php method to delete duplicate values in an array: 1. Use array_unique() to delete duplicate values in an array; 2. Use the array_flip() function to indirectly delete duplicate values in an array. array_flip() reverses the array keys and values. If two values in the array are the same, the last key and value will be retained after the reversal.
php method to delete duplicate values from an array
1. Use array_unique ()Function
array_unique() function is used to remove duplicate values from an array. If two or more array values are the same, only the first value is retained and the other values are removed. [Tutorial Recommendation: PHP Tutorial]
Note: The retained array will retain the key type of the first array item.
Return value: Returns the filtered array.
Code example:
<?php $result1 = array("a" => "green", "red", "b" => "green", "blue", "red"); var_dump($result1); $result2 = array_unique($result1); var_dump($result2); ?>
Output:
array (size=5) 'a' => string 'green' (length=5) 0 => string 'red' (length=3) 'b' => string 'green' (length=5) 1 => string 'blue' (length=4) 2 => string 'red' (length=3) array (size=3) 'a' => string 'green' (length=5) 0 => string 'red' (length=3) 1 => string 'blue' (length=4)
2. Use array_flip() function
array_flip() The function is used to reverse/exchange the key names in the array and the corresponding associated key values.
It has a feature that if two values in the array are the same, then the last key and value will be retained after the inversion. Using this feature, we use it to indirectly achieve deduplication of the array.
<?php header("content-type:text/html;charset=utf-8"); $a = array(1, 5, 2, 5, 1, 3, 2, 4, 5); // 输出原始数组 echo "原始数组 :"; var_dump($a); // 通过使用翻转键和值移除重复值 $a = array_flip($a); // 通过再次翻转键和值来恢复数组元素 $a = array_flip($a); // 重新排序数组键 $a = array_values($a); // 输出更新后的数组 echo "更新数组 :"; var_dump($a); ?>
Output:
原始数组 :array (size=9) 0 => int 1 1 => int 5 2 => int 2 3 => int 5 4 => int 1 5 => int 3 6 => int 2 7 => int 4 8 => int 5 更新数组 :array (size=5) 0 => int 1 1 => int 5 2 => int 2 3 => int 3 4 => int 4
The above is the detailed content of How to remove duplicate values from array in php?. For more information, please follow other related articles on the PHP Chinese website!
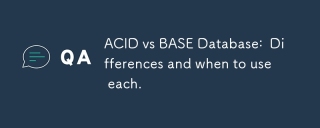
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
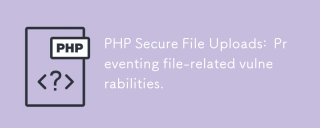
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
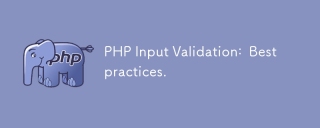
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
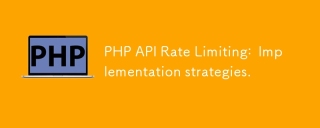
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
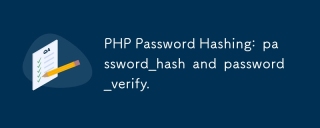
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
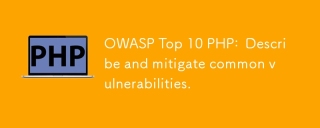
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
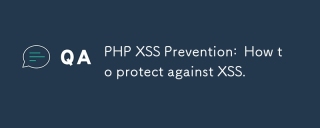
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
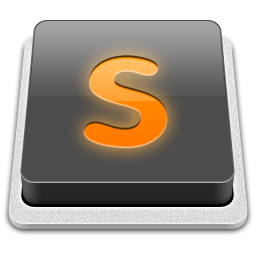
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.