1. Requirements Analysis
In order to conveniently, accurately and quickly display the student's age, the system usually automatically calculates it based on the student's date of birth. Next, please use PHP variables to save the student's year, month, and day respectively, and obtain the current year, month, and day through the data function in PHP, and finally calculate the student's age.
For example:
The date of birth is: August 2, 2000
If it is May 2020, the age is 20 years old
If it is October 2020, the age is 19 years old
2. Design ideas
How to define Variables store student information. What are these variables?
#How to define variables to save the year, month, and day of a student’s birth?
#How to get the year, month and date of the current time?
#How to calculate a student’s age from birth to the current year?
#How to determine whether a student has a birthday?
3. Knowledge reserve
1. Introduction to PHP Date/Time
Date/Time function allows you to Get the date and time from the server where the PHP script is running. You can use the Date/Time functions to format dates and times in different ways.
2. Definition and usage
date() function formats the local date and time and returns the formatted date string.
3. Syntax
date(format,timestamp);
Returns a string generated by integer timestamp according to the given format string. If no timestamp is given, the local current time is used. In other words, timestamp is optional and the default value is time().
For details, please read this article: https://www.jb51.net/article/148360.htm
4. Several commonly used parameters
4. Code implementation
<?php //定义变量保存学生出生的年、月、日 $stu_by = 2001; $stu_bm = 8; $stu_bd = 07; //获取当前时间的年份、月份和日期 $cur_y = date('Y'); //4位数字完整表示的年份 $cur_m = date('n'); //数字表示的月份,没有前导零,1~12 $cur_d = date('j'); //月份中的第几天,没有前导零,1~31 //计算学生从出生到当前年的周岁 $age = $cur_y - $stu_by; //判断学生是否已过生日 if($cur_m < $stu_bm || $cur_m==$stu_bm && $cur_d<$stu_bd){ $age--; } ?>
5. Effect display
Today is November 2, 2020.
The above is the detailed content of PHP basic case 2: Calculating student age. For more information, please follow other related articles on the PHP Chinese website!
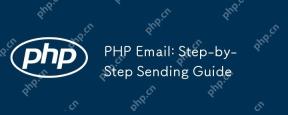
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
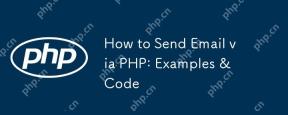
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
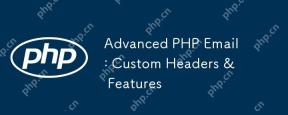
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
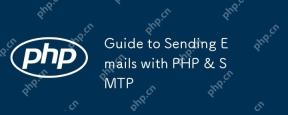
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
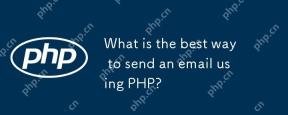
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
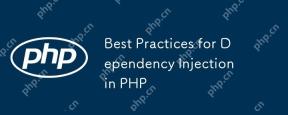
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
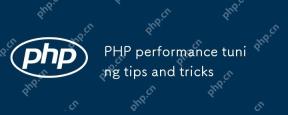
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
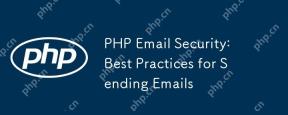
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
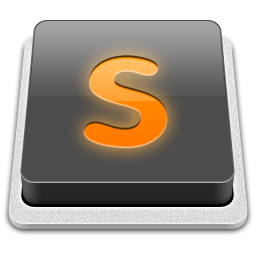
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
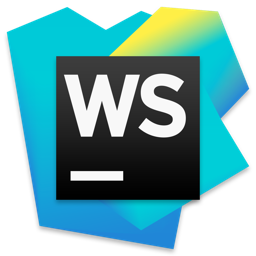
WebStorm Mac version
Useful JavaScript development tools
