In PHP, manipulating arrays is a basic programming task. Arrays are a very useful data type for storing and manipulating multiple values. In some cases we need to remove elements from an array. This can be done by using PHP built-in functions and methods.
The following will introduce how to use some methods and functions in PHP to remove array elements.
- unset()
The unset() function in PHP is used to delete variables. It can also be used to delete elements from an array. The usage is as follows:
<?php $array = array('a', 'b', 'c', 'd', 'e'); unset($array[1]); //删除'b' ?>
In this example, the element with index 1 (i.e. 'b') in the array is deleted. When using the unset() function, we only need to specify the index of the element to be deleted.
- array_splice()
array_splice() function can delete elements in an array and return a new array. It is used as follows:
<?php $array = array('a', 'b', 'c', 'd', 'e'); array_splice($array, 1, 1); //删除索引为1的元素,即 'b' ?>
In this example, the array_splice() function is used to delete the element with index 1 in the array. The first parameter specifies the array to be operated on, the second parameter specifies the starting index of the element to be deleted, and the third parameter specifies the number of elements to be deleted. In this case, we only need to delete one element.
The array_splice() function can also add new elements to the array. This feature can be used when needed.
- array_filter()
array_filter() function is a useful function that can be used to delete specific elements in an array. Its usage is as follows:
<?php $array = array('a', 'b', 'c', 'd', 'e'); $array = array_filter($array, function($value) { return $value != 'b'; }); ?>
In this example, the 'b' element in the array is removed using the array_filter() function. This function requires two parameters. The first parameter is the array to operate on, and the second parameter is a callback function that determines the elements to retain. In this example, we use an anonymous function that returns all elements except 'b'.
- array_values()
array_values() function can be used to remove elements from an array and reorder the index. Its usage is as follows:
<?php $array = array('a', 'b', 'c', 'd', 'e'); unset($array[1]); //删除 'b' $array = array_values($array); ?>
In this example, the 'b' element with index 1 in the array is deleted using the unset() function, and then the array_values() function is used to re-index the array. Once completed, the array will no longer contain 'b' elements, and the indices of the contained elements will be in order.
Various methods can be used to remove array elements in PHP. Each method has different advantages and disadvantages. When choosing one of these methods, you should consider whether that method is more concise, more readable, and easier to maintain.
The above is the detailed content of How to remove array elements in PHP. For more information, please follow other related articles on the PHP Chinese website!
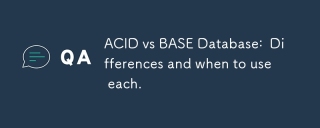
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
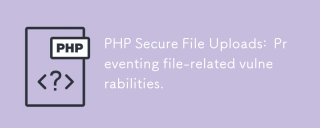
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
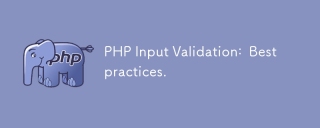
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
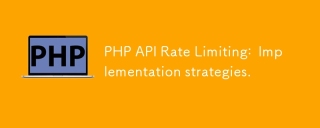
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
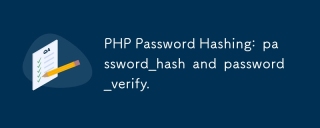
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
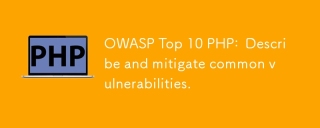
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
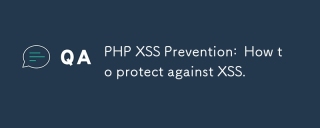
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
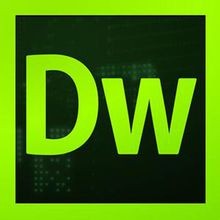
Dreamweaver CS6
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),