With the development of Internet technology, programming languages are also constantly changing and developing. In this context, Erlang and Golang have become popular programming languages. Erlang and Golang themselves have great advantages and applicable scenarios in terms of language features and performance. However, in many cases, due to project requirements or personal preference, programmers or teams need to convert one language to another. Here's how to convert Erlang to Golang.
Why choose Erlang to convert to Golang
Erlang is a programming language oriented to concurrent programming. It is very suitable for high-concurrency, distributed, and scalable system development. In actual project development, Erlang is usually used to build soft real-time systems, such as telephone switches and messaging systems in the telecommunications field. Erlang has the unique Actor model concurrency model, OTP framework, powerful distributed system management and other advantages.
However, Erlang also has some shortcomings, such as complex syntax and lengthy code. In addition, because Erlang is a specialized programming language with good concurrency and scalability, but is not as developed as other languages such as Java and C in other aspects, it also has certain problems in practical applications.
In contrast, Golang has the advantages of better syntax, higher performance, and supports concurrent programming. This is why many companies and teams choose to use Golang for development. Therefore, converting Erlang to Golang is a more reasonable choice.
How to convert Erlang to Golang
For Erlang developers, there are certain differences in the syntax and usage of Golang and Erlang. Below, we will introduce how to convert Erlang to Golang from four aspects:
1. Type conversion
There is no explicit type declaration in Erlang, and variables have dynamic types. In Golang, explicit type declaration is required. Therefore, type conversion is required when converting Erlang to Golang.
For example, defining a string variable in Erlang can be written as:
String = "Hello, World!"
And in Golang, you need to use the variable type declaration:
var String string = "Hello, World!"
For polymorphic functions , called function overloading in Erlang, requires manual type conversion in Golang.
2. Processes and Coroutines
Erlang is a purely message-oriented language based on the Actor model, while Golang uses Goroutine to achieve concurrency. Goroutine is a lightweight thread. Different from Java-like threads, they are executed in the form of coroutine, so they are low-cost and simple to collaborate. When converting Erlang to Golang, you need to convert the process into a coroutine.
For example, in Erlang a process can be created as follows:
Pid = spawn(fun() -> loop() end).
In Golang, you need to use Goroutine to implement:
go loop()
3. Modules and packages
Erlang and Golang are very different in module and package management. In Erlang, modules and code are defined in the same file, and '-module(Name)' is used in the code to represent the module. In Golang, a Java-like package management mechanism is used.
For example, in Erlang, define a module:
-module(example). -export([hello_world/0]). hello_world() -> io:fwrite("Hello, world!").
And in Golang, you need to define a package:
package example import "fmt" func Hello() { fmt.Println("Hello, world!") }
4. Concurrent programming implementation
In Erlang, through the Actor model design, it is extremely simple to implement concurrent programming, and the code is almost unrestricted. In Golang, although Goroutine is very convenient to use, channels need to be used to synchronize Goroutine. When converting Erlang to Golang, appropriate code rewriting is required.
For example, implementing an Actor model in Erlang:
% Worker Actor worker(From) -> receive {multiplication, A, B} -> Result = A * B, From ! {result, Result}, worker(From) end. % Spawn Worker spawn_worker() -> spawn(fun() -> worker(self()) end). % Client Actor client() -> Workers = [spawn_worker() || _ receive {result, Result} -> if length(Workers) > 1 -> workers_sum(tl(Workers), Acc + Result); true -> Acc + Result end end. % Main main() -> client().
And implementing a simple Actor model in Golang:
func worker(ch chan MultiplyRequest) { for { req := <p>Summary</p><p>In practice During project development, due to various reasons such as code performance and system requirements, conversion to different programming languages is required. This article introduces how to convert Erlang to Golang, emphasizing the concurrency advantages of Golang and the need for type conversion, process coroutine, etc. to be rewritten. In short, when selecting and converting a programming language, it is necessary to fully understand the characteristics, advantages and disadvantages of the programming language in order to make reasonable selection and implementation. </p>
The above is the detailed content of How to convert Erlang to Golang. For more information, please follow other related articles on the PHP Chinese website!
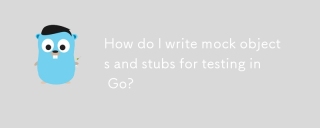
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
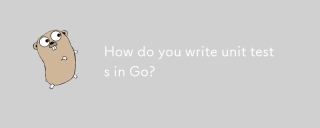
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
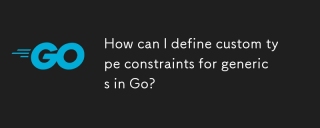
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
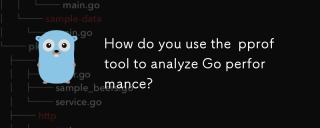
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
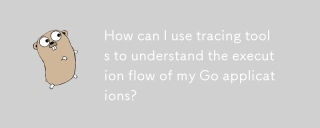
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
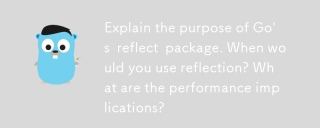
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
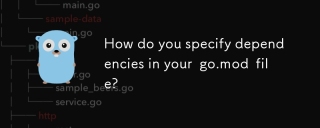
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
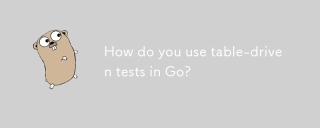
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
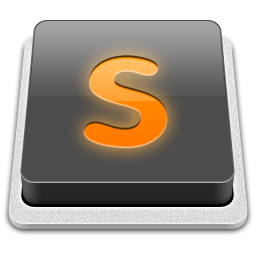
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
