In PHP, arrays and objects are two commonly used data types. When writing PHP programs, you often need to check whether a variable is an array type in order to perform some corresponding processing.
Generally, we can use some built-in PHP functions to determine whether a variable is an array type, such as is_array(), gettype() and instanceof. These functions and their usage are described in detail below.
is_array() function
is_array() function is a built-in PHP function that checks whether a variable is of array type. The usage of this function is as follows:
bool is_array ( mixed $var )
Among them, $var represents the variable to be checked, and mixed represents that the variable can be any PHP data type. This function returns true when the variable $var is an array, false otherwise.
The following example demonstrates how to use the is_array() function to check whether a variable is an array type:
<?php $arr = array('apple', 'banana', 'orange'); if (is_array($arr)) { echo '$arr is an array'; } else { echo '$arr is not an array'; } ?>
Run the above code, the output result is:
$arr is an array
gettype() Function
gettype() function is another built-in PHP function used to get the data type of a variable. The usage of this function is as follows:
string gettype ( mixed $var )
Among them, $var indicates the variable of the data type to be obtained, and mixed indicates that the variable can be any PHP data type. When the variable $var is an array, this function returns the string "array", otherwise it returns the corresponding data type.
The following example demonstrates how to use the gettype() function to obtain the data type of a variable:
<?php $arr = array('apple', 'banana', 'orange'); $type = gettype($arr); echo '$arr is of type '.$type; ?>
Run the above code, the output result is:
$arr is of type array
instanceof operator
The instanceof operator is an operator used to check whether a variable belongs to a certain class. In PHP, array is a built-in class, so you can use instanceof operator to check if a variable is of array type. The usage of this operator is as follows:
bool (object $object instanceof class $class)
Among them, $object represents the variable to be checked, and $class represents the class to be compared. This operator returns true when the variable $object is an object of class $class, false otherwise.
The following example demonstrates how to use the instanceof operator to check whether a variable is an array type:
<?php $arr = array('apple', 'banana', 'orange'); if ($arr instanceof Array) { echo '$arr is an array'; } else { echo '$arr is not an array'; } ?>
Run the above code, the output result is:
$arr is an array
Summary
In PHP, checking whether a variable is of array type is very simple. We can do this using is_array() function, gettype() function and instanceof operator. Which method to choose depends on the actual situation, but it is generally recommended to use the is_array() function because it is a relatively simple and intuitive way, and can quickly check whether a variable is an array.
The above is the detailed content of php check if array. For more information, please follow other related articles on the PHP Chinese website!
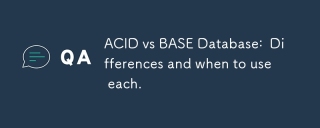
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
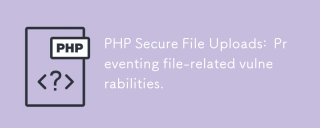
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
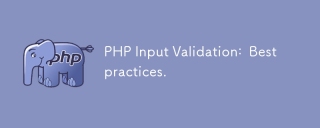
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
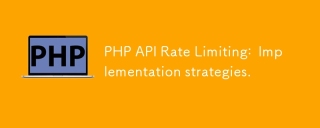
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
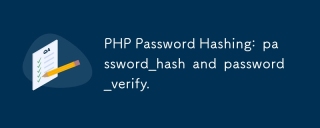
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
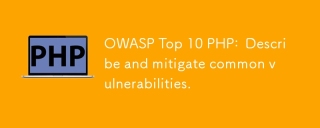
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
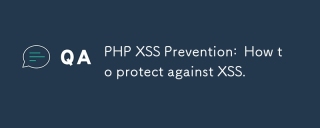
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
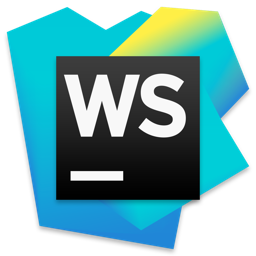
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor