What is parameter hiding in php? How to achieve?
In PHP development, we often need to pass parameters to functions or methods. These parameters can be values of various types, such as integers, strings, arrays, etc. But in some cases, we may encounter a situation where we need to hide some parameters of a function or method. This is called parameter hiding.
What is parameter hiding?
Parameter hiding refers to hiding certain parameters in a function or method so that they do not need to be specified when calling, but can still be used inside the function. This technique is very useful in some situations, such as:
- Hide some fixed parameters to make function calls more concise.
- Hide some private parameters to make the function's logic clearer and avoid being tampered with by external code.
- Hide some parameters that need to be calculated to make the function more efficient.
How to implement parameter hiding?
In PHP, we can use default parameter values to achieve parameter hiding. Default parameter values can be specified when the function or method is defined, so that when the function is called, if no value is passed for the parameter, the default value will be used.
The default parameter value is defined as follows:
function myFunc($arg1, $arg2 = "default_value") { // 代码 }
In the definition, we set the default value of $arg2
to "default_value"
. This means that when a function is called, if no $arg2
argument is passed, "default_value"
will be used.
For example:
myFunc("Hello"); // $arg1 = "Hello", $arg2 = "default_value" myFunc("Hello", "World"); // $arg1 = "Hello", $arg2 = "World"
In this example, we called the myFunc
function twice, and the first time did not pass the $arg2
parameter, so The default value "default_value"
is used. The second time the $arg2
argument was passed, so the passed value "World"
was used.
In addition to default parameter values, we can also use array parameters to implement parameter hiding. In this way, we form all the parameters into an array and use this array as the only parameter of the function. This way, inside the function, the required parameters can be extracted as needed.
Array parameters are defined as follows:
function myFunc($args) { $arg1 = $args["arg1"]; $arg2 = $args["arg2"]; // 代码 }
In the definition, we define the parameters of the function $args
as an array. Inside the function we can extract the required arguments from $args
as needed.
For example:
myFunc(["arg1" => "Hello", "arg2" => "World"]); // $arg1 = "Hello", $arg2 = "World"
In this example, we call the myFunc
function, and the parameters are passed in an array. Inside the function, we extracted the required arguments via $args["arg1"]
and $args["arg2"]
.
Summary
Parameter hiding is a very useful technique that is widely used in PHP development. We can use default parameter values or array parameters to achieve parameter hiding. In this way, we can more flexibly control the transfer and use of function parameters, making the program code more concise and efficient.
The above is the detailed content of What is parameter hiding in php? How to achieve?. For more information, please follow other related articles on the PHP Chinese website!
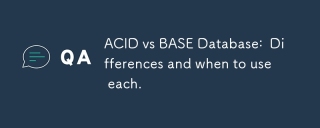
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
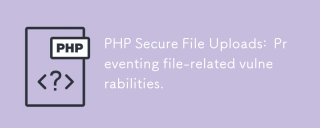
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
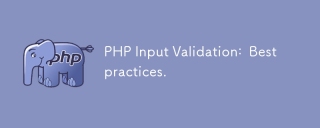
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
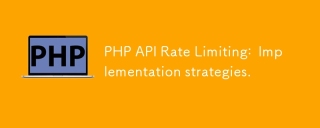
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
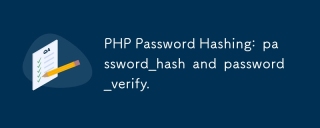
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
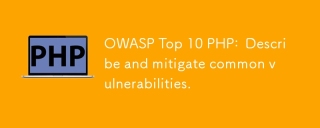
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
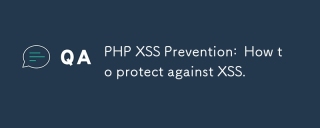
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
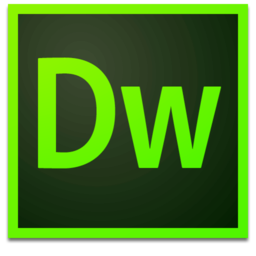
Dreamweaver Mac version
Visual web development tools
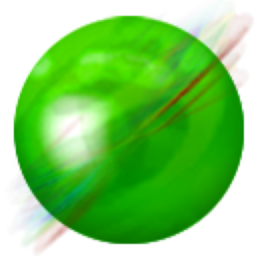
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
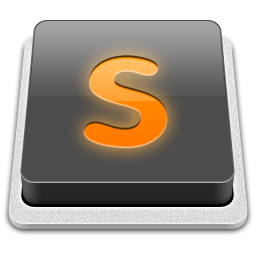
SublimeText3 Mac version
God-level code editing software (SublimeText3)