How to use PHP for audio file processing?
With the widespread use of audio files, more and more applications require audio file processing. PHP is a popular server-side scripting language that can be used to process audio files. This article will introduce how to use PHP for audio file processing.
1. Understand audio file formats
Before using PHP to process audio files, you need to understand audio files in different formats. Common audio file formats include MP3, WAV, FLAC, etc. These file formats have different encoding methods, compression methods and data storage methods. When processing audio files, you need to choose different processing methods according to the file format.
2. Reading audio files
PHP provides many functions and classes for reading audio files. Commonly used functions include file_get_contents(), fread(), fgets(), etc. If you want to read a large audio file, you can use the fread() function, which can read the file in chunks. If you want to read a text file, you can use the fgets() function to read the file contents line by line.
3. Process audio files
After reading the audio file, various processing needs to be performed. These processes include audio file conversion, audio file clipping, and audio file merging.
1. Audio file conversion
PHP can convert audio files in different formats into other formats. For example, you can convert audio files in MP3 format to WAV format files.
Use the FFmpeg library in PHP for audio file conversion:
<?php exec("ffmpeg -i input.mp3 output.wav"); ?>
2. Audio file clipping
You can use PHP to intercept specific parts of the audio file. For example, you can capture the first 30 seconds of a song.
Use PHP's built-in audio processing library - Sonic
<?php include_once('sonic.php'); $sonic = new Sonic(44100, 2); $input_file = 'song.mp3'; $output_file = 'song-30-seconds.mp3'; $sonic->open(44100, 2); $fp = fopen($input_file, 'rb'); $fout = fopen($output_file, 'wb'); $end_pos = 44100 * 2 * 30; $cur_pos = 0; while ($buffer = fread($fp, 4096*2)) { $cur_pos += 4096*2; if ($cur_pos > $end_pos) break; fwrite($fout, $sonic->addData($buffer)); } $sonic->flush(); fwrited($fout, $sonic->getdata()); $sonic->close(); fclose($fp); fclose($fout); ?>
3. Audio file merging
You can use PHP to merge multiple audio files into one file. For example, multiple songs can be combined into a complete album.
Use the FFmpeg library in PHP to merge audio files:
<?php exec("ffmpeg -i 'concat:1.mp3|2.mp3|3.mp3' output.mp3"); ?>
4. Save the audio file
After processing the audio file, you need to save it to the hard disk or upload it to the server. You can use functions such as fwrite() and file_put_contents() in PHP to write files to disk or upload them to the server.
<?php file_put_contents('output.mp3', $output_data); ?>
The above is a brief introduction on how to use PHP for audio file processing. By studying this article, readers can learn how to read, process and save audio files in PHP. It is hoped that readers can have a deeper understanding and use of this knowledge in practical applications.
The above is the detailed content of How to use PHP for audio file processing?. For more information, please follow other related articles on the PHP Chinese website!
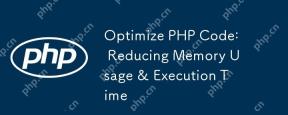
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
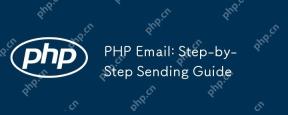
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
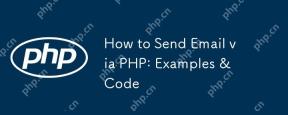
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
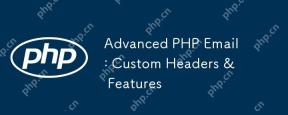
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
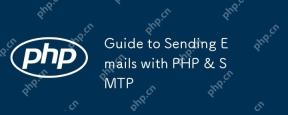
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
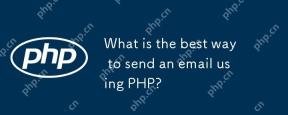
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
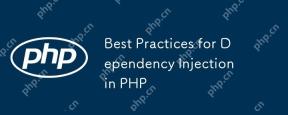
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
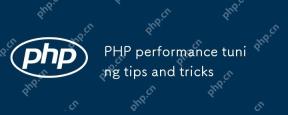
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
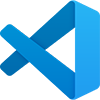
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
