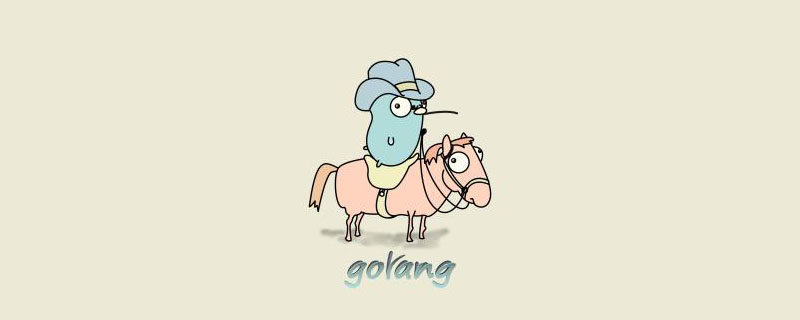
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
