In the process of PHP development, array-related operations are often involved, including array search, addition, modification, deletion, etc. Among them, determining whether an element exists in an array is one of the common operations. In PHP, there are several ways to determine whether an element is in an array.
1. Use the in_array() function
The in_array() function can determine whether the specified element exists in the array. The syntax is as follows:
in_array($needle, $haystack, $strict)
Among them, $needle represents the element to be found, $haystack represents the array to be found, and $strict represents whether to enable it. Strict mode, if enabled, requires the elements to be of the same type as the elements in the array, and the values to be the same.
The following are some examples:
1. Determine whether the number exists in the array
$nums = array(1, 2, 3, 4, 5);
if (in_array(3, $nums)) {
echo "存在";
} else {
echo "不存在";
}
The output result is: existence
2. Judgment Whether the string exists in the array
$fruits = array("apple", "banana", "orange");
if (in_array("banana", $fruits)) {
echo "存在";
} else {
echo "不存在";
}
The output result is: Existence
3. Determine whether the types of elements are the same
$a = 1 ;
$b = "1";
$arr = array(1, "1");
if (in_array($a, $arr, true)) {
echo "存在";
} else {
echo "不存在";
}
if (in_array($b, $arr, true)) {
echo "存在";
} else {
echo "不存在";
}
The output result is: exists or not
2. Use array_search() function
array_search() function can find the position of the specified element in the array. If found , returns the index of the element in the array, otherwise returns false. The syntax is as follows:
array_search($needle, $haystack, $strict)
Among them, $needle represents the element to be searched, $haystack represents the array to be searched, and $strict represents whether to enable it. Strict mode.
The following are some examples:
1. Find the position of the number in the array
$nums = array(1, 2, 3, 4, 5);
$key = array_search(3, $nums);
if ($key !== false) {
echo "该元素在数组中的位置为:" . $key;
} else {
echo "不存在";
}
The output result is: the position of the element in the array is: 2
2. Find the position of the string in the array
$fruits = array("apple", "banana" , "orange");
$key = array_search("banana", $fruits);
if ($key !== false) {
echo "该元素在数组中的位置为:" . $key;
} else {
echo "不存在";
}
The output result is: the position of the element in the array is: 1
3. Use isset() function
isset() function can Used to determine whether the specified key exists in the array. The syntax is as follows:
isset($array[$key])
Among them, $array represents the array to be judged, and $key represents the key to be judged.
The following is an example:
$fruits = array("apple" => 1, "banana" => 2, "orange" => 3);
if (isset($fruits["banana"])) {
echo "存在";
} else {
echo "不存在";
}
The output result is: exists
4. Use array_key_exists() function
array_key_exists() function can determine whether the specified key exists in the array. The syntax is as follows:
array_key_exists($key, $array)
Among them, $key represents the key to be judged, and $array represents the array to be judged.
The following is an example:
$fruits = array("apple" => 1, "banana" => 2, "orange" => 3);
if (array_key_exists("banana", $fruits)) {
echo "存在";
} else {
echo "不存在";
}
The output result is: exists
In summary, PHP provides multiple ways to determine whether an element exists in an array. In actual development, the most appropriate method can be selected according to the specific situation.
The above is the detailed content of php is in the array. For more information, please follow other related articles on the PHP Chinese website!
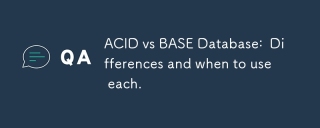
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
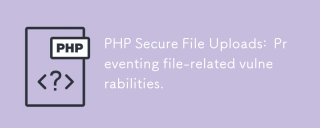
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
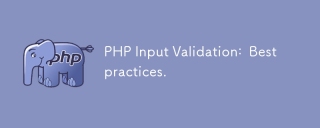
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
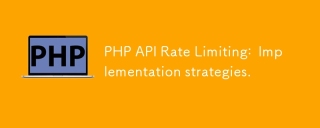
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
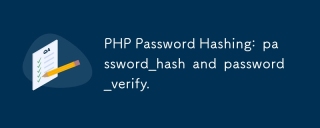
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
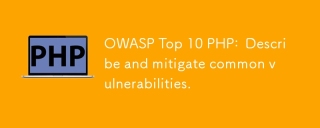
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
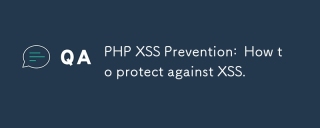
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
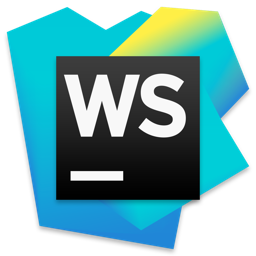
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor