


For PHP developers, operating arrays is one of the very common tasks in daily work. In many cases, we need to find a specific value in a two-dimensional array that contains many other arrays. This article will introduce readers to how to determine whether the value is in the id in a two-dimensional array in PHP.
First, let’s take a look at what a two-dimensional array is. Simply put, a two-dimensional array is an array containing multiple arrays. Each inner array has its own keys and values, usually we can use two keys: the first key is used to access the inner array in the outer array, and the second key is used to access the value in the inner array. For example:
$employees = array( array("id" => 101, "name" => "John"), array("id" => 102, "name" => "Mary"), array("id" => 103, "name" => "Peter") );
In the above example, $employees is a two-dimensional array containing three internal arrays. Each internal array has two keys (id and name) with values 101/John, 102/Mary, and 103/Peter.
Now, suppose we want to find whether a specific id value exists in the above array, we can use the foreach loop in PHP to find it. Here is the code example:
$found = false; $search_id = 102; foreach ($employees as $employee) { if ($employee['id'] === $search_id) { $found = true; break; } } if ($found) { echo "The ID is in the array"; } else { echo "The ID isn't in the array"; }
In this example, we first define a variable $found and initialize it to false. We then define a variable $search_id and assign it the specific id value we are looking for. Next, we use a foreach loop to loop through the $employees array and use an if statement to check if the id key of each inner array is equal to $search_id. If a match is found, we set the $found variable to true and use the break statement to exit the loop. Finally, we check the value of the $found variable to determine if the specific id value is in the array.
Although this method is relatively simple, it may have some performance issues with large two-dimensional arrays. Especially if lookups are required frequently, doing a full array traverse every time can degrade the performance of your application. To avoid this problem, we can use array_column function and in_array function in PHP.
The array_column function is a very useful PHP function that returns a new array from the input array containing the value of the specified key. So, if we want to extract the id value from the $employees array above, we can use the following code:
$ids = array_column($employees, 'id');
In the above example, we are passing the $employees array as the first parameter to the array_column function and Pass the id as the second parameter. It will return a new array containing all the id values in the $employees internal array.
Next, we can use the in_array function to determine if the specific value we are looking for is in the $ids array. Here is the code example:
$search_id = 102; if (in_array($search_id, $ids)) { echo "The ID is in the array"; } else { echo "The ID isn't in the array"; }
In this example, we first define a variable $search_id and assign it the value of the specific id we want to find. We then use the in_array function to check if $search_id exists in the $ids array. If a match is found, the message "The ID is in the array" is output; otherwise, the message "The ID isn't in the array" is output.
To summarize, finding a specific value in a two-dimensional array is a very common task for PHP developers. This article describes two methods: using a foreach loop for full array traversal and using the array_column function and the in_array function. Whichever method you use, you should ensure the performance and readability of your program.
The above is the detailed content of PHP determines whether the value is in the id in the two-dimensional array. For more information, please follow other related articles on the PHP Chinese website!
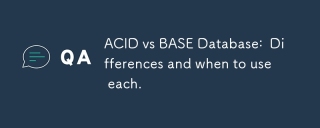
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
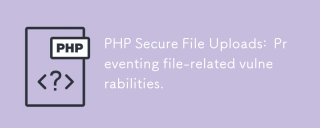
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
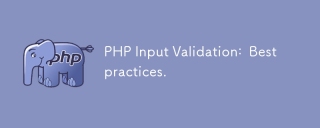
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
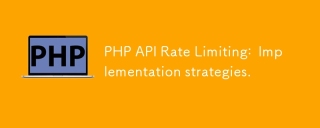
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
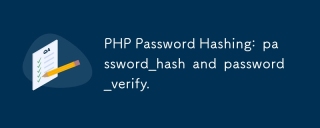
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
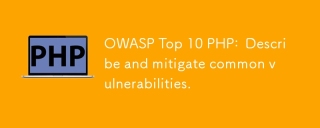
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
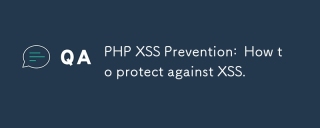
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool