The singleton pattern is a common design pattern that ensures that only one instance of a class exists and provides a global access point so that external programs can obtain the instance. There are many different ways to implement the singleton pattern in PHP, and this article will introduce one of them.
1. What is the singleton pattern?
The singleton pattern is a commonly used design pattern in object-oriented programming. It can ensure that only one instance of a class exists and provide an access to the instance. global entry point. The singleton mode is usually used when only one instance is needed to manage resources, configuration information, etc., and can provide more efficient resource utilization.
In PHP, we can implement the singleton pattern by restricting the instantiation of a class and providing a static access method. Let's take a look at how to implement the singleton pattern.
2. Code Implementation
In PHP, we can implement the singleton mode through the following steps:
- Private constructor: In order to prevent the class from being externalized If the program instantiates directly, we need to privatize the constructor of the class so that the class can only obtain instances through static methods.
- Save the unique instance: In order to ensure that the instance obtained each time is the same, we need to save the unique instance in the class and judge and instantiate it in the static method.
- Provide global access point: In order to facilitate external programs to use instances of the class, we need to provide a static access method in the class to obtain the instance.
Below we use code to demonstrate how to implement the singleton mode:
class Singleton { //保存唯一实例的静态变量 private static $instance; //私有化构造函数 private function __construct() { //初始化处理代码 //... } //静态方法获取实例 public static function getInstance() { //如果实例不存在,就进行实例化 if (!isset(self::$instance)) { self::$instance = new self(); } //返回唯一实例 return self::$instance; } //禁止克隆实例 private function __clone() { //禁止克隆实例 } //禁止反序列化 private function __wakeup() { //禁止反序列化 } //其他方法 //... }
As shown in the above code, we defined a static variable $instance in the Singleton class to save the only instance. . In the getInstance method, we decide whether to instantiate the class by judging whether $instance exists and return the only instance. At the same time, we also privatize the constructor, clone method, and deserialization method of the class to prevent instances of the class from being directly created, copied, or deserialized by external programs.
3. Use singleton mode
In actual applications, using singleton mode can usually improve system performance and resource utilization efficiency. Let's take a look at how to use the Singleton class:
//获取 Singleton 类的实例 $singleton1 = Singleton::getInstance(); $singleton2 = Singleton::getInstance(); //判断两个实例是否相同 if ($singleton1 === $singleton2) { echo '实例相同'; } else { echo '实例不同'; }
In the above code, we obtain an instance of the Singleton class through the Singleton::getInstance() method and save it in the $singleton1 and $singleton2 variables. Since there is only one instance of the Singleton class, $singleton1 and $singleton2 should be the same. We can verify that the Singleton class implements the singleton pattern by determining whether they are the same.
4. Summary
The singleton pattern is a commonly used design pattern that allows a class to have only one instance and provides a global access point to obtain the instance. In PHP, you can implement the singleton pattern by restricting the instantiation of a class and providing a static access method. Implementing the singleton pattern can improve system performance and resource utilization efficiency, but you also need to pay attention to the security and maintainability of the code.
The above is the detailed content of How to use php to implement single column mode. For more information, please follow other related articles on the PHP Chinese website!
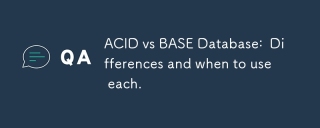
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
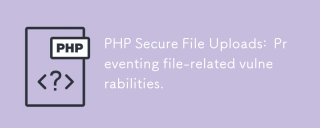
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
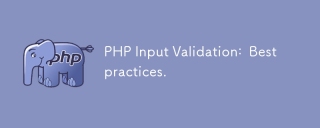
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
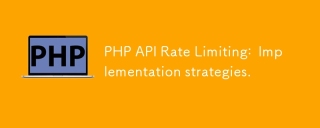
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
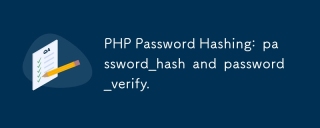
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
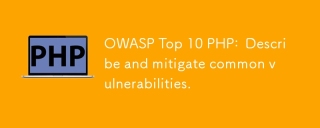
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
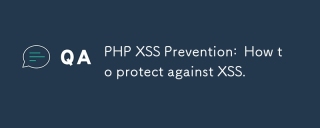
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
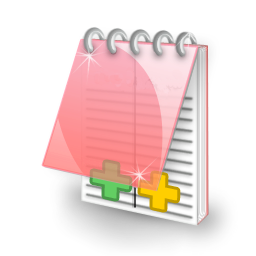
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
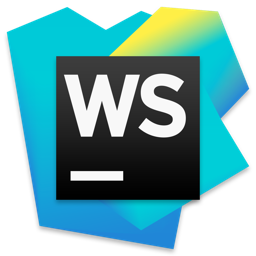
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment