In PHP, passing parameters is a very important operation. In a function or method, passing in parameters allows you to pass data and information that needs to be processed so that they can be processed further. When it comes to arrays, PHP provides corresponding ways to pass array parameters efficiently.
One-dimensional array passing
When you need to pass a one-dimensional array as a parameter, you can use the function's parameters to pass the array. Here is a simple example:
function showArray($arr) { foreach($arr as $value) { echo $value . "<br>"; } } $myArray = array("apple", "orange", "banana"); showArray($myArray);
In this example, we create a function called showArray()
that accepts a parameter $arr
. When we call this function, we pass an array array("apple", "orange", "banana")
. Then, in the function, we use the foreach
loop to iterate through the array to print each value.
Multidimensional array passing
PHP allows you to pass multidimensional arrays by passing the array to a function or method. Here is a simple example:
function showMultiArray($arr) { foreach($arr as $value) { if(is_array($value)) { showMultiArray($value); } else { echo $value . "<br>"; } } } $myArray = array( "fruit" => array("apple", "orange", "banana"), "vegetable" => array("carrot", "pepper", "onion") ); showMultiArray($myArray);
In this example, we create a function called showMultiArray()
that accepts a multidimensional array $arr
. When we call this function, we pass a multidimensional array containing a "fruit" array and a "vegetable" array. We use foreach
to loop through this multidimensional array and check if each value is an array. If it is an array, the showMultiArray()
function is called recursively to iterate over the children of the array. Otherwise, we print out the value.
Passing arrays via the & symbol
By default, PHP copies arrays when passing them to functions. This means that modifying the array inside the function does not affect the original array. But sometimes, you may need to modify the original array inside the function. To do this, you can pass an array by reference. You can pass a variable of an array before a function parameter using the &
notation to pass its reference to the function. This means that when the array is modified inside the function, the original array is affected. Here is a simple example:
function addValues(&$arr) { $arr[] = "cat"; } $myArray = array("dog", "fish"); addValues($myArray); print_r($myArray);
In this example we create a function called addValues()
and pass it # using a &
symbolic reference ##$arr Array. When we call this function and pass
$myArray to it, it will add a new item "cat" to the original array. In the
print_r() function, we print out the contents of the original array, showing the newly added "cat".
The above is the detailed content of How to pass in an array parameter in php. For more information, please follow other related articles on the PHP Chinese website!
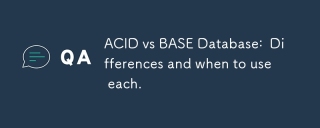
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
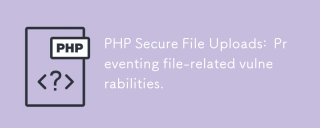
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
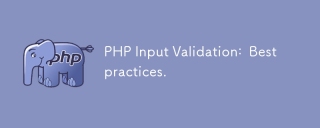
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
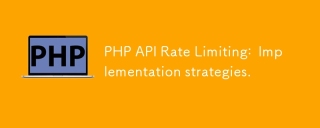
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
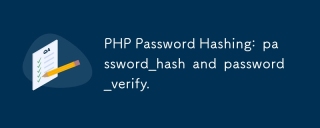
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
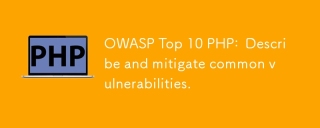
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
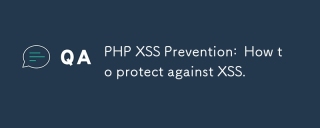
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
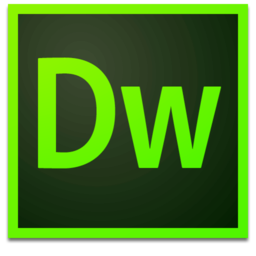
Dreamweaver Mac version
Visual web development tools
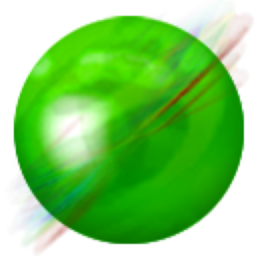
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
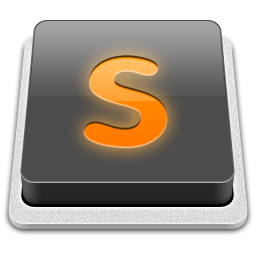
SublimeText3 Mac version
God-level code editing software (SublimeText3)