PHP is a powerful programming language that provides many methods for operating arrays. Array is a very practical data structure that can be used to store a group of related data, such as a product list or a student list. When using an array, we may need to modify some of its values. This article will introduce several ways to modify the values in PHP arrays.
1. Use array subscripts to assign values
Arrays in PHP can use subscripts to access and modify their values. The subscript starts from 0 and can be of any type (integer, floating point number, string, etc.). We can modify the corresponding value by assigning a value to the array subscript. Here is an example:
$fruits = array('apple', 'banana', 'orange'); $fruits[1] = 'pear'; print_r($fruits);
Output:
Array ( [0] => apple [1] => pear [2] => orange )
In the above example, we modified the value at index 1 in the $fruits array to 'pear'. The output indicates that the element in the array has been successfully modified.
2. Use the array_splice() function
The array_splice() function can be used to delete one or more elements in the array and insert new elements at the deleted positions. This function is used as follows:
array_splice(array &$input, int $offset, int $length = 0, mixed $replacement = array()): array
Among them, the parameter $input specifies the array to be operated on; $offset specifies the starting position of deletion/insertion; $length specifies the number of elements to be deleted (the default is 0, that is No elements are deleted); $replacement specifies the element to be inserted. Below is an example that shows how to use the array_splice() function to replace the values of certain elements in an array.
$names = array('Tom', 'Jerry', 'Spike', 'Tyke'); array_splice($names, 2, 1, 'Butch'); print_r($names);
Output:
Array ( [0] => Tom [1] => Jerry [2] => Butch [3] => Tyke )
In the above example, we deleted the element with index 2 in the $names array and inserted a new element 'Butch' at that position.
3. Use foreach loop
The foreach loop can be used to traverse each element in the array and modify it. We can use the reference ampersand symbol in a loop to modify the value of an element. For example:
$grades = array('John' => 85, 'Mary' => 92, 'Bob' => 78); foreach ($grades as &$value) { $value = $value + 5; } print_r($grades);
Output:
Array ( [John] => 90 [Mary] => 97 [Bob] => 83 )
In the above example, we used a foreach loop to traverse each element in the $grades array and modify it. Due to the use of the reference & symbol, we can directly modify the value of the corresponding element in the original array.
Summary
Through the above three methods, we can easily modify the element values in the PHP array. Usually, the first method (assignment using array subscripts) is the most common and simple. Using the array_splice() function, you can delete elements and insert new elements into the array at the same time, which has a certain degree of flexibility. When we need to modify each element in the array, we can use a foreach loop and use the reference & symbol to directly modify the element value in the array. The selection of these methods requires judgment and selection based on specific usage scenarios and needs.
The above is the detailed content of How to change the value in an array in php. For more information, please follow other related articles on the PHP Chinese website!
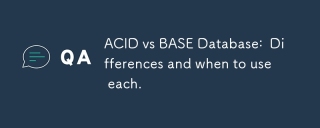
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
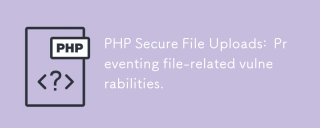
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
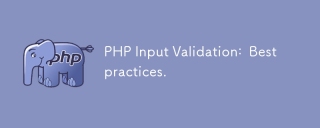
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
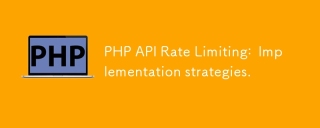
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
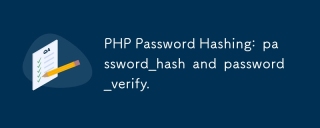
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
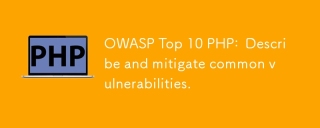
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
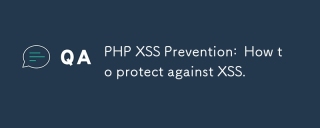
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
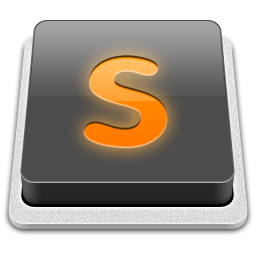
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.