PHP array is a very commonly used data structure that can store a series of data values. In actual use, it will involve sorting the data. This article will introduce how to sort the elements of the PHP array.
1. The basic concept of array sorting
Array sorting refers to the process of arranging the elements in an array in a certain order. The purpose is to facilitate the search, storage, output, etc. of data. operate.
There are two functions for sorting arrays in PHP, namely sort() and rsort(). The sort() function is for ascending sorting and the rsort() function is for descending sorting.
2. Sort() function
The sort() function can sort the indexes of the PHP array from small to large. The syntax format of the sort() function is as follows:
sort($array);
where $array is the array that needs to be sorted.
The following is an example of sorting an array in ascending order:
<?php $fruits = array("apple", "orange", "grape", "banana"); sort($fruits); print_r($fruits); ?>
The output results are as follows:
Array ( [0] => apple [1] => banana [2] => grape [3] => orange )
It can be seen from the output results that the sort() function sorts the elements in the array The elements are arranged in alphabetical order, realizing the function of ascending order.
3. rsort() function
The rsort() function is similar to the sort() function, except that it sorts the elements of the array from large to small. The syntax format of the rsort() function is as follows:
rsort($array);
where $array is the array that needs to be sorted.
The following is an example of sorting an array in descending order:
<?php $numbers = array(4, 2, 8, 6); rsort($numbers); print_r($numbers); ?>
The output results are as follows:
Array ( [0] => 8 [1] => 6 [2] => 4 [3] => 2 )
As can be seen from the output results, the rsort() function sorts the The elements are arranged in order from largest to smallest.
4. asort() function
asort() function can sort PHP arrays according to values. After sorting, the relationship between keys and values remains unchanged. The syntax format of the function As follows:
asort($array);
Among them, $array is the array that needs to be sorted.
The following is an example of sorting an array in ascending order:
<?php $numbers = array(4, 2, 8, 6); asort($numbers); print_r($numbers); ?>
The output results are as follows:
Array ( [1] => 2 [0] => 4 [3] => 6 [2] => 8 )
As can be seen from the output results, the asort() function sorts the elements in the array The elements are arranged in ascending order, while keeping the key-value association unchanged.
5. arsort() function
The arsort() function is similar to the asort() function, except that it sorts the elements of the array from large to small. The syntax format of the arsort() function is as follows:
arsort($array);
where $array is the array that needs to be sorted.
The following is an example of sorting an array in descending order:
<?php $numbers = array(4, 2, 8, 6); arsort($numbers); print_r($numbers); ?>
The output results are as follows:
Array ( [2] => 8 [3] => 6 [0] => 4 [1] => 2 )
As can be seen from the output results, the arsort() function sorts the elements in the array The elements are arranged from largest to smallest, while keeping the key-value association unchanged.
6. ksort() function
The ksort() function can sort the PHP array according to the key, in order from small to large. The syntax format of the function is as follows:
ksort($array);
Among them, $array is the array that needs to be sorted.
The following is an example of sorting an array in ascending order:
<?php $numbers = array( "c" => 4, "a" => 2, "b" => 8, "d" => 6 ); ksort($numbers); print_r($numbers); ?>
The output results are as follows:
Array ( [a] => 2 [b] => 8 [c] => 4 [d] => 6 )
It can be seen from the output results that the ksort() function sorts the The elements are arranged in alphabetical order by key.
7. krsort() function
The krsort() function is similar to the ksort() function, except that it arranges the elements of the array in reverse order of keys. The syntax format of the function is as follows :
krsort($array);
Among them, $array is the array that needs to be sorted.
The following is an example of sorting an array in descending order:
<?php $numbers = array( "c" => 4, "a" => 2, "b" => 8, "d" => 6 ); krsort($numbers); print_r($numbers); ?>
The output results are as follows:
Array ( [d] => 6 [c] => 4 [b] => 8 [a] => 2 )
It can be seen from the output results that the krsort() function sorts the elements in the array The elements are sorted in reverse order of keys.
Summary:
In PHP, sorting is a very common and important operation. You can quickly sort arrays using PHP's built-in functions. Of course, we can also use custom functions to sort array elements, which requires a deep understanding of PHP's array operations.
The above is the detailed content of How to sort elements in php array. For more information, please follow other related articles on the PHP Chinese website!
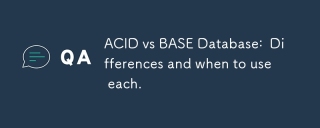
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
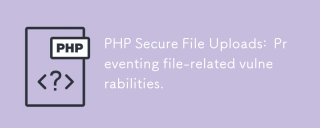
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
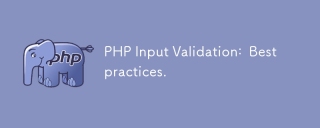
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
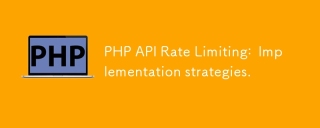
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
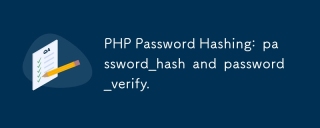
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
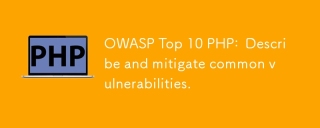
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
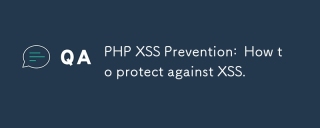
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
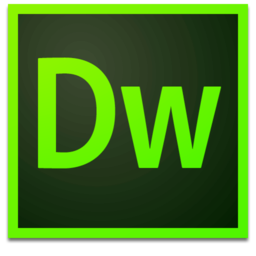
Dreamweaver Mac version
Visual web development tools
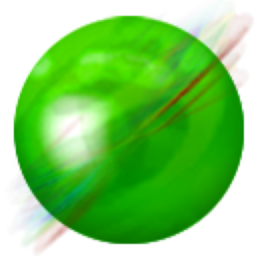
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
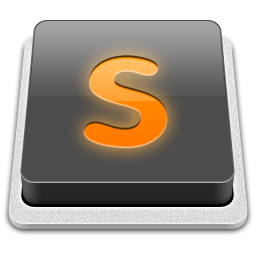
SublimeText3 Mac version
God-level code editing software (SublimeText3)