With the rise of Golang language in recent years, more and more developers have begun to come into contact with and use this language for development, and Golang functions are an important part of its development. So, how to write a high-quality Golang function? Let us find out together below.
1. Function declaration
In Go, a function is declared using the keyword func, and the syntax is as follows:
func 函数名(参数列表) (返回值列表) { // 函数体 }
Among them, the function name follows the naming rules of identifiers, and the parameter list Both the return value list and the return value list can be empty, and multiple return value lists are separated by commas. For example:
func sayHello() { fmt.Println("Hello, world!") } func add(x int, y int) int { return x + y } func swap(x, y string) (string, string) { return y, x }
2. Function parameters
In Go, function parameters are passed by value, which means that the parameters of the function will be copied to the function stack instead of Pass the reference directly. Therefore, if the value of the parameter is modified in the function, the original parameter value will not be affected, as shown below:
func main() { x := 10 modify(x) fmt.Println(x) // 输出: 10 } func modify(x int) { x = 20 }
If you want to modify the parameter value, you can use a pointer to achieve it, as shown below:
func main() { x := 10 modify(&x) fmt.Println(x) // 输出: 20 } func modify(x *int) { *x = 20 }
Note that you need to use & to get the variable address and * to dereference the variable pointer.
3. Anonymous function
Anonymous function is a function type that does not need to be named and can be defined and called directly. In Go, you can use anonymous functions to implement closures, that is, access variables outside the function from within the function. For example:
func main() { x := 10 add := func(y int) int { return x + y } fmt.Println(add(20)) // 输出: 30 }
In this example, we define an add anonymous function, which uses the external variable x to implement an addition operation.
4. Functions as parameters
In the Go language, functions are first-class citizens, and functions can be passed to another function as parameters. For example:
func main() { x := []int{1, 2, 3, 4, 5} fmt.Println(sum(x, func(v int) int { return v * 2 })) // 输出: 30 } func sum(x []int, f func(int) int) int { sum := 0 for _, v := range x { sum += f(v) } return sum }
In this example, we define a sum function that receives an array of integers and a function for processing integers. We pass the function as a parameter to the sum function and use the passed function to process each element in the array, ultimately implementing the sum operation.
5. Variable parameters
The Go language supports variable parameter functions, that is, the number of function parameters can be uncertain. We can use the ... operator to achieve this functionality. For example:
func main() { fmt.Println(add(1, 2, 3, 4, 5)) // 输出: 15 } func add(nums ...int) int { sum := 0 for _, num := range nums { sum += num } return sum }
In this example, we define an add function that receives any number of int type parameters and returns their sum. A variadic argument is actually a slice, and we can operate on it just like a slice.
In short, the writing of Golang functions is one of the cores of Golang development and is crucial to the writing of high-quality code. Here we give a brief introduction to the basic knowledge of Golang functions, hoping to help Golang developers better understand the use of Golang functions.
The above is the detailed content of How to write golang function. For more information, please follow other related articles on the PHP Chinese website!
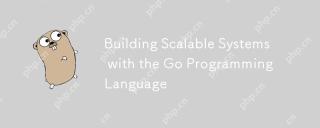
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
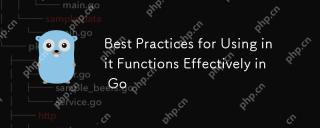
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
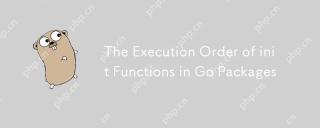
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations
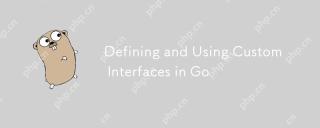
CustominterfacesinGoarecrucialforwritingflexible,maintainable,andtestablecode.Theyenabledeveloperstofocusonbehavioroverimplementation,enhancingmodularityandrobustness.Bydefiningmethodsignaturesthattypesmustimplement,interfacesallowforcodereusabilitya
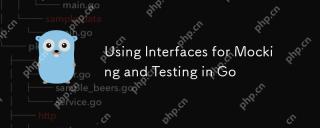
The reason for using interfaces for simulation and testing is that the interface allows the definition of contracts without specifying implementations, making the tests more isolated and easy to maintain. 1) Implicit implementation of the interface makes it simple to create mock objects, which can replace real implementations in testing. 2) Using interfaces can easily replace the real implementation of the service in unit tests, reducing test complexity and time. 3) The flexibility provided by the interface allows for changes in simulated behavior for different test cases. 4) Interfaces help design testable code from the beginning, improving the modularity and maintainability of the code.
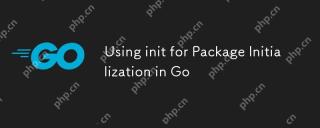
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
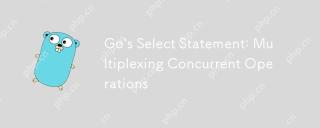
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
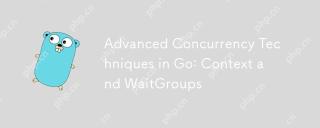
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
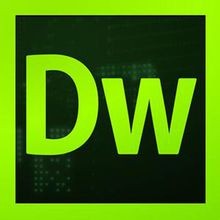
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
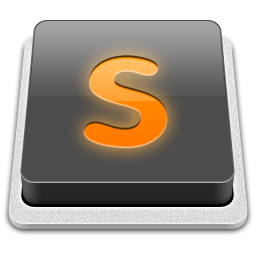
SublimeText3 Mac version
God-level code editing software (SublimeText3)
