PHP is a very popular server-side language often used for web development. Arrays are a very common data type in PHP. When processing data, we often need to insert new elements into an existing array. This article will introduce various methods on how to insert array elements in PHP.
1. Use the array_push function to insert array elements
The array_push function is a built-in function of PHP that can insert one or more elements to the end of the array. It can accept a variable number of parameters. For example:
$colors = array("red", "green"); array_push($colors, "blue", "yellow"); print_r($colors);
In this example, we first define an array containing two elements. Next, we use the array_push function to insert two new elements into the end of the array. Finally, we used the print_r function to output the final array, and the output result is:
Array ( [0] => red [1] => green [2] => blue [3] => yellow )
2. Use the [] operator to insert array elements
You can also use the [] operator to insert in PHP Array elements. This method is more concise than the array_push function. For example:
$colors = array("red", "green"); $colors[] = "blue"; print_r($colors);
In this example, we first define an array containing two elements. Next, we use the [] operator to insert a new element at the end of the array. Finally, we used the print_r function to output the final array, and the output result is:
Array ( [0] => red [1] => green [2] => blue )
3. Use the array_unshift function to insert array elements
The array_unshift function is another PHP built-in function that can convert an Or multiple elements are inserted at the beginning of an array. For example:
$colors = array("red", "green"); array_unshift($colors, "blue", "yellow"); print_r($colors);
In this example, we first define an array containing two elements. Next, we use the array_unshift function to insert two new elements into the beginning of the array. Finally, we used the print_r function to output the final array, and the output result is:
Array ( [0] => blue [1] => yellow [2] => red [3] => green )
4. Use the array_splice function to insert array elements
The array_splice function is another built-in function of PHP that can be used Remove or insert elements from an array. It can accept a variable number of parameters. For example:
$colors = array("red", "green", "yellow"); array_splice($colors, 1, 0, "blue"); print_r($colors);
In this example, we first define an array containing three elements. Next, we use the array_splice function to insert a new element into the second position of the array. The first parameter specifies the array to operate on, the second parameter specifies the position to insert, the third parameter specifies the number of elements to remove (zero in this case because we don't want to remove any elements), and the fourth parameter Specifies the new element to be inserted. Finally, we used the print_r function to output the final array, and the output result is:
Array ( [0] => red [1] => blue [2] => green [3] => yellow )
Summary
In PHP, there are many ways to insert new elements into the array. The array_push function can insert one or more elements to the end of the array, the [] operator can insert one element to the end of the array, the array_unshift function can insert one or more elements to the beginning of the array, and the array_splice function can insert an element Insert into the array at any position. Choosing a different method depends on your specific needs. The above methods are commonly used in PHP to insert array elements. I hope they will be helpful to you.
The above is the detailed content of How to insert php array. For more information, please follow other related articles on the PHP Chinese website!
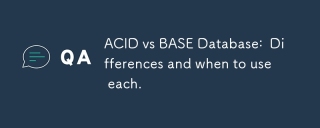
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
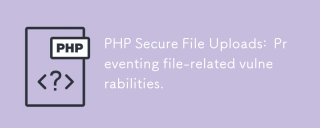
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
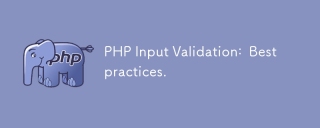
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
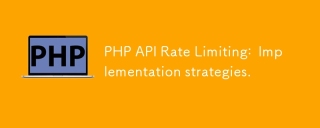
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
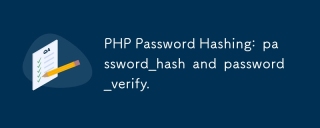
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
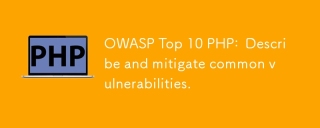
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
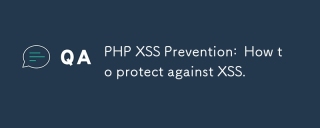
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
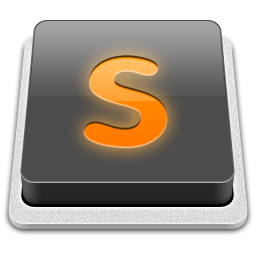
SublimeText3 Mac version
God-level code editing software (SublimeText3)