PHP is a popular server-side scripting language widely used in web development and data processing fields. In PHP, JSON (Javascript Object Notation) is one of the commonly used data formats, and converting JSON into an array is also a very useful operation in PHP development.
JSON is a lightweight data exchange format that can be transmitted in text format and is easy to parse and generate. In PHP, you can convert a JSON string into an associative array or object through the built-in function json_decode().
The following is a simple example showing how to convert a JSON string into an array:
$json_string = '{"name": "John Smith", "age": 30, "city": "New York"}'; $array = json_decode($json_string, true); print_r($array);
In the above example, we first define a variable $json_string that contains a JSON string. We then convert the JSON string into an associative array using the PHP built-in function json_decode() and store the result in the variable $array. Finally, we use the print_r() function to output the contents of the array.
The output results are as follows:
Array ( [name] => John Smith [age] => 30 [city] => New York )
As you can see, the json_decode() function successfully converts the JSON string into an array, and each element in the array contains the corresponding key and value.
In addition to using the json_decode() function to convert a JSON string into an array, you can also use this function to convert the JSON data returned by a JSON file or URL into an array, that is, use the following code:
$jsonURL = "http://example.com/api/getData.php"; $data = json_decode(file_get_contents($jsonURL), true); print_r($data);
In the above code, we use the file_get_contents() function to get a URL containing JSON data and use the json_decode() function to convert the data into an array. Finally, use the print_r() function to output the contents of the array.
In general, converting JSON into an array is very common in PHP development, and you can use the json_decode() function to complete this task. This function is very easy to use, just pass in a JSON string or a file or URL containing JSON data.
The above is the detailed content of How to convert json to array in php. For more information, please follow other related articles on the PHP Chinese website!
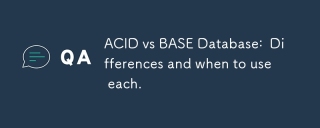
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
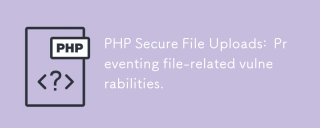
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
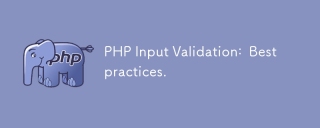
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
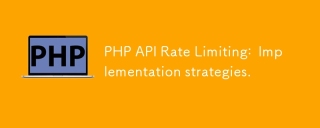
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
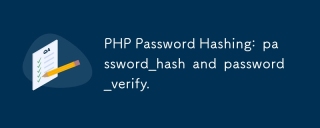
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
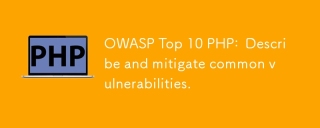
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
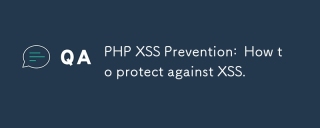
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
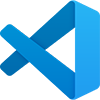
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
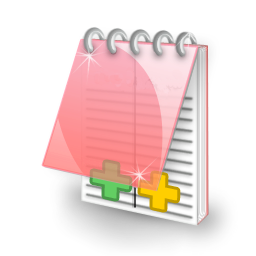
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function