In PHP, array is a very common and useful data type. When you need to calculate the number of specific elements in an array, you can use the built-in functions provided by PHP to make the operation simpler and easier to use.
This article will introduce how to use PHP built-in functions to query the number of specified elements in an array. Through the introduction of this article, readers will learn how to use PHP arrays, how to traverse arrays, how to use built-in functions to calculate array length and the number of occurrences of elements, etc.
1. Use the array_count_values() function to get the number of occurrences of an element
PHP provides a built-in function called array_count_values(), which is used to get the number of occurrences of an element in an array. This function receives an array as a parameter and returns a new array. The key of this new array is the element value in the original array, and the value is the number of times this element value appears in the original array.
The following is an example of using the array_count_values() function to calculate the number of occurrences of array elements:
$arr = array(1, 2, 3, 2, 2, 4, 5, 5); $counts = array_count_values($arr); print_r($counts);
The execution results are as follows:
Array ( [1] => 1 [2] => 3 [3] => 1 [4] => 1 [5] => 2 )
In the above example, we define an array $ arr, which has 8 elements, then the array_count_values() function is called, passing $arr as a parameter to the function, and the function returns a new array $counts, which represents the number of occurrences of each element in $arr. As you can see, 2 appears 3 times in the array $arr, so the value corresponding to 2 in the $counts array is 3.
However, the array_count_values() function can only count the number of occurrences of array elements whose values are string or integer types. For other types of elements, the function will return false. When using this function, you need to pay attention to the type issue, otherwise it will cause errors.
2. Use a for loop to check the number of occurrences of elements one by one
In addition to using the array_count_values() function, we can also use a for loop to check the elements in the array one by one and count the number of occurrences.
The following is an example of using a for loop to calculate the number of occurrences of an array element:
$arr = array(1, 2, 3, 2, 2, 4, 5, 5); $count = 0; $target = 2; for ($i = 0; $i <p>The execution results are as follows: </p><pre class="brush:php;toolbar:false">2出现了3次
In the above example, we defined an array $arr, There are 8 elements in it, and we also define a variable $target, which represents the target value we want to query. Then, we use a for loop to traverse the array and check the elements in the array one by one. If this element is equal to $target, add 1 to the value of the counter $count.
Finally, we output the number of times the target value $target appears in the array $arr.
It should be noted that when using a for loop to calculate the number of occurrences of an array element, you need to use the count() function to obtain the length of the array in order to loop through each array element.
3. Use foreach loop to traverse the array and count the number of occurrences of elements
In addition to using the for loop to check elements one by one, we can also use the foreach statement to traverse the array and count the number of occurrences of elements. Compared with a for loop, the foreach statement can traverse an array more concisely and clearly.
The following is an example of using a foreach loop to count the number of occurrences of array elements:
$arr = array(1, 2, 3, 2, 2, 4, 5, 5); $count = 0; $target = 2; foreach ($arr as $value) { if ($value === $target) { $count++; } } echo $target . "出现了" . $count . "次";
The execution results are as follows:
2出现了3次
In the above example, we use the foreach statement to traverse the array $arr , for each array element, if the element value is equal to the target value $target, then add 1 to the value of the counter $count.
It should be noted that when using the foreach statement to traverse an array, you can use the $key=>$value syntax, where $key represents the subscript of the array element and $value represents the value of the array element. If you do not need to use the $key variable, you can omit it.
Summary
In PHP, it is very convenient to query the number of specified elements in an array. There are three methods to achieve this:
1. Use the array_count_values() function to obtain the occurrence of elements. Number of times
2. Use a for loop to check the number of occurrences of elements one by one
3. Use the foreach statement to traverse the array and count the number of occurrences of elements
You need to choose the appropriate method according to the specific situation. At the same time, you need to pay attention to the type of array elements to avoid errors. I hope this article can inspire and help PHP array operations.
The above is the detailed content of PHP query the number of specified elements in an array. For more information, please follow other related articles on the PHP Chinese website!
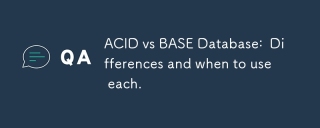
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
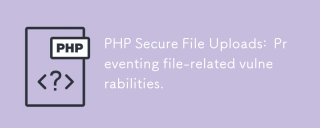
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
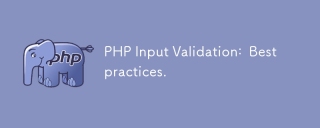
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
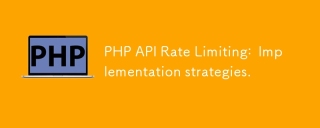
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
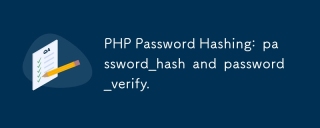
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
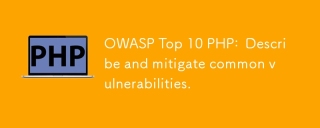
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
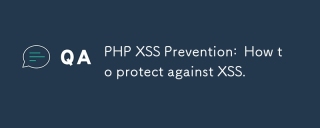
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
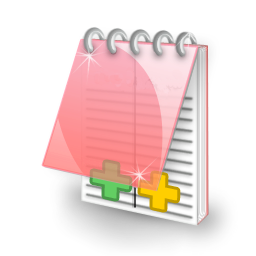
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment