Golang is a language widely used in system programming, Web development, network programming and other fields. It has the advantages of efficiency, simplicity, and easy learning, and is deeply loved by developers. In Golang programming, code jumping is also a common operation. This article will introduce the knowledge and usage of code jump in Golang.
1. Jump basics
In Golang, there are two ways to jump to code: goto statements and labels, which together realize non-sequential execution of the program.
- goto statement
The goto statement is used to unconditionally jump to the specified identifier (i.e. label). It can be used to break out of multi-level loops (for, switch, select), and can also be used for error checking and other control flow operations. The syntax of the goto statement is as follows:
goto label
where label is an identifier, which can be any legal identifier or an identifier that needs to be defined. For example:
goto End ... End: fmt.Println("end")
The End here is a label, and the goto statement will unconditionally jump to the location of the label, that is, execute the fmt.Println("end") statement.
- tag
The tag is the identifier defined for the goto statement. They are used to identify a location in a program and are defined in the form of a colon (:) followed by an identifier, for example:
End: fmt.Println("end")
Here, End is a label followed by a line of code. When the goto statement reaches the label, this line of code is executed.
It should be noted that the goto statement can only jump to defined labels. Undefined labels will cause compilation errors.
2. Jump out of multi-layer loops
In Golang programming, sometimes you need to jump out of multi-layer loops. At this time, goto statements and labels can come in handy.
Suppose there is a nested for loop now:
for i := 0; i < 10; i++ { for j := 0; j < 10; j++ { if i+j > 15 { // 跳出两层循环 } } }
If you want to jump out of the two-level loop when the if condition is true, you can use goto statements and labels to achieve this:
outer: for i := 0; i < 10; i++ { for j := 0; j < 10; j++ { if i+j > 15 { goto outer } } }
Here, outer is a label that defines the block-level scope of a for loop. When the condition is true, the goto statement jumps to the location of the outer label, that is, it jumps out of the two-level loop.
3. Error Checking
In Golang programming, error handling and checking are often required. goto statements and labels can make error checking easier and more efficient.
For example, the following is a code that reads data from a file:
f, err := os.Open("file.txt") if err != nil { fmt.Println("open file failed:", err) return } defer f.Close() ...
Here, if the file fails to open, the program will print an error message and terminate execution.
However, if the developer wants to continue performing other operations in the program after the file opening fails, they need to use goto statements and labels.
f, err := os.Open("file.txt") if err != nil { fmt.Println("open file failed:", err) goto ERR_EXIT } defer f.Close() ... ERR_EXIT: fmt.Println("error exit")
Here, ERR_EXIT is a label used to jump to the last execution of the program. If opening the file fails, the program will execute the goto statement, jump to the ERR_EXIT label, print the error message, and then continue executing the last statement of the program.
4. Summary
This article introduces the knowledge and usage of Golang's code jump. In Golang programming, jumps are often used to jump out of multi-layer loops, error checking and other operations, which can effectively improve the flexibility and robustness of the program.
It should be noted that excessive use of goto statements and labels may lead to a decrease in the readability and maintainability of the program, so you should be cautious when using them, and make appropriate analysis and judgment based on the actual situation.
The above is the detailed content of golang code jump. For more information, please follow other related articles on the PHP Chinese website!
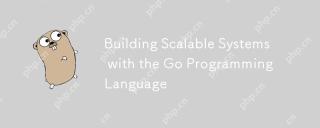
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
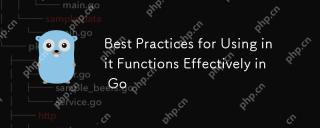
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
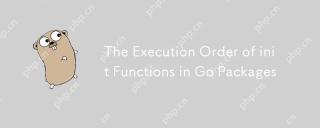
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations
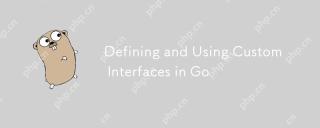
CustominterfacesinGoarecrucialforwritingflexible,maintainable,andtestablecode.Theyenabledeveloperstofocusonbehavioroverimplementation,enhancingmodularityandrobustness.Bydefiningmethodsignaturesthattypesmustimplement,interfacesallowforcodereusabilitya
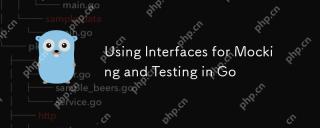
The reason for using interfaces for simulation and testing is that the interface allows the definition of contracts without specifying implementations, making the tests more isolated and easy to maintain. 1) Implicit implementation of the interface makes it simple to create mock objects, which can replace real implementations in testing. 2) Using interfaces can easily replace the real implementation of the service in unit tests, reducing test complexity and time. 3) The flexibility provided by the interface allows for changes in simulated behavior for different test cases. 4) Interfaces help design testable code from the beginning, improving the modularity and maintainability of the code.
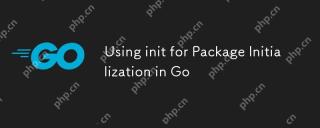
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
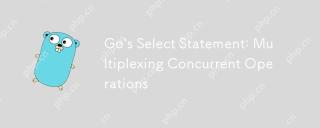
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
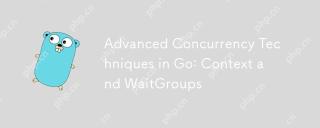
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
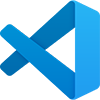
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment
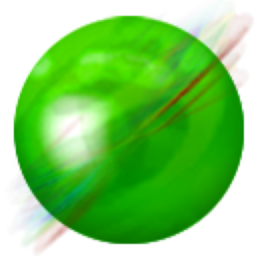
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
