PHP is a powerful programming language that provides many commonly used data structures, such as arrays. An array is a collection of elements that can be accessed based on index or key value. In PHP, we can use arrays to store and manage data. When we use PHP arrays, sometimes we need to determine whether a certain subscript exists. This article will introduce how to determine whether an array subscript exists in PHP.
There are many ways to determine whether an array subscript exists in PHP. We will introduce these methods separately below.
Method 1: Use the isset() function to determine
In PHP, the isset() function is used to check whether a variable has been set and is not NULL. This function can determine whether ordinary variables or array subscripts exist. If the array subscript exists, it returns true; otherwise, it returns false. Here is a demonstration example:
$country = array("China" => "Beijing", "USA" => "Washington D.C.", "Japan" => "Tokyo"); if(isset($country["USA"])) { echo "USA's capital city is ".$country["USA"]."."; } else { echo "Unknown country."; }
In the above example, by using isset() function, we checked whether "USA" exists as an array subscript. If it exists, output "USA's capital city is Washington D.C."; otherwise, output "Unknown country.".
It should be noted that the isset() function also needs to avoid "Notice" warnings when using it. If the array index does not exist, a "Notice: Undefined index" warning will appear. In order to avoid this situation, we can use the PHP built-in function error_reporting() to turn off warning output, or use error_reporting(E_ALL & ~E_NOTICE) to only turn off warning output without affecting other error messages.
Method 2: Use the array_key_exists() function to determine
PHP provides another function to determine whether the array subscript exists, that is, the array_key_exists() function. This function checks whether the specified key exists in the array. If it exists, return true; otherwise, return false. The following is a demonstration example:
$fruit = array("apple", "banana", "orange"); if(array_key_exists(1, $fruit)) { echo "The second fruit in the array is ".$fruit[1]."."; } else { echo "Unknown fruit."; }
In the above example, we use the array_key_exists() function to determine whether array subscript 1 exists. If it exists, output "The second fruit in the array is banana."; otherwise, output "Unknown fruit.".
It should be noted that the first parameter of the array_key_exists() function is the key name, and the second parameter is the array. This function should only be used when you need to determine whether a specific key name exists. When you only need to know whether the key value exists, it is best to use the isset() function.
Method 3: Use the in_array() function to determine
In addition to isset() and array_key_exists() functions, PHP also provides another function to determine whether the array subscript exists, namely in_array( )function. This function searches the array for the specified value and returns a Boolean value. If the specified value is found in the array, it returns true; otherwise, it returns false. The following is a demonstration example:
$color = array("red", "green", "blue"); if(in_array("green", $color)) { echo "Green is in the array."; } else { echo "Not found."; }
In the above example, we use the in_array() function to determine whether the "green" element exists in the array. If it exists, "Green is in the array." is output; otherwise, "Not found." is output.
It should be noted that the first parameter of the in_array() function is the value to be found, and the second parameter is the array. This function can only find values in the array, not keys.
Method 4: Use the key name to traverse the array
When we use foreach to traverse the array, we can use the key name to determine whether the array subscript exists. Here is a demonstration example:
$animal = array("dog" => "golden retriever", "cat" => "persian", "fish" => "goldfish"); foreach($animal as $key => $value) { if($key == "cat") { echo "The breed of cat is ".$value."."; } }
In the above example, we used foreach to traverse the $animal array. When the key name is "cat", the key value corresponding to the key name is output. "$key" represents the key name of the current array element, and "$value" represents the key value of the current array element. If the key name does not exist, the corresponding output statement will not be executed.
It should be noted that by using foreach to traverse the array, you can only determine whether a key name exists, but you cannot repeatedly check whether the key name exists.
Conclusion
In PHP, we can use a variety of methods to determine whether an array subscript exists. Each method has its advantages and disadvantages and should be chosen based on the actual situation. When using these methods, you also need to pay attention to avoid "Notice" warnings and conduct appropriate testing of the code to ensure the correctness and stability of the code.
The above is the detailed content of Does the php array subscript exist?. For more information, please follow other related articles on the PHP Chinese website!
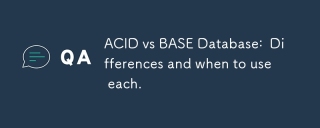
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
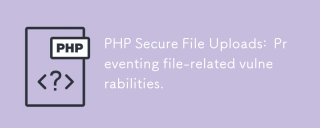
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
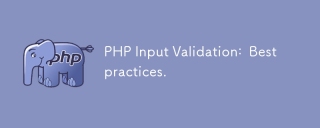
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
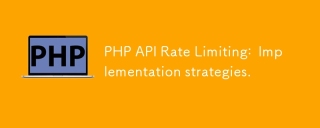
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
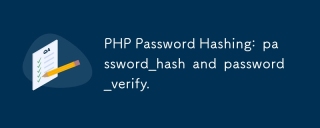
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
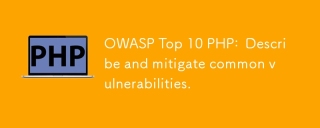
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
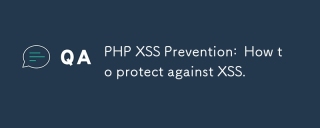
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
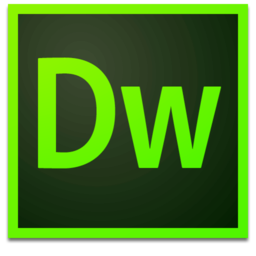
Dreamweaver Mac version
Visual web development tools
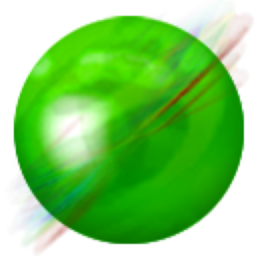
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
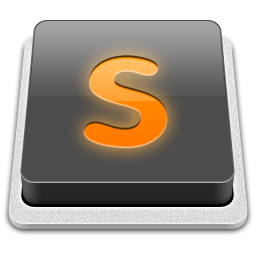
SublimeText3 Mac version
God-level code editing software (SublimeText3)