In PHP programming, arrays and strings are very common data types. Arrays are usually used to store the same or different data types, such as numbers and strings, while strings are usually a continuous sequence of characters consisting of a number of characters.
Sometimes, we need to convert an array to a string or a string to an array. In this article, we will discuss in detail how to do this type of conversion in PHP.
Convert an array to a string
In PHP, you can use the implode() function to convert an array to a string. The implode() function concatenates the elements of an array together, separated by a specified delimiter.
The following is an example of converting an array to a string:
$colors = array('red', 'blue', 'green'); $string = implode(',', $colors); echo $string; // Output: red,blue,green
First, we define an array containing colors. We then use the implode() function to convert the array into a string where each element is separated by commas. Finally, we output the string to the screen.
You can also convert the array to a delimiter-less string by omitting the delimiter in the implode() function, as shown below:
$colors = array('red', 'blue', 'green'); $string = implode('', $colors); echo $string; // Output: redbluegreen
The above code uses the same array, but We omitted the delimiter in the implode() function. As a result, the function returns an undelimited string and prints it to the screen.
Convert a string to an array
In PHP, you can use the explode() function to split a string into an array. The explode() function requires two parameters: the delimiter to be used and the string to be split.
The following is an example of using the explode() function to convert a string into an array:
$string = 'red,blue,green'; $colors = explode(',', $string); print_r($colors); // Output: Array ( [0] => red [1] => blue [2] => green )
First, we define a string containing comma delimiters. Then, we use the explode() function to split the string into an array, using commas as delimiters. Finally, we print the entire array using the print_r() function.
Please note that the explode() function returns an array where each element is a delimiter-separated portion of the original string.
If the delimiter does not exist in the string, the explode() function returns a single-element array containing the entire string:
$string = 'Hello, World!'; $words = explode(',', $string); print_r($words); // Output: Array ( [0] => Hello [1] => World! )
The above code defines a character that contains the comma delimiter string. However, since the comma delimiter does not exist in the string, the explode() function will return a single-element array containing the entire string and print it to the screen.
Conclusion
Arrays and strings are very common data types in PHP programming. Sometimes, we need to convert an array to string or a string to array. In this article, we explained how to convert an array to a string using the implode() function and how to split a string into an array using the explode() function. These conversion functions are very convenient and easy to use, and are one of the indispensable tools in PHP programming.
The above is the detailed content of php array string conversion. For more information, please follow other related articles on the PHP Chinese website!
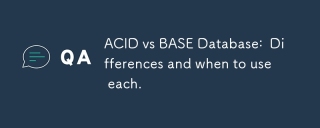
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
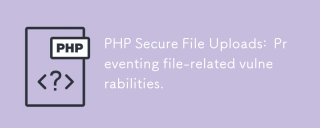
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
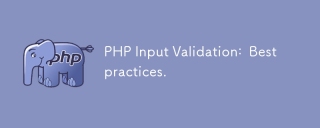
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
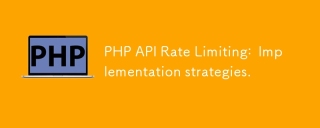
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
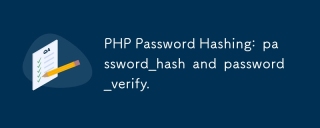
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
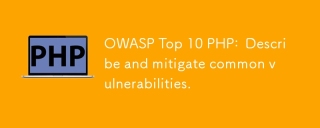
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
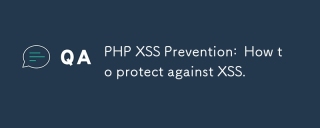
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
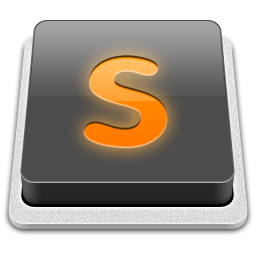
SublimeText3 Mac version
God-level code editing software (SublimeText3)
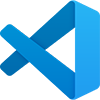
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft