In PHP, if we need to save some elements in a list, the most natural idea is to use an array. Arrays can easily store large numbers of elements and support a range of powerful operations. However, when our array contains duplicate elements, we may need to do some additional operations to find these duplicate values. This article will explain how to query an array for duplicate values in PHP.
1. Use the array_count_values function
PHP's array_count_values function can count the occurrences of each value in the array and return a new array, where the key is the value in the original array and the value is The number of times this value occurs in the original array. Therefore, we can use this function to find duplicate values. Here is a simple example:
$numbers = array(1, 2, 3, 2, 4, 1, 5, 2); $counts = array_count_values($numbers); $repeats = array(); foreach ($counts as $value => $count) { if ($count > 1) { $repeats[] = $value; } } print_r($repeats);
Output result:
Array ( [0] => 1 [1] => 2 )
In the above example, we first define an array $numbers that contains repeated values. We then called the array_count_values function to count the number of occurrences of each value. The output is an array containing two key-value pairs, where the key is the value and the value is the number of times that value occurs.
We then use a foreach loop to iterate through the array and check if the number of occurrences of each value is greater than 1. If it is, the value is added to another array $repeats. Finally, we print the $repeats array to the screen using the print_r function to show the repeated values.
2. Use array_unique function and counting function
Another way to find duplicate values in an array is to use array_unique function and counting function. The array_unique function returns a deduplicated array, while the counting function counts the number of times each value appears in the array. Therefore, we can use these two functions to find duplicate values. The following is an example:
$numbers = array(1, 2, 3, 2, 4, 1, 5, 2); $unique = array_unique($numbers); $counts = array_count_values($numbers); $repeats = array(); foreach ($unique as $value) { if ($counts[$value] > 1) { $repeats[] = $value; } } print_r($repeats);
The output result is the same as the previous example:
Array ( [0] => 1 [1] => 2 )
In the above example, we first use the array_unique function to remove duplicate values in the $numbers array, and get A new array $unique that does not contain duplicate values. We then use the array_count_values function to count the number of occurrences of each value in the original array.
Next, we use a foreach loop to loop through the $unique array and use a count function to check if the number of times the value appears in the original array is greater than 1. If it is, then the value is added to a new array $repeats and finally the $repeats array is output to the screen to show the repeated values.
3. Use the array_keys function
Another way to find duplicate values in an array is to use the array_keys function. This function returns an array containing all keys with the specified value. Therefore, if we use this function to find duplicate values, the length of the resulting array will be the number of duplicate values. The following is an example:
$numbers = array(1, 2, 3, 2, 4, 1, 5, 2); $repeats = array(); foreach (array_unique($numbers) as $value) { $keys = array_keys($numbers, $value); if (count($keys) > 1) { $repeats[] = $value; } } print_r($repeats);
The output result is also the same:
Array ( [0] => 1 [1] => 2 )
In the above example, we first use the array_unique function to remove duplicate values in the $numbers array and get a unique A new array containing duplicate values. We then use a foreach loop to loop through the array and use the array_keys function to find all keys in the original array that contain that value. If the number of keys found is greater than 1, the value is added to a new array $repeats. Finally, we print the $repeats array to the screen to show the repeated values.
Summary
The above are three methods for querying duplicate values in PHP arrays. Each method performs slightly differently, but all methods are effective at finding duplicate values. By learning these methods, you can better master the techniques of array manipulation in PHP and can more easily handle duplicate elements in arrays in your projects.
The above is the detailed content of How to query array for duplicate values in PHP. For more information, please follow other related articles on the PHP Chinese website!
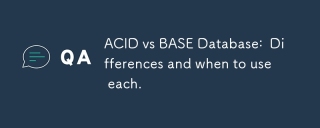
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
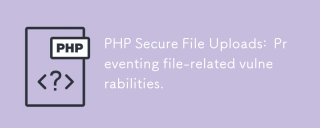
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
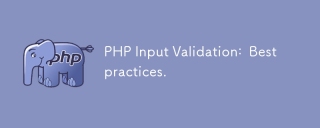
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
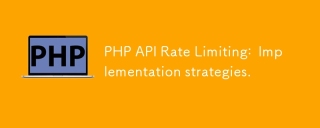
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
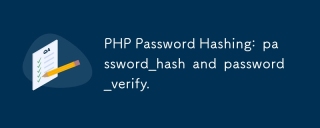
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
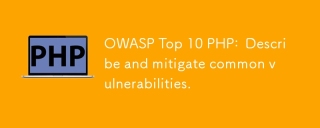
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
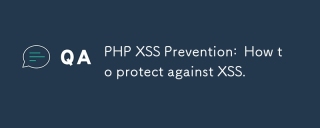
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
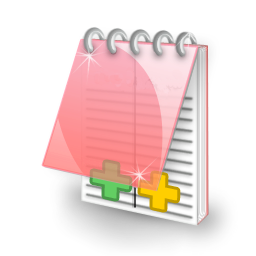
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool