As a widely used programming language, PHP has many built-in functions available. During the coding process, mastering these functions allows developers to write code more quickly and efficiently. This article will explore some of the commonly used functions in PHP.
1. String processing function
- strlen(string $string): Get the string length
This function will return the length of the given string. For example:
$str = "Hello, world!"; echo strlen($str); // 输出:13
- strpos(string $haystack, mixed $needle [, int $offset = 0]): Find the first occurrence of the substring in the string
The The function returns the position of the first occurrence of the specified substring in the given string. If the substring is not found, returns FALSE. For example:
$str = "Hello, world!"; echo strpos($str, "world"); // 输出:7
- str_replace(mixed $search , mixed $replace , mixed $subject [, int &$count ]): Replace the substring in the string
This function will Returns a new string in which all strings matching $search are replaced with the $replace string. For example:
$str = "Hello, world!"; echo str_replace("world", "PHP", $str); // 输出:Hello, PHP!
2. Array processing function
- count(mixed $array_or_countable [, int $mode = COUNT_NORMAL]): Returns the number of elements in the array
This function returns the number of elements in the array. For example:
$arr = [1, 2, 3]; echo count($arr); // 输出:3
- array_push(array &$array , mixed $value1 [, mixed $... ]): Push one or more elements to the end of the array
This function Appends one or more elements to the end of the given array, returning the number of elements in the new array. For example:
$arr = [1, 2, 3]; echo array_push($arr, 4, 5); // 输出:5 print_r($arr); // 输出:Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
- array_merge(array $array1 [, array $... ]): Merge one or more arrays
This function merges two or more arrays into a new array. For example:
$arr1 = [1, 2, 3]; $arr2 = [4, 5]; print_r(array_merge($arr1, $arr2)); // 输出:Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
3. File processing function
- file_get_contents(string $filename [, bool $use_include_path = FALSE [, resource $context [, int $offset = - 1 [, int $maxlen = -1 ]]]]): Read the entire file into a string
This function reads the contents of the specified file into a string. For example:
$file_str = file_get_contents("./test.txt"); echo $file_str;
- file_put_contents(string $filename , mixed $data [, int $flags = 0 [, resource $context ]]): Write a string to the file
This function writes the specified string to the specified file. For example:
$data = "Hello, world!"; file_put_contents("./test.txt", $data);
- scandir(string $directory [, int $sorting_order = SCANDIR_SORT_ASCENDING [, resource $context ]]): Returns the files and directories in the specified directory
This function will Returns all files and directories in the specified directory. For example:
$files = scandir("./"); print_r($files);
The above are only some basic functions built into PHP. In the process of using PHP, other more complex functions and components will also be involved. Understanding and becoming familiar with these functions will help developers develop PHP applications more quickly and efficiently.
The above is the detailed content of [Summary] Some commonly used built-in functions in PHP. For more information, please follow other related articles on the PHP Chinese website!
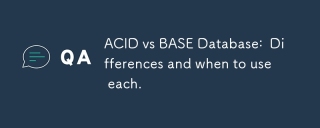
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
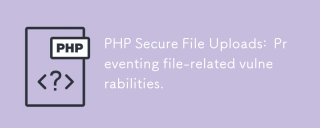
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
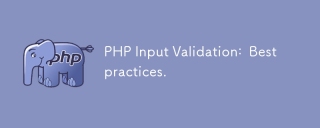
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
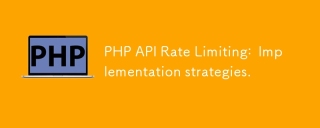
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
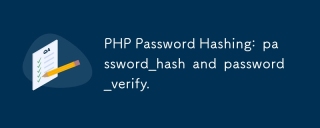
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
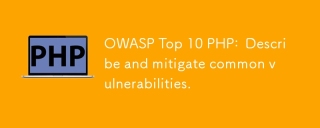
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
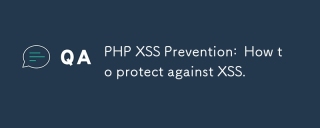
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
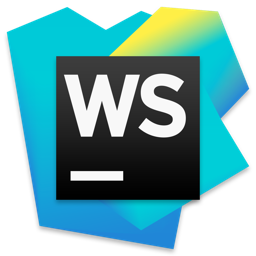
WebStorm Mac version
Useful JavaScript development tools
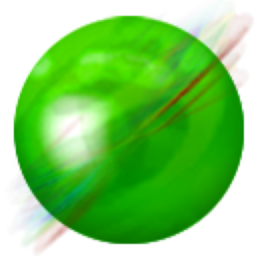
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.